Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial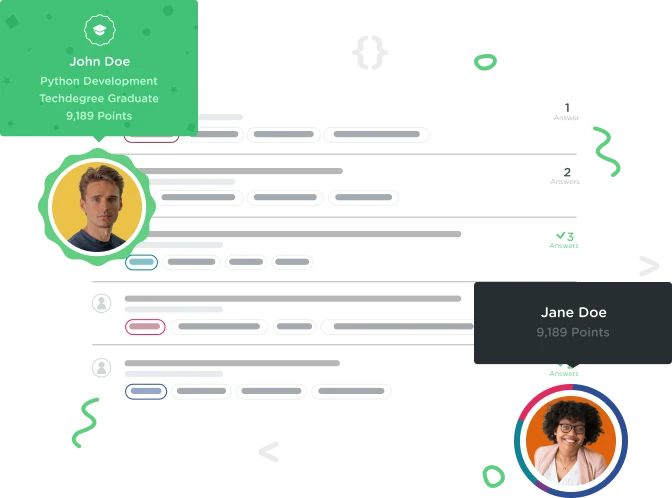
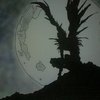
Lucas Santos
19,315 PointsInterface and Implementation?
So i'v been going through the Java Data Structure course and one thing I did not understand was when Craig was talking about Lists and Sets.
Example:
List<String> fruits = new ArrayList<>();
Set<String> people = new HashSet<>();
Craig in couple of the videos now keeps saying "We add the interface on the left and Implementation on the right"
I do not understand that.
So List & Set are both interfaces while ArrayList & HashSet are implementations. To my understanding Interfaces are a lot like a class but with contracts that you can imbed in a class with the keyword implements myInterface.
So this is where I am getting confused because why would you use an Interface as a type of an instance variable??
And not to mention that I do not know what an implementation is and what it's purpose is.
Can someone please explain Interface and Implementation?
Thank you.
1 Answer
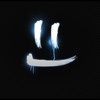
Grigorij Schleifer
10,365 PointsHi Lucas,
I had a similar question times ago. Craig and lain explain the topic very good !
Grigorij
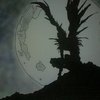
Lucas Santos
19,315 PointsAhh I see I think I got it so if im understanding this correctly it means that Interface and implementations are much like Super Classes and Sub Classes in the sense that when you are creating an instance of a Class you you can use a Super Class and an Interface as the type and Sub Class or Implementation as the reference.
Heres an example and correct me if im wrong, lets say we have this Super Class and Interface structure.
public class People{
int mAge;
String mName;
public People(int age, String name){
mAge = age;
mName = name;
}
}
public class Kids extends People{
String[] sports;
}
People is the super class and Kids is the sub class. Then we do.
interface List {
void returnStrings(String);
}
class ArrayList implements List{
public void String returnString(String){
String someString = "This is some string";
return someString;
}
}
List would be the interface and the class ArrayList implements that interface. Which means we can do this.
People ppl = new Kids(12, "Tom");
Because Kids is a sub class of people and,
List lst = new ArrayList("some args");
Because the class ArrayList implements List.
Am I correct?

Craig Dennis
Treehouse TeacherYeah I can see what you are selling ;) It's similar to subclassing in concept.
Think of interfaces more like defining behavior. It allows you to say "This object can do these things because it implements the interface." It signs a contract (by using the implements
keyword) that is checked at compile time to make sure that it can indeed do the things required by the interface. And then any code can say I need something that is guaranteed to be able to do these things specified in the interface.
It's such a great practice that the Java Collections Framework embraced it and allows you to not think about the underlying algorithms, but just what behavior the collection exposes. Not how it does that, that is left up to the implementation.
That help?
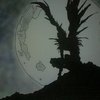
Lucas Santos
19,315 PointsThanks for the reply, I understand this much better now but just to be 100% sure and certain please answer one last question for me Craig Dennis
Is an Implementation considered ANY class that implements an interface with the "implements" key word?
Grigorij Schleifer
10,365 PointsGrigorij Schleifer
10,365 PointsThis is a sentence that describes the difference of both very good :