Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial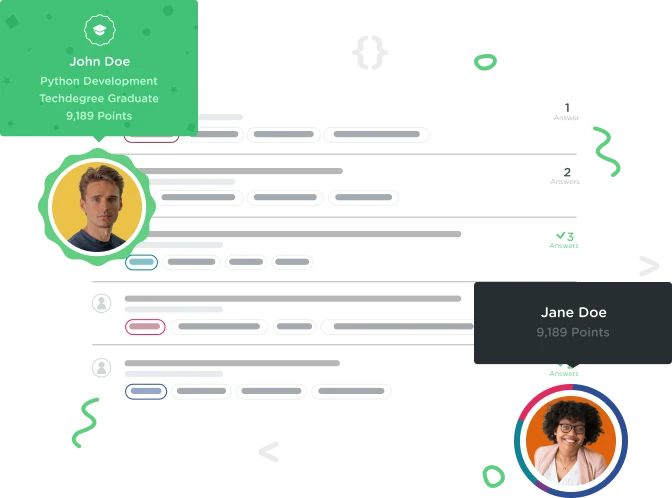
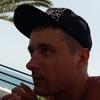
Nerijus Balakauskas
5,370 PointsLess code, no additional variables or functions, but result much the same. Is this ok in practice?
for (i=0; i < students.length; i +=1) {
for ( var key in students[i] ) {
document.write("<p>" + key + ": " + students[i][key] + "</p>");
}
document.write("<br>");
}
And this works. The only difference is that first line Student: name is not bolded. Thanks for comments.
1 Answer
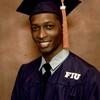
Dane Parchment
Treehouse Moderator 11,077 PointsWell your code would be fine, however it does one thing wrong: Time Complexity!
Programs generally have an execution time (in non mathematical terms) and some code that does the same thing will take longer to run than others. We refer to this time complexity as Big O Notation in computer science. It looks like this O(some value representing run time here). I know that this can be confusing to beginners but understanding time complexity can make the difference between a program that takes 10 nanoseconds to run and one that takes 10 years, and both do the same thing. There are three types of time complexity but the most important in my opinion is the Big O, which refers to the time that a program will take to run in its worst case scenario.
In the code that the instructor provides in the video his time complexity is: O(n). This is because at its worst-case scenario, it has to run through each student in the student array at least once. Meaning that his program run time will grow linearly with the number of students that are in the array. We call this complexity: Linear
Your code however, has a higher time complexity of: O(n^2) (it should read as "Oh en Squared"). Your program will run through each student, and then at each student run through a list of keys that the particular student has. This means that your program will not only grow based on the number of students in the list, but also the number of keys. This means that your program is running at the n - the number of students by n - the number of keys! In math terms it becomes: n x n = n^2. This gives us the time complexity of: O(n^2) which we call exponential!
Your program will take much longer to run than his if there are say 1000 students in the list.
If you need any more help understanding this read this. Or ask me for more assistance!
gyorgyandorka
13,811 Pointsgyorgyandorka
13,811 PointsDane Parchment Correct me if I'm wrong, but I don't really see the difference here - in Dave's version we just "hardcoded" the inner loop by writing out every operation line by line (we could do that because of the small number of properties), but the total number of operations is the same in either case (Big O-wise).
Dane Parchment
Treehouse Moderator 11,077 PointsDane Parchment
Treehouse Moderator 11,077 PointsTLDR; You are not wrong when you say that the two programs do the same thing, however, Nerijus' version is less efficient based on how he implemented it.
No not exactly, you need to look at this in terms of mathematics. Remember, the computer doesn't really care that the inner for-loop is doing the same thing that we can hard code, its time complexity will simply be based on how we go about telling it what to do.
In the instructors version the computer only has to go through one loop, again, when we look at how long it takes to run a loop mathematically it takes n amount of time (where n is the number of students). All the instructor does in the loop is print out variables. It only takes a constant amount of time for a computer to access a variable: 1. So assuming that each student only has 3 property variables his code will take: O(n) x O(1 + 1 + 1 = 3) = O(n) - since the 3 is so negligible compared to n, we ignore it.
However, in the students version, he is not taking just one loop, he is taking 2 loops. This means that one loop will have a time complexity of O(n) where n is the number of students, and the other will take O(m) where m is the number of keys each student has. Because the O(m) loop is taking place inside of the O(n) loop the time complexities are not added but are multiplied (that's how inner loops work). So his total time complexity becomes: O(n) x O(m) = O(n x m). At its worst case the number of keys each student has is equal to the number of students. In which case the time complexity becomes: O(n x n) = O(n^2). At its best it becomes O(n) x O(m) = O(n x m) where the m is not negligible and as such cannot be ignored. Either way both are worst in performance than the instructors. Hopefully this helps answer your question.
gyorgyandorka
13,811 Pointsgyorgyandorka
13,811 PointsDane Parchment You talk about 3 properties in the first case, then suddenly about n properties in the second case. The inner loop's time complexity is O(n), but if we assume that we have n porperties, and not a constant number, then the time complexity of the hardcoded version would also be O(n) - we're just making visible the same operations that the loop would do. On the other hand, if we have a constant number of properties, then the time complexity of the whole function will still be O(n) x O(1), it doesn't matter if we write down the lines manually or loop through that 3 properties (that loop's time complexity is linear, but since we feed it only with a specific (constant size) input, it will run in constant time).