Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial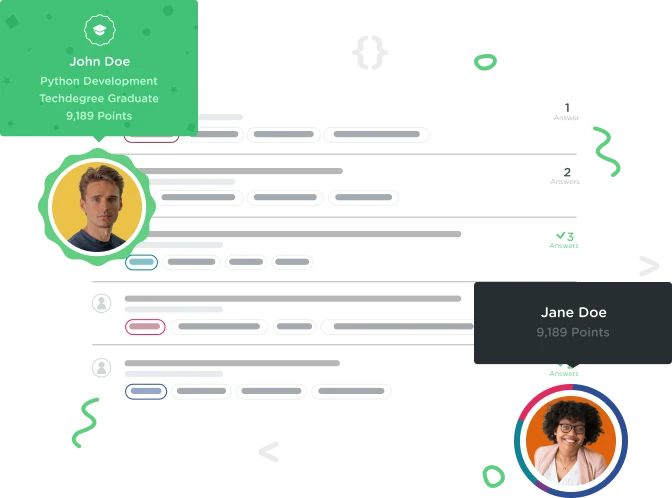
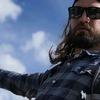
Bryce Vernon
3,936 PointsGenerics Code Challenge Help!
I've been stuck on this code challenge. I need to iterate over the array but check each string's prefix character. Not sure how to do this with an array.
In the editor I've defined a protocol, PrefixContaining, that specifies that conforming types can determine whether a value contains a prefix. I've also gone ahead and added conformance to the String type. Your task is to extend the Array type and add a function that lets you filter by prefix but only in cases where the array contains types that conform to PrefixContaining. The function signature should be as follows: func filter(byPrefix prefix: String) -> [Element] For example, your code should return the following results
let test = ["aa", "ba", "ca", "ab"]
let result = test.filter(byPrefix: "a") // result = ["aa", "ab"]
Any help would be awesome.
protocol PrefixContaining {
func hasPrefix(_ prefix: String) -> Bool
}
extension String: PrefixContaining {}
// Enter code below
5 Answers
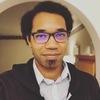
Harold Davis
Courses Plus Student 14,604 PointsI don't know if you are still stuck on this one it was a challenge I figured it out. If you want to look and understand...
protocol PrefixContaining {
func hasPrefix(_ prefix: String) -> Bool
}
extension String: PrefixContaining {}
extension Array where Element: PrefixContaining {
func filter(byPrefix prefix: String) -> [Element]{
var output = [Element]()
for element in self {
if (element.hasPrefix(prefix)) == true {
output.append(element)
}
}
return output
}
}
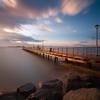
Qasa Lee
18,916 Pointsprotocol PrefixContaining {
func hasPrefix(_ prefix: String) -> Bool
}
extension String: PrefixContaining {}
// Enter code below
extension Array where Element: PrefixContaining {
func filter(byPrefix prefix: String) -> [Element]{
var filteredArray = Array<Element>()
for element in self {
if(element.hasPrefix(prefix)) {
filteredArray.append(element)
}
}
return filteredArray
}
}
//let test = ["aa", "ba", "ca", "ab"]
//let result = test.filter(byPrefix: "a") // result = ["aa", "ab"]
This may help, good luck!

Dhanish Gajjar
20,185 PointsBryce Vernon Check out my post from a few days ago https://teamtreehouse.com/community/generics-in-swift-3-final-challenge

Emin Grbo
13,092 PointsThis helped a lot guys ! Turns out i was really close, but only forgot the proper Array declaration with "<Element>" :)
Cheers !

Hong Duong Dang
17,777 PointsThis is a more elegant way to do
protocol PrefixContaining {
func hasPrefix(_ prefix: String) -> Bool
}
extension String: PrefixContaining {}
// Enter code below
extension Array where Element: PrefixContaining {
func filter(byPrefix prefix: String) -> [Element] {
return self.filter{$0.hasPrefix(prefix)}
}
}
Tony Burrus
10,280 PointsTony Burrus
10,280 PointsI had the same solution except for a minor change I've called out below.
"if" statements automatically check if the condition is true, so in the following code
you can simply remove the check and be left with this