Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial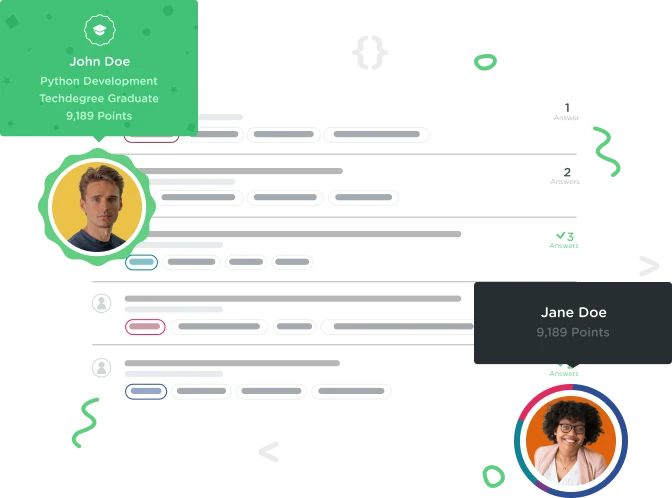

Andrew Smith
2,516 PointsFlies spawning out of control
Everytime I click the play button in Unity, the flies start spawning out of control and I have to stop it before it crashes. I have looked back through the videos to make sure I didn't miss a step, and I have tried to figure out what the problem is. It seems to me like the condition keeps going because of there being no MAX amount but it works fine in the tutorials?? Here is my code:
using UnityEngine; using System.Collections;
public class FlySpawner : MonoBehaviour {
[SerializeField]
private GameObject flyPrefab;
[SerializeField]
private int totalFlyMinimum = 12;
private float spawnArea = 25f;
// public accessor can allow other scripts/classes can change it's value
// static variable creates a reference variable that is the same across all
// instances of the class
public static int totalFlies;
// Use this for initialization
void Start () {
totalFlies = 0;
}
// Update is called once per frame
void Update () {
// while the total number of flies is less than the minimum...
while (totalFlies < totalFlyMinimum) {
// ...then increment the total number of flies
totalFlies++;
// ...create a random position for a fly...
float positionX = Random.Range(-spawnArea, spawnArea);
float positionZ = Random.Range(-spawnArea, spawnArea);
Vector3 flyPosition = new Vector3(positionX, 2f, positionZ);
// ...and create a new fly.
Instantiate(flyPrefab, flyPosition, Quaternion.identity);
}
}
}

Andrew Smith
2,516 PointsNope, the value of totalFlyMinimum is 12 in the inspector.
3 Answers

Joel Alcantara Nuñez
2,289 PointsHi Andrew,
Try this, delete the script and create it again maybe is a bug

Tung Nguyen
1,519 PointsThank you, this works for me.
I copied the source code > delete "FlySpawner.cs" > remove "Fly Spawner Script component" in the Inspector panel and create that component again with the copied source code. It works correctly, and that seems a bug of Unity.
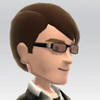
James Marchment
2,048 PointsI'm thinking that either totalFlies++ is being read as part of the comment line above somehow (and therefore not incrementing) or the Instantiate is winding up outside the 'while' loop somehow (and running on every frame as part of Update). From the code posted, it doesn't look like either of those things is happening, but the problem sounds like something along those lines is somehow taking place.
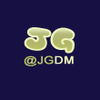
Jonathan Grieve
Treehouse Moderator 91,253 PointsI had this problem and was able to fix it by removing the script component from the game manager and making sure it was added to the Fly prefab. :)
Helgi Helgason
7,599 PointsHelgi Helgason
7,599 Pointsdid you change the value of totalFlyMinimum in the inspector?