Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial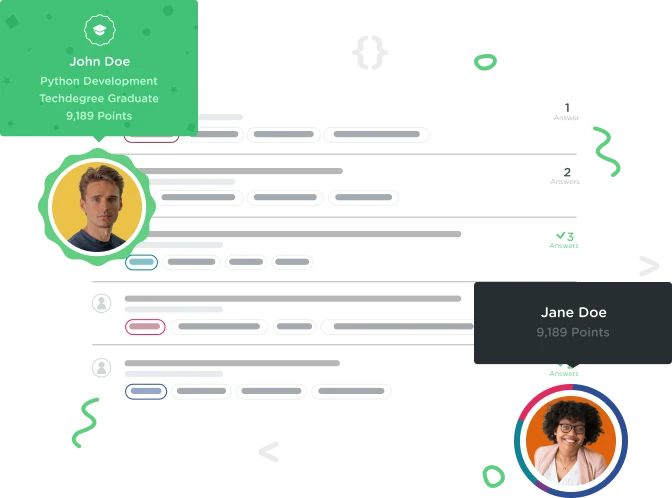
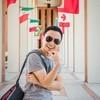
hamsternation
26,616 PointsFiltering High Scores submission failing
I think there's an issue with all these Gradle based code submission tasks. I'm pretty certain my code itself is correct, but every time I try to submit the code to these challenges, it says:
"Oh no! We encountered an error with your last submission."
My code:
public static void printBurgerTimeScoresDeclaratively(List<Score> scores) {
// TODO: Open a stream off of scores and filter "Burger Time" scores that are larger than 20,000
// TODO: Print out those scores using the forEach method off of the Stream
scores.stream()
.filter(score -> score.getGame().equals("Burger Time"))
.filter(score -> score.getAmount() >= 20000)
.forEach(System.out::println);
}
my output locally in my IDE:
2:59:40 PM: Executing ':Main.main()'...
:compileJava
:processResources
:classes
:Main.main()
There are 3549 total scores.
Imperative Burger Time Scores Greater than 20,000
Score{game='Burger Time', measuringUnit='NTSC - Points', amountAsString='2,078,500', player='Matthew Grimm', platform='Nintendo Entertainment System'}
Score{game='Burger Time', measuringUnit='NTSC - Points', amountAsString='225,650', player='Christopher Jedrzejek', platform='Nintendo Entertainment System'}
Score{game='Burger Time', measuringUnit='NTSC - Points', amountAsString='73,400', player='Patrick Scott Patterson', platform='Nintendo Entertainment System'}
Score{game='Burger Time', measuringUnit='NTSC - Points', amountAsString='59,550', player='Eric Cummings', platform='Nintendo Entertainment System'}
Declarative Burger Time Scores Greater than 20,000
Score{game='Burger Time', measuringUnit='NTSC - Points', amountAsString='2,078,500', player='Matthew Grimm', platform='Nintendo Entertainment System'}
Score{game='Burger Time', measuringUnit='NTSC - Points', amountAsString='225,650', player='Christopher Jedrzejek', platform='Nintendo Entertainment System'}
Score{game='Burger Time', measuringUnit='NTSC - Points', amountAsString='73,400', player='Patrick Scott Patterson', platform='Nintendo Entertainment System'}
Score{game='Burger Time', measuringUnit='NTSC - Points', amountAsString='59,550', player='Eric Cummings', platform='Nintendo Entertainment System'}
BUILD SUCCESSFUL
Total time: 1.338 secs
2:59:41 PM: Execution finished ':Main.main()'.
This happens to the for each challenge and the using method references challenges as well!
@Craig Dennis

Andrew Stevens
11,083 PointsI am having the same issue hamsternation did you ever pass this? can't see anything obviously wrong with your code or mine.
public class Main {
public static void main(String[] args) {
ScoreService service = new ScoreService();
List<Score> scores = service.getAllScores();
System.out.printf("There are %d total scores. %n %n", scores.size());
System.out.println("Imperative Burger Time Scores Greater than 20,000");
printBurgerTimeScoresImperatively(scores);
System.out.println("Declarative Burger Time Scores Greater than 20,000");
printBurgerTimeScoresDeclaratively(scores);
}
public static void printBurgerTimeScoresImperatively(List<Score> scores) {
for (Score score : scores) {
if (score.getGame().equals("Burger Time") && score.getAmount() >= 20000) {
System.out.println(score);
}
}
}
public static void printBurgerTimeScoresDeclaratively(List<Score> scores) {
// TODO: Open a stream off of scores and filter "Burger Time" scores that are larger than 20,000
scores.stream()
.filter(score -> score.getGame().equals("Burger Time"))
.filter(score -> score.getAmount() >= 20000)
// TODO: Print out those scores using the forEach method off of the Stream
.forEach(System.out::println);
}
}
hamsternation
26,616 Pointshamsternation
26,616 PointsCraig Dennis