Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial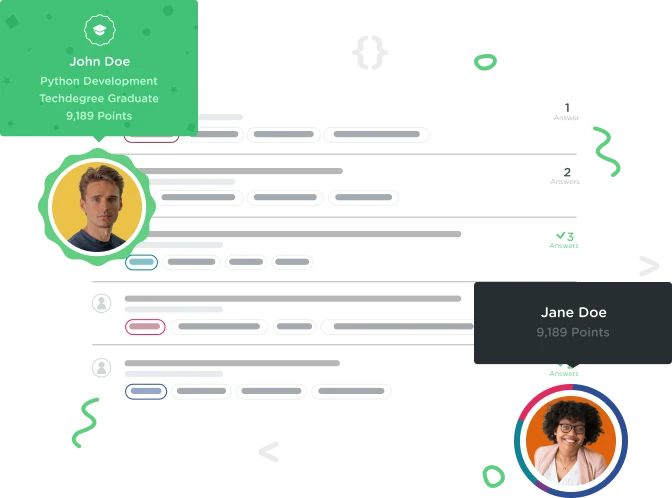

Ben Ella
17,872 PointsI get an error when I try to makemigrations and migrate
error:
django.db.migrations.exceptions.InconsistentMigrationHistory: Migration admin.0001_initial is applied before its dependency accounts.0001_initial on database 'default'.
My User model:
class User(AbstractBaseUser, PermissionsMixin):
email = models.EmailField(unique=True)
username = models.CharField(max_length=40, unique=True)
display_name = models.CharField(max_length=140)
avatar = models.ImageField(blank=True, null=True)
date_joined = models.DateTimeField(default=timezone.now)
is_active = models.BooleanField(default=True)
is_staff = models.BooleanField(default=False)
objects = UserManager()
USERNAME_FIELD = "email"
REQUIRED_FIELDS = ["display_name", "username"]
def __str__(self):
return self.display_name
def get_short_name(self):
return self.display_name
def get_full_name(self):
return "{} ({})".format(self.display_name, self.email)
def get_long_name(self):
return "{} ({})".format(self.display_name, self.email)
1 Answer
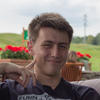
Jonatan Witoszek
15,384 PointsIn my case though deleting all other migrations (that step might have been unnesesary), removing django.contrib.admin
and path('admin/', admin.site.urls),
in msg/urls.py, then running migrations resolved the problem. You can add these again later to bring the admin back.
It is much bigger though:
This issue is described in Django Documentation
Quoting it:
Changing
AUTH_USER_MODE
L after you’ve created database tables is significantly more difficult since it affects foreign keys and many-to-many relationships, for example. Due to limitations of Django’s dynamic dependency feature for swappable models, the model referenced byAUTH_USER_MODEL
must be created in the first migration of its app (usually called0001_initial
); otherwise, you’ll have dependency issues.
In older version they've even stated:
Changing this setting after you have tables created is not supported by
makemigrations
and will result in you having to manually fix your schema, port your data from the old user table, and possibly manually reapply some migrations.
While researching this issue I've also found this great answer on StackOverflow.
You have to be extra-careful when doing this sort of things. As shown in the video, I have deleted all users this way and the new user I have created mapped all Kenneth posts to that user ¯_(ツ)_/¯
Fernando Cordeiro
34,597 PointsFernando Cordeiro
34,597 PointsOne year too late, but here's how I solved it:
__init__.py
s.python manage.py makemigrations
andpython manage.py migrate
Kenneth Love, is there any other, less drastic way?