Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial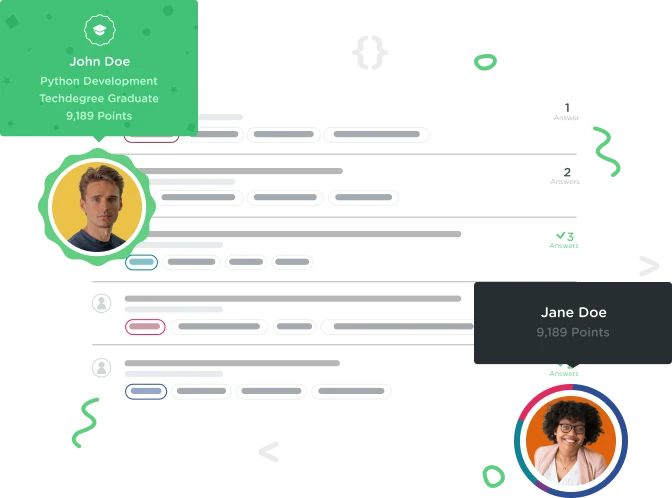
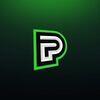
Pratham Mishra
14,191 PointsIncorrect format of rows and columns in Memory Game (OOP)
My final output doesn't show the exact format of rows and columns as demonstrated in the video.
This is my game.py file:
from cards import Card
import random
class Game:
def __init__(self):
self.size = 4
self.card_options = ['Add', 'Boo', 'Cat',
'Dev', 'Egg', 'Far', 'Gum', 'Hut']
self.columns = ['A', 'B', 'C', 'D']
self.cards = []
self.locations = []
for column in self.columns:
for num in range(1, self.size + 1):
location = f'{column}{num}'
self.locations.append(location)
def set_cards(self):
used_locations = []
for card in self.card_options:
for i in range(2):
random_location = random.choice(
list(set(self.locations) - set(used_locations)))
used_locations.append(random_location)
game_card = Card(card, random_location)
self.cards.append(game_card)
def create_row(self, num):
row = []
for column in self.columns:
for card in self.cards:
if card.location == f'{column}{num}':
if card.matched:
row.append(str(card))
else:
row.append(' ')
return row
def create_grid(self):
header = ' | ' + ' | '.join(self.columns) + ' |'
print(header)
for row in range(1, (self.size + 1)):
print_row = f'{row}| '
get_row = self.create_row(row)
print_row += ' | '.join(get_row) + ' |'
print(print_row)
def check_match(self, loc1, loc2):
cards = []
for card in self.cards:
if card.location == loc1 or card.location == loc2:
cards.append(card)
if cards[0] == cards[1]:
cards[0].matched = True
cards[1].matched = True
return True
else:
for card in cards:
print(f'{card.location}: {card}')
return False
def check_win(self):
for card in self.cards:
if card.matched is False:
return False
return True
def check_location(self, time):
while True:
guess = input(f"What's the location of your {time} card? ")
if guess.upper() in self.locations:
return guess.upper()
else:
print(
"That's not a valid location. Location should be column name then row name like A1.")
def start_game(self):
game_running = True
print('Memory Game')
self.set_cards
while game_running:
self.create_grid()
guess1 = self.check_location('first')
guess2 = self.check_location('second')
if self.check_match(guess1, guess2):
if self.check_win:
print("Congrats! You've guessed them all")
self.create_grid()
game_running = False
else:
input("Those cards are not a match. Please enter to continue")
print("GAME OVER!")
if __name__ == '__main__':
game = Game()
game.start_game()
Here's a look at my output:
Memory Game
| A | B | C | D |
1| |
2| |
3| |
4| |
What's the location of your first card?
1 Answer
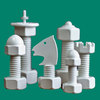
Steven Parker
229,744 PointsLine 80 is apparently intended to invoke self.set_cards, but the parentheses after the method name are missing.
Steven Parker
229,744 PointsSteven Parker
229,744 PointsIt's not clear what course and lesson you are referring to.
You might want to take a look at this video about Posting a Question.