Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial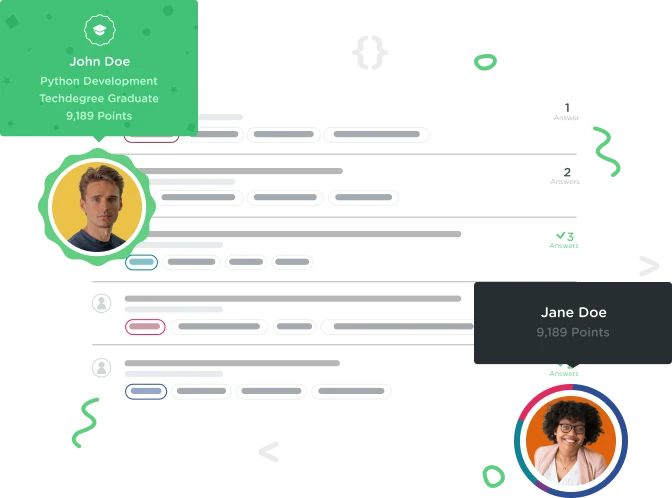

Greg Schudel
4,090 PointsLost....::sigh::...yet again
function randomNumBetween(){
var randomNumber1 = Math.floor(Math.random() * (6 - 1 + 1)) + 1;
var randomNumber2 = Math.floor(Math.random() * (6 - 1 + 1)) + 1;
if(randomNumber1 < randomNumber2){
alert("Looks like" + randomNumber1 + "is larger than" + randomNumber2);
}else (randomNumber1 > randomNumber2) {
alert("Looks like" + randomNumber2 + "is larger than" + randomNumber1);
}
};
I am trying to do this challenge and I'm completely lost as to how this was suppose to be easy to deduce. I understand my code is wrong above, but I would rather understand what I did wrong to not get the answer I need. I needed an upper and lower number. What and where in the previous videos were we taught on how to get an upper and lower random number? Is there anything up in my code that could be salvaged to answer the "Upper and lower " random number problem, or should it all be scrapped?
5 Answers
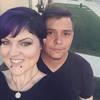
pastry assassin
Full Stack JavaScript Techdegree Student 11,693 Pointsfunction randomGenerator() { return Math.floor(Math.random() * 6 - 1 + 1) + 1; }
function randomNumBetween(one, two) { if (one > two) { return "Looks like " + one + " is larger than " + two; } else { return "Looks like " + two + " is larger than " + one; } }
var result = randomNumBetween(randomGenerator(), randomGenerator());
alert(result);
This seems to work for me. It looks like you have a condition to be tested in your "else" statement which I do not think is possible. If the code above doesn't make sense, just let me know and I will explain.

David Willis
Full Stack JavaScript Techdegree Student 18,600 PointsHi Greg,
I understand that you asked this question a while ago, but maybe I can help clarify where you went astray.
First, you're right. We didn't learn how to generate a random upper or lower number. However, the challenge isn't asking you to generate a random upper and lower number; instead, it wants you to create parameters for your randomNumBetween function. The video " Giving Information to Functions" teaches us how to insert parameters and then use them within the function.
function randomNumBetween(upper, lower) {
//Your code here
}
Second, by adding parameters users have the ability to determine the upper and lower values of the function for their own needs. For example, if I wanted the function to generate a number between 1 and 6 for my dice game, I would call the function with parameters filled like so:
randomNumBetween(6,1);
The function would then give me a random number between the two numbers I inputted into the function.
Third, Dave MacFarland gives us a hint as to what code should be put into the function in order to generate a random number between an upper and lower limit:
Math.floor(Math.random() * (6 - 1 + 1)) + 1;
As he demonstrated in the video "The Random Challenge Solution" in the "Working with Numbers" section, '6' is the upper limit while the ' - 1' is the lower limit. For the function to be changeable, you need to assign the code above to a variable, then return that variable at the end of the function. Be sure to switch the 6 and 1 with your parameters!
I hope this helps. Good Luck!
