Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial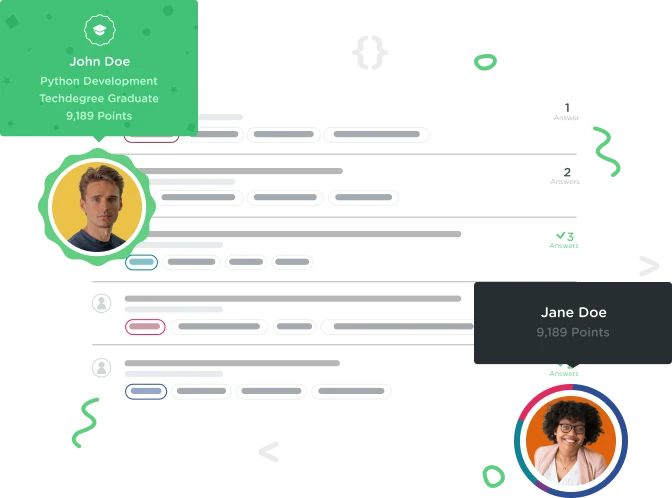

Pratham Patel
4,976 PointsI am having a hard time on approaching this code challenge
Could someone give me a hint
def sillycase(a):
a
2 Answers

Travis Bailey
13,675 PointsThis one can be really intimidating at first, but if you break down the problem it becomes a lot easier to approach.
First, you need to split the word in half. Then you need to lowercase the first half, and uppercase the second half. Finally, you combine the first and second half and return it. All of this is done in one function that takes in a string.
So you've got the function setup to take in one argument, so that's the first step solved. Next, you'll need to split the word in half. Keep in mind, you won't know the length of the word ahead of time. To do this, you'll use slices, which you've been learning about in this course. With slices, you need to use whole numbers. To make sure you get a whole number when finding the length and dividing it in half you'll want to either use int() which will round the result to the nearest whole number or (edit) integer division // which does the same thing. So how would that look?
example_word = "Treehouse"
len(example_word) #would return 9
int(len(example_word)/2) #would return 4
first = example_word[:int(len(example_word)/2)] #would store "Tree"
Next, you just need to use some tools from past lessons upper() and lower().
# continued from above example
lower = first.lower() #would store "tree"
upper = first.upper() #would store "TREE"
Then just combine the two strings in your return.

Christian Mangeng
15,970 PointsHi Pratham,
first try to calculate the index for the middle of the string (half). Then you can use something like:
string[:half].lower()
to lowercase the first half of the string. The same you can do with the other half, but uppercased.

Pratham Patel
4,976 PointsI tried that but I was having trouble finding half

Christian Mangeng
15,970 PointsCalculate the length of the string, convert the length to an integer, then use that integer to divide it by 2, using the rounded division (//). EDIT: // is integer division
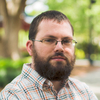
Kenneth Love
Treehouse Guest Teacher//
isn't rounded division, Christian Mangeng, it's integer division. 5 // 2
should be 3
but it'll come back as 2
because Python calculates 2.5
and then turns it into an integer, which is 2
.

Christian Mangeng
15,970 PointsAh, thank you for the correction, Kenneth Love!