Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial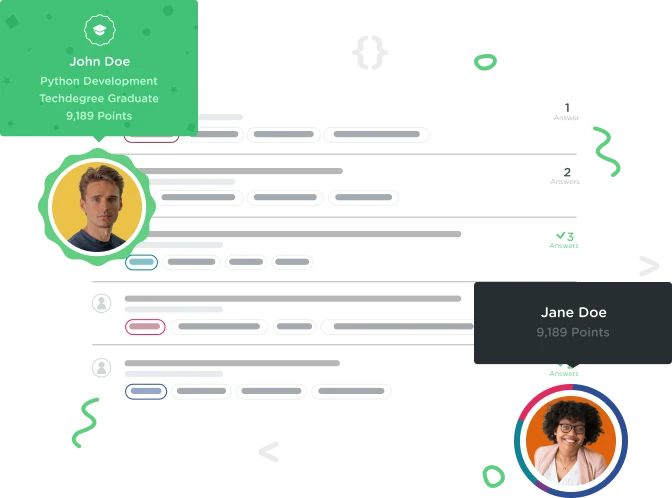

Greg Kaleka
39,021 PointsHere's a slightly more complex but more dynamic solution.
At the end of this video, Ben Deitch has a suggestion that you might want to return all rooms with the max capacity in case there is more than one. Rather than hard-coding in the number 40, you can use a subquery:
SELECT ID, CAPACITY FROM ROOMS
WHERE CAPACITY = (
SELECT MAX(CAPACITY) FROM ROOMS
);
Just thought I'd share
Cheers
-Greg
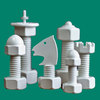
Steven Parker
241,783 PointsThat's a misuse of an aggregate function. You cannot use aggregate functions in a WHERE clause or in a JOIN condition.

Michael Hill
3,960 PointsThis is a great answer and I can't think of a better way to optimize this, but I've been having fun finding alternate solutions. Here is my take using a CTE. I used MIN for this example since there are two of them in the playground tied to this challenge.
WITH room_limit AS (
SELECT MIN(CAPACITY) AS CAPACITY FROM ROOMS
)
Select ROOMS.ID, ROOMS.CAPACITY FROM ROOMS
JOIN room_limit ON ROOMS.CAPACITY = room_limit.CAPACITY
WHERE ROOMS.CAPACITY = room_limit.CAPACITY;

Sumiya Malik
3,976 PointsSELECT ID, MAX(CAPACITY) AS LARGEST_ROOM FROM ROOMS
7 Answers

nfs
35,526 Points+1 Greg Kaleka.
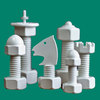
Steven Parker
241,783 PointsExcellent idea.
You could also do this:
SELECT ID, MAX(CAPACITY) as CAPACITY FROM ROOMS
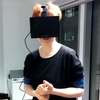
Mia Filisch
16,117 PointsBut this will aggregate the results so you only see one row, even when there are multiple rooms with the same max capacity... whereas the task, as you point out below, was "to return all rooms with the max capacity in case there is more than one".
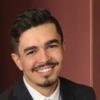
kaganulucay
Full Stack JavaScript Techdegree Student 16,491 PointsHere is my solution:
SELECT capacity FROM rooms ORDER BY capacity DESC LIMIT 1

kevin hudson
Courses Plus Student 11,987 PointsThat's what I did but I think we are supposed to progress in complexity by using the next way of doing things. The DRY way I guess.
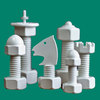
Steven Parker
241,783 PointsBut this clearly limits the output to one row. The task was "to return all rooms with the max capacity in case there is more than one".

Tatia Burdett
1,470 PointsThat's what I did. :D

Ryan Leadenham
139 PointsNot only is this a better solution, it is the only correct solution. OK technically not the only one.... but the query shown in the video doesn't return the ID for the room with the largest capacity... it returns a random row then an unrelated field with the largest capacity.
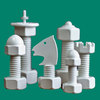
Steven Parker
241,783 PointsNo, the video query reliably returns only the row with the maximum capacity. Are you trying it outside of the "SQL Playground", or with some other database than SQLite?

Guilherme Mergulhao
4,002 PointsUsing
SELECT * FROM ROOMS WHERE CAPACITY = (SELECT CAPACITY FROM ROOMS ORDER BY CAPACITY DESC LIMIT 1);
will work as well.

Ryan Leadenham
139 PointsI use MySQL and MariaDB and I'm sure a significant portion of the teamtreehouse audience will as well. Consider this example (Run in the playground).
SELECT *, MAX(capacity), MIN(capacity) FROM rooms
I guess my thought is that, with this behavior, SQLite is in the minority. But I can' t find any references to back this up, so I could be wrong. Either way, with this being framed as an "SQL" class, the particular query in the video should not be recommended for this purpose.

Greg Kaleka
39,021 PointsHey Ryan,
Unfortunately there is no universal SQL syntax that would cover all common DB implementations. You'll find some suggestions for resources on DBs other than SQLite in the teachers notes. If you come across something like this, you can always send an email to support@teamtreehouse.com to suggest adding some additional teachers notes about MySQL implementations.
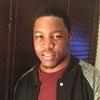
Marcus Grant
Courses Plus Student 2,546 PointsI am using T-SQL on Microsoft SQL Server Management Studio to run my queries. Another dynamic solution I came up with is:
SELECT TOP 1 * FROM ROOMS ORDER BY CAPACITY DESC;
Matthew Stevenson
4,877 PointsMatthew Stevenson
4,877 PointsJust out of curiosity, why doesn't the following query work?