Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial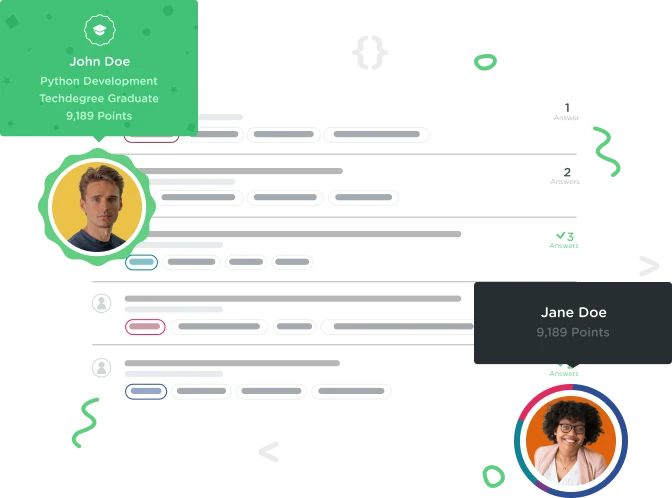

Anthony Scott
Courses Plus Student 9,001 Pointsjquery loop help
This is killin' me. I want to be able to use my Treehouse profile JSON feed to display a list of my badges and related info on a web page. I sort of got it working... this code does display a list of the badges but each badge is displayed multiple times. Can't figure out why. Maybe this isn't even the best solution. Thanks
var treehouseAPI = "https://teamtreehouse.com/anthonyscott4.json";
$.getJSON(treehouseAPI, function(json) {
var fullName = json.name;
console.log('Name: ', fullName);
var arr = [];
for(var x in json.badges) {
arr.push(json.badges[x])
var badgeList = $('ul.badgeList')
$.each(arr, function(key, val) {
var $li = $('<li>' + arr[x].name + '</li>');
$("#badgeList").append($li)
});
}
});
2 Answers
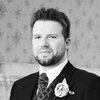
Joel Bardsley
31,249 PointsHi Anthony,
Let's go through your for loop to see what's happening:
for(var x in json.badges) {
arr.push(json.badges[x])
$.each(arr, function(key, val) {
var $li = $('<li>' + arr[x].name + '</li>');
$("#badgeList").append($li)
});
}
Let's say x = 0, you start off by retrieving the first badge (index [0]) from the json data and you push it to a separate arr array. Before the loop can start again to retrieve the next badge, you're already looping through your arr array and appending the badges to your unordered list. As x = 0, only the first badge is in your arr array (arr[0]) so it appends that to the list before the loop starts again.
Hopefully you now see why it's repeating your list items.
Here's my attempt if you want to use it as a reference:
var treehouseAPI = "https://teamtreehouse.com/anthonyscott4.json";
var badgeList = $('#badgeList');
$.getJSON(treehouseAPI, function(data) {
var badges = data.badges;
var arr = [];
// Retrieve badges and push them to a new array
$.each(badges, function(key) {
arr.push(badges[key]);
});
// Loop through array and append badge names as list items
$.each(arr, function(key) {
var $li = $('<li>' + badges[key].name + '</li>');
badgeList.append($li);
});
});
Demo on CodePen here. Hope that helps and good luck!

Anthony Scott
Courses Plus Student 9,001 PointsThank you, both! Very helpful.
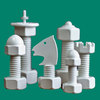
Steven Parker
230,688 PointsIt looks like you have a double loop.
For each badge in the data, you collect it into an array and then you put the entire array into your list. You can probably eliminate the inner loop and array, and just put each item directly into your list:
var treehouseAPI = "https://teamtreehouse.com/anthonyscott4.json";
$.getJSON(treehouseAPI, function(json) {
var badgeList = $("ul.badgeList");
for (var x in json.badges) {
var $li = $("<li>" + json.badges[x].name + "</li>");
$("#badgeList").append($li);
}
});
Steven Parker
230,688 PointsSteven Parker
230,688 PointsThis question appears to be related to this previous question.