Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial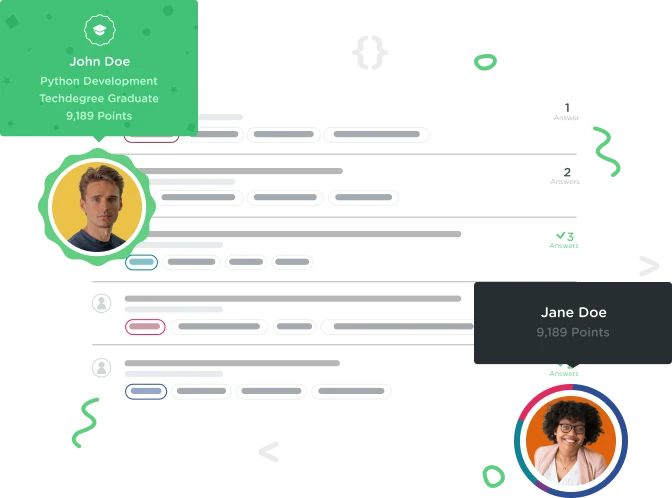
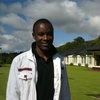
Admire Dhodho
5,411 PointsHelp me on this one i am mixing things up
Let's allow our BlogPost to be sorted. In this first step, just make the class implement Comparable interface, and produce a compareTo method that accepts an object as a parameter. Have it always return 1 for this first step, we'll do more in the next step.?
package com.example;
import java.util.Date;
public class BlogPost {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
@Override
public String[] getWords() {
return mBody.split("\\s+");
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
@override
public int campareTo(Object obj){
BlogPost other = (BlogPost) obj;
return 1;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
5 Answers

Alex Stephens
13,342 Pointshello i have just done the first part of the code challenge but in my code am not using @Override in my code but i still pass the code challenge why is it letting me pass without @Override ? here is my code
package com.example;
import java.util.Date;
public class BlogPost implements Comparable{
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost (String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public String[] getWords() {
return mBody.split("\\s+");
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
public int compareTo(Object obj) {
BlogPost other = (BlogPost) obj;
return 1;
}
}
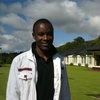
Admire Dhodho
5,411 Pointsits because your code is correct but later in the next stages it might tell you that the test 1 is no longer passing
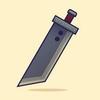
Allan Clark
10,810 PointsCheck out this post i made on another forum question. Let me know if there is anything else I can clear up.
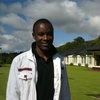
Admire Dhodho
5,411 Points/com/example/BlogPost.java:24: error: method does not override or implement a method from a supertype @Override

Craig Dennis
Treehouse TeacherTry changing @override
to @Override
. Case matters.
Also, @Override
doesn't belong on getWords
, just on compareTo
.
Hope it helps!
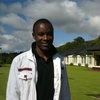
Admire Dhodho
5,411 Points/com/example/BlogPost.java:24: error: method does not override or implement a method from a supertype @Override its giving me this error Found out that i heard forgoten to implement the comparable from the class BlogPost . Thanx a lot craig

Schwartz Prince
Courses Plus Student 2,132 PointsHi, Is that necessary to write @Override in the code? What will happen if we not writing the same in the code? Please explain

Matt Bloomer
10,608 PointsI copied and pasted the answer above, and the error message said that the class needs to implement the Comparable method; what exactly is it asking me to do?

Craig Dennis
Treehouse Teacher// Assuming the 'implements Comparable' portion
public class BlogPost implements Comparable {
//...
}
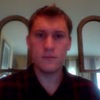
Casey Huckel
Courses Plus Student 4,257 PointsI get 5 errors from this:
package com.example;
import java.util.Date;
public class BlogPost implements Comparable { private String mAuthor; private String mTitle; private String mBody; private String mCategory; private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) { mAuthor = author; mTitle = title; mBody = body; mCategory = category; mCreationDate = creationDate; }
public String[] getWords() { return mBody.split("\s+"); }
public String getAuthor() { return mAuthor; }
public String getTitle() { return mTitle; }
public String getBody() { return mBody; }
public String getCategory() { return mCategory; }
public Date getCreationDate() { return mCreationDate; }
@Override public compareTo(Object object) { BlogPost other = (BlogPost) obj; return 1; } }
Dumitru Petrov
908 PointsDumitru Petrov
908 PointsYou write "campareTo" instead of "compareTo"