Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial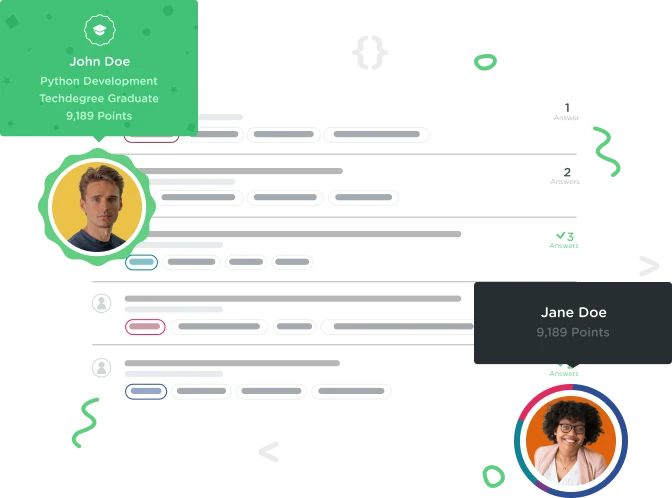

Vlatko .
2,526 PointsHow is the sizeof(float) 4 bytes?
I see why the array length is 3. Just by looking at it, it has 3 values, so the length is 3, correct?
What I'm stumped on is why and how the float size is 4 bytes. Also, how does the sizeof(numbers) equal to 12 bytes?
I feel so lost with this concept.
4 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Vlatko,
The size of a float or other data types for that matter is dependent upon the system. It has to do with the hardware architecture and the compiler.
This float, 10498.429
, would also be 4 bytes in memory. If a given computer system had a float size of 4 bytes then all floats are 4 bytes. You should not be thinking of these floating point numbers as characters in an array and counting how many characters there are.
So when you do float numbers[] = {11.11, 22.22, 33.33};
The computer will set aside 12 bytes of memory because it knows each float is 4 bytes and it has to make room for 3 of them. The array could have been float numbers[] = {0.5, 9.3, 32897.4, 98.568};
and the computer will still reserve 12 bytes of memory.
So when you do sizeof(numbers)
that evaluates to 12 because you're asking for the total size in memory that is occupied by that array.
I think one of the main points that you should get from this video is that you don't really need to know the size of a float. You want to do your calculations in a way that is independent of the system it's running on.
For instance, the teacher calculated the array length with sizeof (numbers) / sizeof (float)
and that comes out to 3 (12 / 4 = 3)
Well, you could get that answer by doing this sizeof(numbers) / 4
The problem with this is that we have assumed a float size of 4. If we now run this program on a system where the float size is something else then we're going to get the wrong length.
I hope this clears it up for you.

Stone Preston
42,016 Pointsyou have come to an incorrect conclusion. in objective C, datatypes use different amounts of memory. a float takes up 4 bytes of space regardless of how many numbers are in it. 0.1 takes up 4 bytes as does 0.222222.
here are some common sizes of primitive types:
Primitive sizes:
The size of a char is: 1.
The size of short is: 2.
The size of int is: 4.
The size of long is: 4.
The size of long long is: 8.
The size of a unsigned char is: 1.
The size of unsigned short is: 2.
The size of unsigned int is: 4.
The size of unsigned long is: 4.
The size of unsigned long long is: 8.
The size of a float is: 4.
The size of a double is 8.
now since a float can only fit into 4 bytes of memory, there is a limit to the size of the numbers you can use. this is true of all data types as well. the limit is usually very high, so you normally dont have to worry about using too much memory.
since your array of numbers is an array of floats, you just multiple the size of the data type it stores (in this case float) by the length of the array. if you have 3 floats in your array you would multiple 4 * 3 to get 12. the size of your array is 12.

Vlatko .
2,526 PointsWow, I would've never realized that with the way the tutorial is set up. Thanks for the list of of sizes. I have to go back and update my notes now.
Mike Baxter
4,442 PointsHere's a little extra information for the more curious:
The way memory works is 0's and 1's. And the way computers count is by using a bunch of 0's and 1's all in a row (binary). The reason it's 0's and 1's has to do with storing electrical charge.
If you want any more than two lonely numbers—0 and 1—you have to start setting them next to one another.
A byte is 8 of these 0's and 1's set next to one another. Here's an example of a byte: 00101100. A byte has 256 possible combinations (2 to the power of 8). This is part of why you'll see RGB colors valued up to 256.
If you have four bytes, you have over 4 billion possible combinations. Computers actually do something cool and tricky with floats in C (or in Objective-C, all the same), but ignoring that, someone back in the history of computing decided it would make sense for a float to be 4 bytes (or 32-bits).
All "sizeof" is asking for in C is the number of bytes for that primitive float type. It doesn't matter what number you assign to that float, it's going to be 4 bytes regardless.
So now if you have some array of floats, and you ask it what it's "sizeof" is, the C language is only going to understand you as asking what the size of a general float is. It doesn't actually understand that you have an array; it thinks you're just asking about a float. So if you want to keep track of how large your array is (for example, if you ever do anything in OpenGL), you'll need to save an integer that refers to the size of the float array. For instance, it might look like this:
float myarray[] = malloc( sizeof(float) * 300 ); // suppose I have 300 elements in my array.
int myarray_size = sizeof(float) * 300; // or you could use size_t instead of int.

Vlatko .
2,526 Points[Deleted]
I don't want anyone to stumble upon my wrong conclusion and think it's correct by chance.

Roger Zurawicki
2,523 PointsBe careful.
Assuming a 32-bit processor
(float)0 has size 4 bytes (float)0.1 has size 4 bytes (float)11.11 has size 4 bytes (float)1234.5678 has size 4 bytes
The size of a float is constant, regardless of how many characters it has.
Vlatko .
2,526 PointsVlatko .
2,526 PointsThanks for correcting me on that, didn't realize that different variables has different sizes that were constant. I originally thought it was getting the float size from the numbers in the equation.
Anyway, wanted to ask why this in your example would still be 12 bytes:
If the float size is 4 bytes, and there are 4 float numbers, wouldn't the memory size be 16, not 12? I'm confused about this.
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsSorry, I did not mean to add to the confusion you already had. Thanks for the correction! That array would be 16 bytes. My intention was to show numbers with differing numbers of digits and how it would still be 12 bytes and I got carried away and added another number.
Vlatko .
2,526 PointsVlatko .
2,526 PointsAhh, gotcha. Thanks for the response!