Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial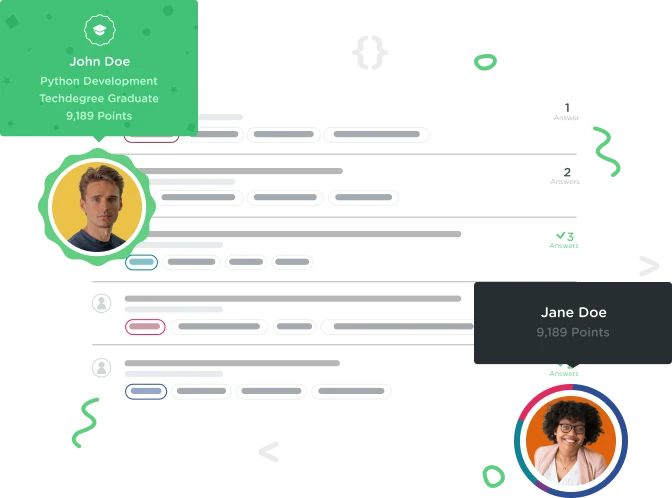

Jonell Alvi
4,259 Pointsminutes.py expected 5 got 55
I'm not sure what I'm doing incorrectly here, nor how to figure out the answer:
import datetime
def minutes(dt_1, dt_2):
return round(dt_1.minute - dt_2.minute)
The error is "Expected 5 got 55". I can pass the challenge by just subtracting 50 in my return statement, but I'm sure that isn't right.
What am I missing?
2 Answers
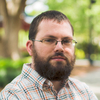
Kenneth Love
Treehouse Guest TeacherI'm really stuck on why this isn't working. Doesn't subtracting the datetimes create a timedelta? I don't need to return the hour, just the minutes in the timedelta, correct? Doens't timedelta mean the difference between the two times?
Yes, subtracting two datetime
s will get you a timedelta
but your code has you accessing the minute
of each datetime
before you're subtracting them. If datetime1
has a minute
of 55 and datetime2
has a minute
of 25, your code will give back 30, even if they were really 5 1/2 hours away from each other. You need to get the timedelta
first and then calculate the minutes from that.

Jonell Alvi
4,259 PointsThanks for helping me! I understand that I need to get the time delta first. Here's what I'm attempting:
import datetime
def minutes(dt_1, dt_2):
delta = (dt_1 - dt_2)
return round(delta.seconds / 60)
But it isn't returning the correct value to pass the exercise. I'd love to know how to figure this out on my own. It would really help in these exercises to know what the inputs are. Or some kind of hint. I've spent so much time on this and searching the forums for help.
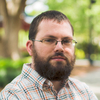
Kenneth Love
Treehouse Guest TeacherYou found a place where I need to provide more information. I'm going to update the CC in just a minute. Sorry about that!
EDIT: I've updated the challenge to explain a bit more. I thought the comment of "The first will always be older" would explain it but now I see that that wasn't nearly enough (thanks for finding that!).
Since the first datetime
is older, that makes it a smaller number. That means you need to do datetime2 - datetime1
, not datetime1 - datetime2
.

Jason Anello
Courses Plus Student 94,610 PointsHi Kenneth Love ,
Are we supposed to assume for this challenge that the 2 datetime's will be less than a day apart?
The code posted here does pass, once subtraction order is corrected, but I thought delta.seconds
would only give you the total number of seconds in a partial day. It seems like we also need to convert the number of days into minutes as well.
Something like return round(delta.days * 1440 + delta.seconds / 60)
I saw in the documentation that there was a total_seconds()
method which could make it cleaner.
return round(delta.total_seconds() / 60)
The docs also mentioned that this method was the same as doing td / datetime.timedelta(seconds=1)
Then I thought, if we need an answer in minutes then take the timedelta and divide by a timedelta that's a minute long.
return round(delta / datetime.timedelta(minutes=1))

Andrew VanVlack
20,155 PointsIn this challenge I got a timedelta by subtracting the two datetimes. I then used delta.second to get the seconds and divided by 60 to get the minutes. I really had to play with this one in the terminal to see what kind of numbers datetime and timedelta were giving me. Is there a cleaner way with some functions I am not seeing?
import datetime
def minutes (dt1, dt2):
delta = dt2 - dt1
return delta.seconds / 60
The reason you were getting a wrong answer is you are only looking at minutes, and not the hour, consider this.
if dt1 = 2:55 you are going to get 55 with .minute and dt2 = 3:00 you get 00 with .minute Then you are doing 55-00.
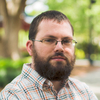
Kenneth Love
Treehouse Guest TeacherNope, the only way to get the difference is by making a timedelta.
Jonell Alvi
4,259 PointsJonell Alvi
4,259 PointsI'm really stuck on why this isn't working. Doesn't subtracting the datetimes create a timedelta? I don't need to return the hour, just the minutes in the timedelta, correct? Doens't timedelta mean the difference between the two times?
Please help?