Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial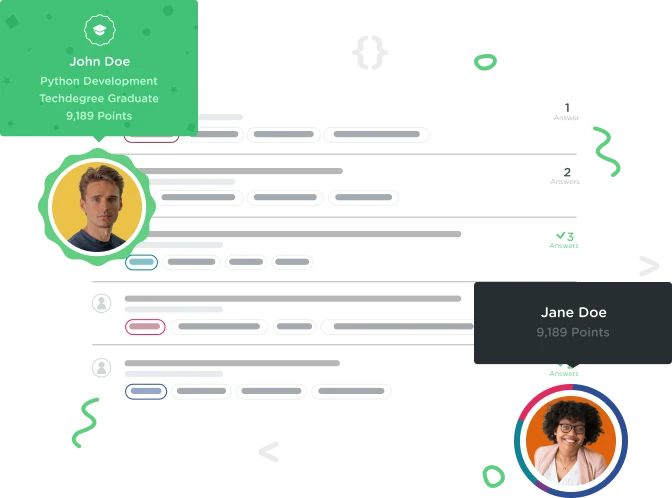

Anthony Scott
Courses Plus Student 9,001 PointsTrying to parse treehouse's JSON to get badge names.
I am trying to build off a node.js project here on Treehouse. Currently, it gets the number of badges and JavaScript points. I want to get the individual badges names and some other info. I can't figure out how to access the badge names. I am not dead set on the node path, it was just the closest example I had. Ultimately I want to display this info on a web page. Thanks!!
//require http module for status codes
const http = require('http');
//Require https module
const https = require('https');
//Print error messages
function printError(error) {
console.error(error.message);
}
//Function to print message to console
function printMessage(username, badgeCount, points, x) {
const message = `${username} has ${badgeCount} total badge(s) and ${points} points in JavaScript. here is a list of badges ${badgeNames}`;
console.log(message);
}
function get(username) {
try {
// Connect to the API URL (https://teamtreehouse.com/username.json)
const request = https.get(`https://teamtreehouse.com/${username}.json`, response => {
if (response.statusCode === 200) {
let body = "";
// Read the data
response.on('data', data => {
body += data.toString();
});
response.on('end', () => {
try {
// Parse the data
const profile = JSON.parse(body);
// Print the data
let arr = [];
for(var x in profile.badges) {
arr.push(profile.badges.name[x])
}
printMessage(username, profile.badges.length, profile.points.JavaScript, x);
} catch (error) {
printError(error); //catch error from non-existent user
}
});
} else {
const message = `There was an error getting the profile for ${username} (${http.STATUS_CODES[response.statusCode]})`;
const statusCodeError = new Error(message);
printError(statusCodeError);
}
});
request.on('error', printError);
} catch (error) {
printError(error);
}
}
module.exports.get = get;
2 Answers
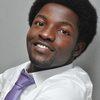
Emeka Okoro
Courses Plus Student 11,724 Pointstry this for(var x in profile.badges) { arr.push(profile.badges[x].name); } Also, you called printMessage with x as the last argument instead of arr call printMessage(username, profile.badges.length, profile.points.JavaScript, arr);
if you omit the (var x = 0; x < profile.badges.length; x++) in a for loop, the x variable is a counter that starts from 0. just inspected it without running it.

Anthony Scott
Courses Plus Student 9,001 PointsThanks, Emeka. That worked in Node. Steven, I decided to also try the same thing with jquery so I posted another question.
Steven Parker
231,271 PointsSteven Parker
231,271 PointsThis question appears to be extended with this later question.