Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial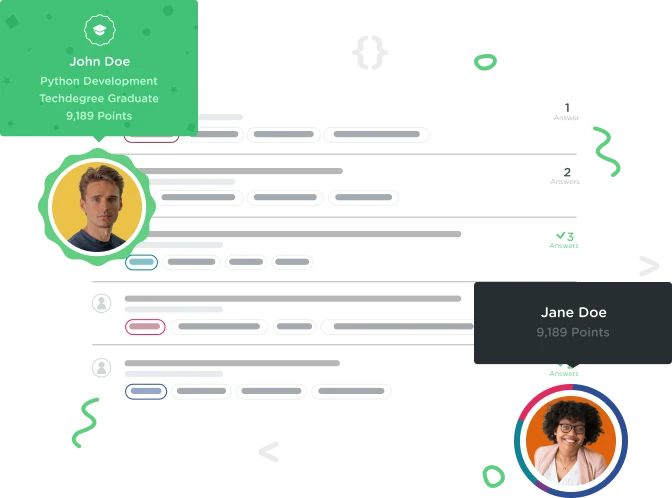

Jason Bourne
2,301 PointsHaving a problem implementing additional functionality
Hey!
So I've developed the number game a little further. When the user makes a guess and it's not the right answer, the function allows the user to try again. Once the user guesses the right number, they are asked if they want to play the game again. The problem I'm having is trying to make the game generate a new random number when the user wants to play again. In its current state, the game always uses the same random number. I've tried so many different ideas but nothing works.... Any ideas? Code is below.
I also removed the while loop because I want the program to end when the user decides to not play again after making one successful guess.
import random, time
print("Guess a number between 1 and 10:")
secret_numb = random.randint(1, 10)
def game():
guess = int(input("> "))
if guess == secret_numb:
print("You win! The secret number was {}.".format(secret_numb))
again = input(
"""=================================
Do you want to play again? y/n:
> """)
if again.lower() == "y":
print("Guess a number between 1 and 10:")
game()
else:
print("Thanks for playing. Bye!")
time.sleep(2)
elif guess > secret_numb:
print("Lower!")
game()
elif guess < secret_numb:
print("Higher!")
game()
game()
3 Answers
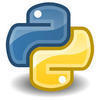
Gerald Wells
12,763 PointsThe issue is global vs local variables, the program runs exactly how you told it to. If you initialize a global variable outside of the scope of the function you won't be able to iterate over it. You need to move the randomly generated value into your fxn.
def game():
number = random.randint(1,10)
while True:
guess = int(input("> "))
....rest of your code
Now when a condition isn't met the while loop will continue run your test sequences. In the same context when a condition is met and the individual wants to "play again" a new randomly generated number will be created when you call that fxn again.
import random
import sys
def game():
randomNumber = random.randint(1,10)
print("Guess a number in between 1 and 10")
while True:
guess = int(input("> "))
if guess != randomNumber:
if guess < randomNumber:
print("Higher")
else:
print("Lower")
else:
print("{} is the correct number".format(guess))
again = input("Play again[Yn]: ").lower()
if again == 'y':
game()
else:
print("Thanks for playing!")
sys.exit()
game()
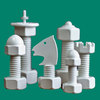
Steven Parker
231,269 Points
You just need to pick a new number before restarting the game.
if again.lower() == "y":
print("Guess a number between 1 and 10:")
secret_numb = random.randint(1, 10) # pick a fresh number
game()

Jason Bourne
2,301 PointsI tried that before. When I run the script, it prompts me for a number as usual, but once I type a number and hit Enter, I get this error:
UnboundLocalError: local variable 'secret_numb' referenced before assignment
Logically, it doesn't make sense to me. I even tried adding a del secret_numb
one line above, but that did nothing.
:(
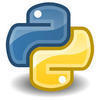
Gerald Wells
12,763 PointsThe variable randomly generated in the scope of the function but outside the the control loop , I'm confused as to what it is you are asking then. You stated " The problem I'm having is trying to make the game generate a new random number when the user wants to play again. " When the code I wrote runs, it generates a random number once until that value is met, when that value is met you have the option to get a different random number if you respond 'y'. Try running some debugging print statements on the code I posted to help you better understand. if you are looking to have a script run and for every bad guess you want to the number to be randomly generated again then this would work:
import random
import sys
def game():
randomNumber = random.randint(1,10)
print("Guess a number in between 1 and 10")
while True:
guess = int(input("> "))
if guess != randomNumber:
print("Incorrect, the number was {}".format(randomNumber))
game()
else:
print("Hurray! {} was indeed the correct value!".format(guess))
Now every time the a guess occurs if the value is incorrect randomNumber is reinitialized.
Overall I think you need to read some information outside of what Teamtreehouse provides. Kenneth Love does a great job in his course but it isn't really a one stop shop on the subject. I recommend you read "Fundamentals of Python: My First Programs" and "Fundamentals of Python: Data Structures" By Kenneth A Lambert. A good article in regards to this current topic is http://python-textbok.readthedocs.io/en/1.0/Variables_and_Scope.html

Jason Bourne
2,301 PointsAh now I understand, thanks!
I fixed my problem :)
Jason Bourne
2,301 PointsJason Bourne
2,301 PointsThanks a lot for the reply!
Unfortunately you've removed functionality with your code. When the program spits out
Higher
orLower
, I'd like it to ask the user to try again, like in my script.In order to do this, I make my script re-run
game()
after every bad guess, but if you define the variableSecret_numb
inside the loop, then the random number changes at every guess...So basically I don't know where to declare the variable to make it do what I want!