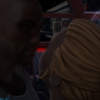
Jonathan Carroll
3,438 PointsAlso remember that the last + 1 in the Math.floor(Math.random() * (6 - 1 + 1)) + 1; generator needs to also be your second argument you passed into your second parameter. For example if I was trying to get a number between 10 and 20, "10" being my lower limit, 20 would be passed into where the 6 is originally and 10 would be passed into where the first 1 is in the generator example above. In addition, the 10 would also be used where the last +1 is in the formula. Hope this helps. Happy Coding.
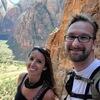
Jeremy Castanza
12,081 PointsHi Greg, I think it may have just been an understanding in the requirements in the project rather than your actual code having issues.
The goal of the project is to have a program that will take a variable range. Think of different types of dice. In the standard example, the code is using fixed values of 1 and 6, which is the range of a standard six sided die. You're basically updating the function to make it more flexible to accept different values from a user. For example, let's say they only wanted a random number between 1 and 4 for a four sided die or maybe for a D&D game, they have a die that has higher numbers. By doing this, the function now has more value since it can be used for more things.
To do this, you're only generating ONE random number between a set range - Not TWO random numbers. I think this is what threw you off. For example, a random number between 1 and 100, might be 57. Where as a random number between 1 and 6, might be four.
To do that, all you have to do is simply update the formula that generates your random value to include the range of values that may be of interest to the user.
Here's how I did it:
function randomGenerator( lower, upper ) {
if (isNaN(lower) || isNaN(upper)) {
throw new Error("An argument is not a number.");
}
var randomValue = Math.floor(Math.random() * (upper - lower + 1)) + lower;
return randomValue;
}
var lowerInput = prompt("Please enter lower number");
var upperInput = prompt("Please enter upper number");
// updated to convert string inputs to numbers
var lowerNumber = Number(lowerInput);
var upperNumber = Number(upperInput);
document.write( randomGenerator(lowerNumber, upperNumber) );
Beyond that, try not to get discouraged. There's a secret to programming that a lot of people don't know - most of your time spent programming is fixing bugs rather than actually coding. Best of luck to you!
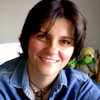
Paula Mourad
5,154 PointsI don't understand why we don't have to declare variables. Just be writing "upper" and "lower", how does the computer know what number will go there? I don't get it. I would have declared a var lower = 0 and a var upper=10000000
Help? :'(
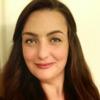
Jennifer Nordell
Treehouse TeacherHi Paula! Actually, you did declare the variables, although it might not be immediately obvious because of the way it works in JavaScript. When we define a function and list the parameters, those parameters are variable declarations for the scope of the function. Take a look at this example I made:
function sayHi(myName, otherName){
console.log("Hi there, " + otherName + "! My name is " + myName);
}
sayHi("Jennifer", "Paula");
If you run this in the console you'll see that it prints out "Hi there, Paula! My name is Jennifer". When we set up the function the myName
and otherName
act as variable declarations. Then when we call the function, we send in the data that we want to set the variables equal to. So myName
will be equal to "Jennifer" and òtherName
will be equal to Paula. While the function is executing these will act just like any other variables, but they will cease to exist the moment the function exits.
So when he declares and defines the function with this first piece of code:
function getRandomNumber( lower, upper) {
}
That is a function that will now take in two numbers. The numbers will be respectively assigned to lower and upper. And inside the body of that function you will have access to them just like any other variable. So even if you think you haven't declared those variables, you actually have, but only for that function.
Hope this clarifies things!
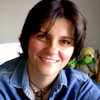
Paula Mourad
5,154 PointsThanks for the reply Jennifer. I will read your comment over and over until I get it. :) Thanks again!
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherTo begin with your markdown is a little odd looking here and sort of difficult to evaluate. However, I will say (without watching the full video again) that I'm fairly sure they want someone else to give the upper and lower numbers. That is to say that the user who is using this webapp/whatever will be sending the numbers. So you would want the function to accept two incoming numbers. So, if I write this: