Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial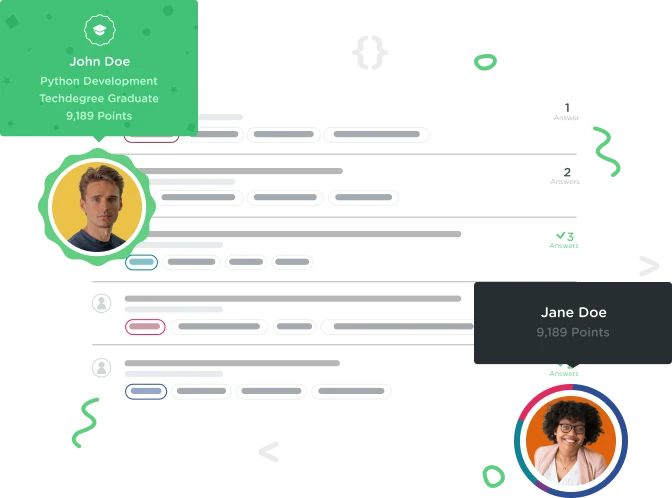

amandae
15,727 PointsHow to add an "and" before the last item of a joined list for readability?
I'm working on the list of school year birthdays in the "For Loops" practice session.
I'm curious, just for nice end-point formatting, how does one join a list with correct grammar.
Right now, I end up with:
Shawna, Amaya, Kamal, Xan have school year birthdays.
James, Sam have birthdays in the summer.
I would like it to say:
Shawna, Amaya, Kamal, and Xan have school year birthdays.
James and Sam have birthdays in the summer. (This would be easy if I knew ahead there were only two, but we don't know the number to start.)
Here's the code I have so far.
BIRTHDAYS = (
("James", "9/8", True, 9),
("Shawna", "12/6", True, 22),
("Amaya", "28/2", False, 8),
("Kamal", "29/4", True, 19),
("Sam", "16/7", False, 22),
("Xan", "14/3", False, 34),
)
sybdays = []
nsybdays = []
for e in BIRTHDAYS:
bday = e[1].split('/')
if int(bday[1]) <= 6 or int(bday[1]) >= 9:
sybdays.append(e[0])
else:
nsybdays.append(e[0])
print(f'{", ".join(sybdays)} have school year birthdays.')
print(f'{", ".join(nsybdays)} have birthdays in the summer.')
Thanks!
1 Answer

jb30
44,807 PointsI don't know if there is a built-in method for that. If you don't care about the serial comma and you know that there are at least two elements in each list, you could try
print(f'{", ".join(sybdays[:-1]) + " and " + sybdays[-1]} have school year birthdays.')
print(f'{", ".join(nsybdays[:-1]) + " and " + nsybdays[-1]} have birthdays in the summer.')
If you do care about the serial comma and don't know that each list has at least two elements, you could try
print(f'{", ".join(sybdays[:-1])}{"," if len(sybdays) > 2 else "" }{" and " if len(sybdays) > 1 else ""}{sybdays[-1]} {"have school year birthdays" if len(sybdays) != 1 else "has a school year birthday"}.')
print(f'{", ".join(nsybdays[:-1])}{"," if len(nsybdays) > 2 else ""}{" and " if len(nsybdays) > 1 else ""}{nsybdays[-1]} {"have birthdays" if len(nsybdays) != 1 else "has a birthday"} in the summer.')
[MOD: added ```python formatting -cf]
amandae
15,727 Pointsamandae
15,727 PointsThank you! I wondered if it was something like that, or if it had become a built-in. I appreciate the code examples.
jb30 and Chris Freeman - Thank you, again! I got the code to work with a combination of your ideas.
Chris Freeman
Treehouse Moderator 68,460 PointsChris Freeman
Treehouse Moderator 68,460 PointsI had a similar idea: