Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial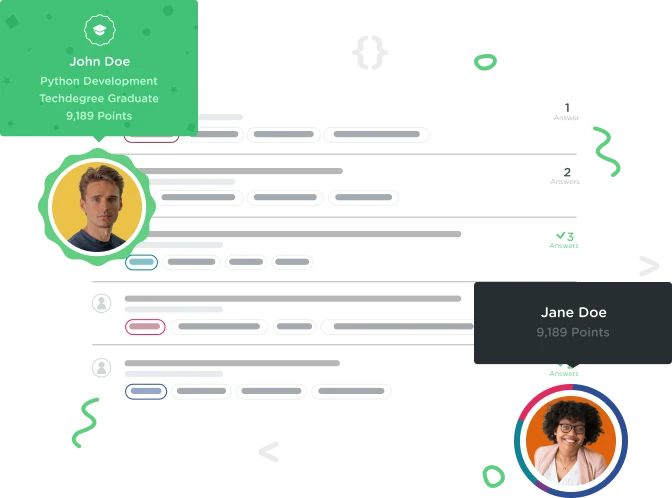
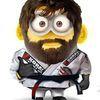
Jared Ledbetter
21,672 PointsMongoDB Connection Error
I'm working on this video: https://teamtreehouse.com/library/default-values-in-mongoose
Background: Last week, I purchased an iMac and migrated from a Windows 10 machine. On Win10, I didn't have this error. Now that I've migrated to Mac, I'm getting this error.
Initially, I thought I was missing NPM packages, so I reinstalled all of them. Then I thought it might have been my code, which likely isn't the issue because I didn't have the issue the week before using the same exact files.
'use strict';
var mongoose = require('mongoose');
mongoose.connect('mongodb://localhost:27017/sandbox');
var db = mongoose.connection;
db.on("error", function(err) {
console.error("Connection Error:", err);
});
db.once("open", function() {
console.log("Database connection successful");
// All database communication goes here
var Schema = mongoose.Schema;
var AnimalSchema = new Schema({
type: {type: String, default: "goldfish"},
color: {type: String, default: "small"},
size: {type: String, default: "golden"},
mass: {type: Number, default: 0.007},
name: {type: String, default: "Angela"}
});
var Animal = mongoose.model("Animal", AnimalSchema);
var elephant = new Animal({
type: "elephant",
size: "big",
color: "gray",
mass: 6000,
name: "Lawrence"
});
var animal = new Animal({}); // goldfish
Animal.remove({}, function() {
if (err) console.error("Save Failed", err);
elephant.save(function(err) {
if (err) console.error("Save Failed", err);
animal.save(function(err) {
if (err) console.error("Save Failed", err);
db.close(function() {
console.log("db connection closed");
});
});
});
});
});
When I run "node mongoose_sandbox.js" this is the error I receive in the console. I don't know how to use console syntax here.
(node:20191) DeprecationWarning: open()
is deprecated in mongoose >= 4.11.0, use openUri()
instead, or set the useMongoClient
option if using connect()
or createConnection()
. See http://mongoosejs.com/docs/4.x/docs/connections.html#use-mongo-client
Connection Error: { MongoError: failed to connect to server [localhost:27017] on first connect [MongoError: connect ECONNREFUSED 127.0.0.1:27017] at Pool.<anonymous> (/Applications/MAMP/htdocs/treehouse_rest_api_with_express/node_modules/mongodb-core/lib/topologies/server.js:336:35) at Pool.emit (events.js:198:13) at Connection.<anonymous> (/Applications/MAMP/htdocs/treehouse_rest_api_with_express/node_modules/mongodb-core/lib/connection/pool.js:280:12) at Object.onceWrapper (events.js:286:20) at Connection.emit (events.js:198:13) at Socket.<anonymous> (/Applications/MAMP/htdocs/treehouse_rest_api_with_express/node_modules/mongodb-core/lib/connection/connection.js:189:49) at Object.onceWrapper (events.js:286:20) at Socket.emit (events.js:198:13) at emitErrorNT (internal/streams/destroy.js:91:8) at emitErrorAndCloseNT (internal/streams/destroy.js:59:3) at process._tickCallback (internal/process/next_tick.js:63:19) name: 'MongoError', message: 'failed to connect to server [localhost:27017] on first connect [MongoError: connect ECONNREFUSED 127.0.0.1:27017]' }
1 Answer
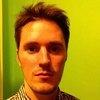
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsIt's been a while since I did this course, so I can't answer 100% confidently.
Mongo needs to be running. It's not a question of just having packages installed. There's a process that needs to be running on localhost:27017 for your app to talk to. I can't remember if it's running mongo or the mongo daemon but I suggest backtracking a bit in the course. You probably ran some command to start mongo a while back and it was just running in the background on your computer.
Ken Alger
Treehouse TeacherKen Alger
Treehouse TeacherAs Brendan Whiting pointed out, the mongo server needs to be running on port 27017 before running your application. In a terminal window run
mongod
to get the server running. After that you should be able to connect to it with your application(s).Jared Ledbetter
21,672 PointsJared Ledbetter
21,672 PointsThese files still don't work. But I made a copy of them into a different folder, and everything worked fine. At this point, I have no idea what is going on. But I appreciate the comment, and I'm glad I got it working "somehow".