Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial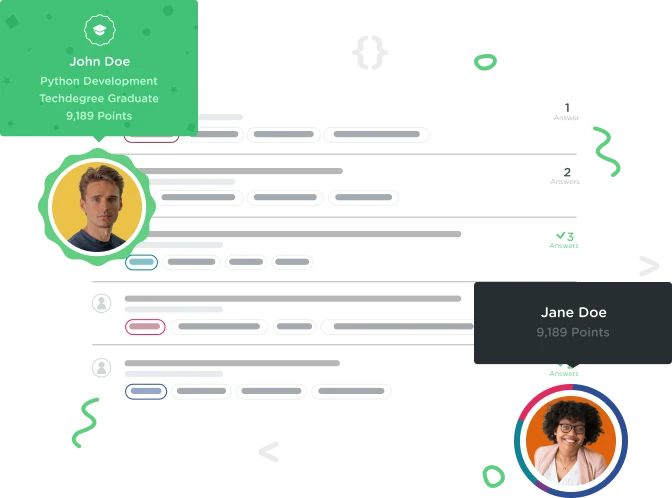

Matt Juergens
16,295 PointsI would Kenneth Love it if you would play and critique my dungeon game Kenneth Love.
Not a question, just following directions from the last video about adding features to "dungeon game". Definitely looking for ways to improve my code because it is UGLY and pretty slow. Also let me know if you like it. Kenneth Love
1 Answer
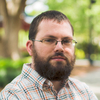
Kenneth Love
Treehouse Guest TeacherHey Matt!
First off, love the title. You really know how to pique my curiosity.
Secondly, that's a lot of code! I'm sure we can find a thing or two to improve. So, I'm gonna copy and paste all of your code into my editor (PyCharm) and see what it tells me.
- lines 117, 119, and 121 should probably be using
=
instead of==
- same for 187, 192, and 202
- oh, and 225, 230, and 235
OK, so, I fixed those things and reformatted the file to fit PEP 8's standards (if you haven't seen PEP 8 I highly recommend you read it. It's the guide for how your Python code should look). From this point on, line numbers wouldn't necessarily match up, so I'll post snippets of code.
elif cansee == True and cell == trap1:
output = tile.format("^")
elif cansee == True and cell == trap2:
output = tile.format("^")
While these lines are fine, it's usually best not to compare directly to True
or False
. You should rewrite these as:
elif cansee and cell == trap1:
output = tile.format("^")
elif cansee and cell == trap2:
output = tile.format("^")
Also, you have a few places with really long strings. Let's look at this one:
print(
"The 'X', '@', 'o', ']', and '^' symbols stand for the player, monster, switch, door and trap respectively")
Strings often feel really hard to break up but they're really not. You can break them into multiple lines and combine them with plus signs. Or, since it's inside of the print()
function, you can actually just stop one string and start the next one and Python'll join them up.
print("The 'X', '@', 'o', ']', and '^' symbols stand for the player, "
"monster, switch, door and trap respectively")
Now let's talk about your RPS
function. I love that you brought this in for battle. It's a great idea! There's a couple of places, though, where you wrote a bit more code than you needed to. I'll just show one example and I'm sure you'll see how to change the others.
if p_rps == "SCISSORS" and mon_rps == "PAPER":
Since you're always comparing these two things, you can save yourself some time by comparing tuples instead of each variable explicitly.
if (p_rps, mon_rps) == ("SCISSORS", "PAPER"):
Yes, it's still doing the same comparison, but it's a bit less to type and you don't have to remember that and
. You might be amazed how often and
and or
get used incorrectly or just forgotten. I love 'em but they're a pain in the butt sometimes.
Overall, great job!
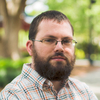
Kenneth Love
Treehouse Guest TeacherActually, those two trap comparisons could be even easier.
elif cansee and cell in (trap1, trap2):
output = tile.format("^")

Matt Juergens
16,295 PointsThanks a lot for the feedback! As I had guessed there was quite a bit wrong with my code. However I have just finished the OOP python course and the one covering PEP 8 so I plan to revise and improve my code greatly. Thank you for the multitude of suggestions I didn't actually expect to get such a great response. Keep up the good work, your python courses have been the most challenging and rewarding of the tracks I've been through. Building games is awesome.
Matt Juergens
16,295 PointsMatt Juergens
16,295 PointsHere's the code