Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial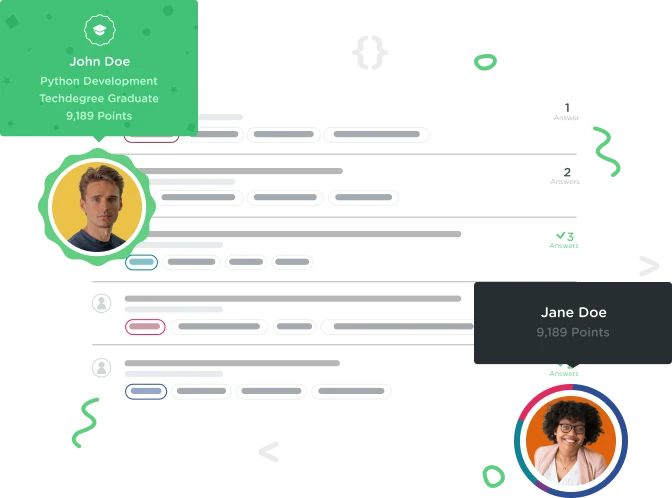
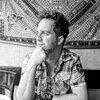
jlampstack
23,932 PointsHow would we write this loop NOT using a function?
I am revisiting this a year later and still having trouble understanding this. I know how to do it, but I simply don't understand. I keep getting crossed up with the $id and $Item.
Can anyone show an example WITHOUT using the function we created?
Thanks in advance!
1 Answer
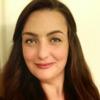
Jennifer Nordell
Treehouse TeacherHi there! Sure, we can do that!
<ul class="items">
<?php
foreach ($catalog as $id=>$item) {
// echo get_item_html($id,$item);
$output = "<li><a href='#'><img src='"
. $item["img"] . "' alt='"
. $item["title"] . "' />"
. "<p>View Details</p>"
. "</a></li>";
echo $output;
}
?>
</ul>
There you go. But I feel like it's not the code that's tripping you up or maybe even the variable names, but rather what is stored in that $catalog
. So I'm going to take a look at one at random.
$catalog[201] = [
"title" => "Forrest Gump",
"img" => "img/media/forest_gump.jpg",
"genre" => "Drama",
"format" => "DVD",
"year" => 1994,
"category" => "Movies",
"director" => "Robert Zemeckis",
"writers" => [
"Winston Groom",
"Eric Roth"
],
"stars" => [
"Tom Hanks",
"Rebecca Williams",
"Sally Field",
"Michael Conner Humphreys"
]
];
The $catalog is an array containing books, movies, and music. Each book, movie, or music resides at a particular index in that $catalog array. So it's just as well to use the index as the $id
as anything else, since it must be unique. Inside that entry is a set of key-value pairs. Those key-value pairs comprise the $item
as a whole.
In the above code, $id
will be equal to 201. That is the index at which this $item
is sitting.
Hope this helps somewhat!
jlampstack
23,932 Pointsjlampstack
23,932 PointsThanks Jennifer. This is a great explanation! The variable names were tripping me up. For the example I was talking about was for the index.php page for the array_rand($catalog, 4) exercise. This part of the exercise I understand. Are you able to help me with the index page? Thanks!
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse Teacherjaycode The part I posted in the first black box there is in the
index.php
page. The only thing I didn't do was do the random bit. But if you want that, too, then here we go:That
$random
variable now contains four "keys" or "indexes" (depending on how semantically correct you want to be). They are integers. And they represent 4 indexes that are currently present in our $catalog. So if it rolled 102, 201, 204, and 303, then we would expect to see "Clean Code: A Handbook of Agile Software Craftsmanship", "Forrest Gump", "The Princess Bride" and "No Fences". This is how that code would look without the function.So for each integer in the
$random
array, set that integer to be the value for$id
during this iteration. If the first number was102
then take a look at $catalog[102].On the first iteration: $catalog[$id]["title"] will be equal to "Clean Code: A Handbook of Agile Software Craftsmanship". Obviously, you could access any other properties you like here as well.
The next iteration of the loop it will get $catalog[201] and so forth.
Hope this helps clarify things!