Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial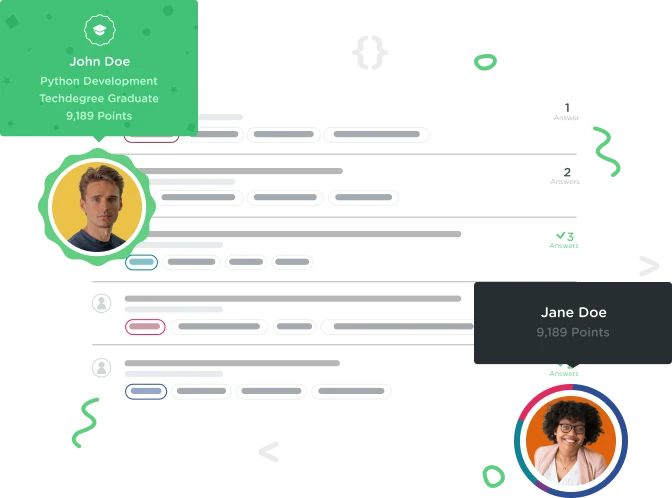

shekhar bhardwaj
Full Stack JavaScript Techdegree Student 12,373 PointsKenneth, dungeon game with debug mode and few other upgrades, let me know what do you think.
New functionalities
- User can move diagonally
- And if you input "debug" instead of return in the start of the program, program will enter into debug mode.
here's the git link
2 Answers

shekhar bhardwaj
Full Stack JavaScript Techdegree Student 12,373 PointsAwesome,
- Thanks for testing the code, I will fix the bug.
- I knew I had cyclomatic complexity with so many ifs, I should've used elifs, but I loved the switch case scenario.
Thanks alot for reviewing it. :)

Jon Mirow
9,864 PointsHi there!
haha good job!
Only bug I can see is that in debug mode, if the door or monster are at x == 4, they aren't drawn, but that's a simple fix :) I like it!
Only tip might be to look at those long if/if/if/if/if blocks? For example:
if move == "LEFT":
x -= 1
if move == "RIGHT":
x += 1
if move == "UP":
y -= 1
if move == "DOWN":
y += 1
if move == "XUPRIGHT":
x +=1
y -=1
if move == "XDOWNRIGHT":
x +=1
y +=1
if move == "XUPLEFT":
x -=1
y -=1
if move == "XDOWNLEFT":
x -=1
y +=1
This works fine of course, but calling if each time is giving more work for python to do. So say we move left. Python checks if move is left, and adjusts x as we want, but then it continues to check if move is right, then if move is up, then if move is down and so on until the end of the if statements. This is where you'd use an if/elif/elif block. If you do that, python will keep checking until it finds a match, then skip the rest of the block. This is the start of optimising your code's performance :)
Consider this example:
def check(string):
if string == "yep":
print("This one")
return True
else:
print("Not this one")
return False
print("Example 1:")
if check("yep"):
print("Entered if")
if check("nope"):
print("Entered if")
if check("nope"):
print("Entered if")
print("\n\nExample 2:")
if check("yep"):
print("Entered if")
elif check("nope"):
print("Entered if")
elif check("nope"):
print("Entered if")
Also, if you're interested in a bit of an advanced technique, This is basically a what other languages call a switch statement: the parameter Move will only ever be one of some pre-defined value, and you want to do an action based on which it of those it is. Python doesn't have switch statements, but it does have dictionaries, and we can use the key:value property of dictionaries just like switch in other languages. For your example:
moves_dict = {
"LEFT": (-1, 0),
"RIGHT": (1, 0),
"UP": (0, -1),
"DOWN": (0, 1)
}
x += moves_dict[move][0]
y += moves_dict[move][1]
But I think with that knowledge, you can take it a bit further and refactor some other parts too :)
Jon Mirow
9,864 PointsJon Mirow
9,864 PointsNo worries, and again great job! It's by making the projects that you really learn!