Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial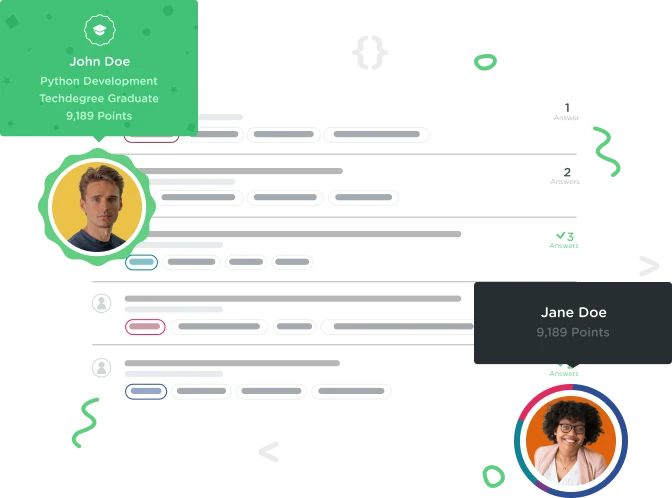

Michael Leonard
1,279 PointsHaving issues with python challenge
This is the script I wrote for the Python Basics Challenge test.
my_list = [1, 2, 3]
def add_list(my_list):
total = 0
for item in my_list:
total = total + item
continue
return(total)
totalnum = add_list(my_list)
print(totalnum)
total_sum = add_list(my_list)
def summarize(total_sum, my_list):
print("The sum of {} is {}.".format(str(my_list), total_sum))
summarize(total_sum, my_list)
It runs perfectly in the terminal but when I check code in the workspace it gives me this error. "TypeError: summarize() missing 1 required positional argument: 'my_list'"
Any help is appreciated. Thanks!
Update:
Fixed it with this code:
def summarize(my_list)
final_string = "The sum of {} is {}.".format(str(my_list), total_sum)
return(final_string)
Not sure why i don't have to include total_sum in the function parameters.
Can somebody explain this?
1 Answer
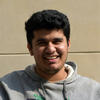
Ratik Sharma
32,885 PointsYou don't have to include total_sum in the function parameters because in your script, you've defined total_sum outside any block of code (for instance, a function) and Python treats it as a variable with global scope (ie, it can be accessed anywhere in the script). And that's why summarize() doesn't require it as a parameter.
Also, the challenge just requires you to just create two functions. You don't need to create a list and pass it into the functions.
Here is how I would write this script:
def add_list(my_list):
total = 0
for item in my_list:
total += item # this is equivalent to total = total + item
# the `continue` isn't really required
return(total)
def summarize(my_list):
print("The sum of {} is {}.".format(str(my_list), add_list(my_list)))

Michael Leonard
1,279 PointsThanks Ratik! That clears it up for me.
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsThe challenge is supposed to require the function
summarize()
call the functionadd_list()
to get the correct results. It may be a bug in how the grader is evaluating your code that is letting it pass when you create a global with the results ofadd_list
. Tagging Kenneth Love