Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial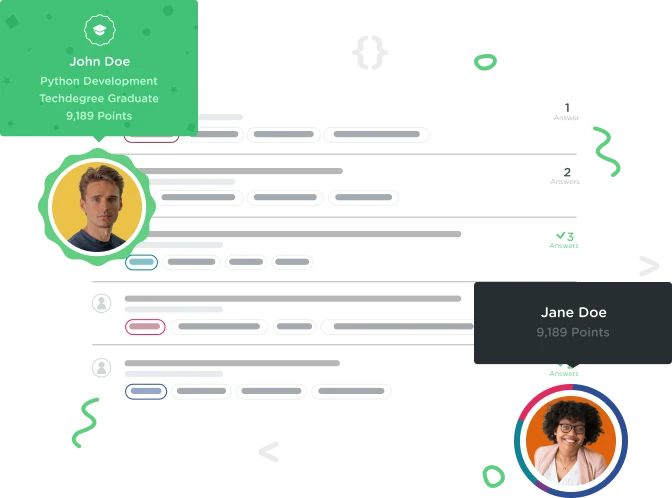
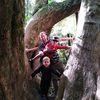
Brett McGregor
Courses Plus Student 1,903 PointsHere is my solution. It works, but can someone help me get rid of spaces in the print output for half_birthdays. Thanks!
# Columns: name, day/month, celebrates, age
BIRTHDAYS = (
("James", "9/8", True, 9),
("Shawna", "12/6", True, 22),
("Amaya", "28/6", False, 8),
("Kamal", "29/4", True, 66),
("Billy", "16/7", True, 1),
("Sam", "21/4", True, 18),
("Jordan", "19/9", True, 55),
)
# problem: celeberations
# loop through all of the people in Birthdays
# if they celebrate their birthday, print out
# "Happy Birthday" and their name
print("Celebrations:")
for person in BIRTHDAYS:
if person[2] == True:
print("Happy Birthday,",person[0])
print("-"*20,"\n")
# problem 2
# half birthdays
# loop through and calc half birthday (six months later)
# print out their name and half birthday
print("Half birthdays:")
for person in BIRTHDAYS:
half_birthday = int(person[1].split("/")[1])+6
if half_birthday > 12:
half_birthday -= 12
print(person[0],person[1].split("/")[0],"/",half_birthday)
print("-"*20,"\n")
#3: only school year birthdays
# loop through and if birthday is between Sept and June
# print their name
print("School birthdays:")
for person in BIRTHDAYS:
birth_month = int(person[1].split("/")[1])
if birth_month not in range(7,9):
print(person[0])
print("-"*20,"\n")
# 4: wishing stars
# loop through if person celebrates birthday
# and age <=10 print out name and as many stars as their age
print("Stars:")
for person in BIRTHDAYS:
if person[2] == True and person[3] <= 10:
print(person[0], "*" * int(person[3]))
print("-"*20,"\n")
I get spaces either side of the slash e.g.
Half birthdays: James 9 / 2 Shawna 12 / 12 Amaya 28 / 12 Kamal 29 / 10 Billy 16 / 1 Sam 21 / 10 Jordan 19 / 3
My guess is that the unwanted spaces are something to do with data types?
1 Answer

Jon Mirow
9,864 PointsHi there,
Nice work! The spaces are coming from the commas in your print statements. The TL;DR: of this is that you just have to change your commas (,) in the print statement for plus signs (+):
print(person[0], person[1].split("/")[0]+"/" + str(half_birthday))
Note that you have to convert half_birthday to a string before joining them Explanation follows.
It's all to do with string concatenation and how functions work. print() is a function, so if you feed strings in seperatedd by a comma, they go in as separate arguments, if you use the + sign, python will concatenate (join) the strings before you pass them to print as a single argument. Because + is a method of strings, you have to convert any numbers to strings before you can use this (otherwise python will try and perform addition on the string and number).
Python's print function has a couple of formatting presets - for example a newline is by default inserted at the end of each function call, and similarly white space is inserted between arguments.
So, say I want to print the classic hello world text:
>>>print("hello", "world")
>>># print receives 2 arguments and separates with a space:
hello world
>>>print("hello" + "world")
>>># the strings are joined together first and then passed to print as a single argument:
helloworld
We can change the default behaviour of print using the 'sep' and 'end' keywords. For example, the following will take two surnames, hyphenate them and add a full stop at the end before adding a newline:
>>>surname_one = "Newton"
>>>surname_two = "John"
>>>print(surname_one, surname_two, sep="-", end=".\n")
Newton-John.
>>>
Don't worry you don't have to memorise all that the print function can do, you can just look it up when you need it: https://docs.python.org/3/library/functions.html#print
Hope it helps!
Brett McGregor
Courses Plus Student 1,903 PointsBrett McGregor
Courses Plus Student 1,903 PointsJon, Thanks for taking the time to provide a detailed response.
I have fixed that line of code and now get the output I was after, without the spaces.
You da man!