Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial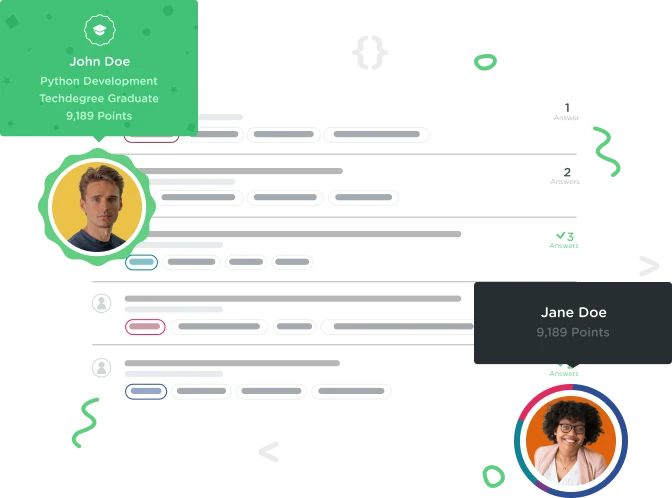
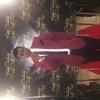
Billy Potts
Courses Plus Student 2,344 Pointshow do i make this struct conform to the protocol im getting an error
In the editor the protocol you created, UserType, is provided along with an empty struct. For this task, Person needs to conform to UserType. After you've written code to achieve this task, create an instance of Person and assign it to a constant named somePerson.
protocol UserType {
var name: String { get }
var age: Int { get set }
}
struct Person: UserType {
var name: String
var age: String
init(name: String, age: Int) {
self.name = name
self.age = age
}
}
let somePerson = Person(name: "Billy", age: 22)
4 Answers

Jonathan Fernandez
4,047 PointsWhy in the above examples, we dont use get constructs or set constructs for age and a get construct for name? Is it because in the protocol when the property is said to "{ get set }" that essentially means it has to be able to be read and has to be able to be changed, which can be done through a declaration in the syntax of a stored property?

Helgi Helgason
7,599 Pointsthe age variable is of type String in your struct but Int in the protocol
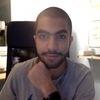
Mohammed Alkandari
3,054 PointsThe (age) in protocol is Int but it's a String in the struct, also you don't need an init method in your struct. Xcode provides you automatically with it without the need to code it!
protocol UserType {
var name: String { get }
var age: Int { get set }
}
struct Person: UserType {
var name: String
var age: Int
}
let somePerson = Person(name: "Billy", age: 22)
hope it helps :)

i6ze5srftzgi8o5iu46z5etr
6,931 Pointsprotocol User{
var name: String { get }
var age: Int { get set }
}
struct Person: User{
var name: String
var age: Int
}
let somePerson = Person(name: "xxxx", age: 22)