Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial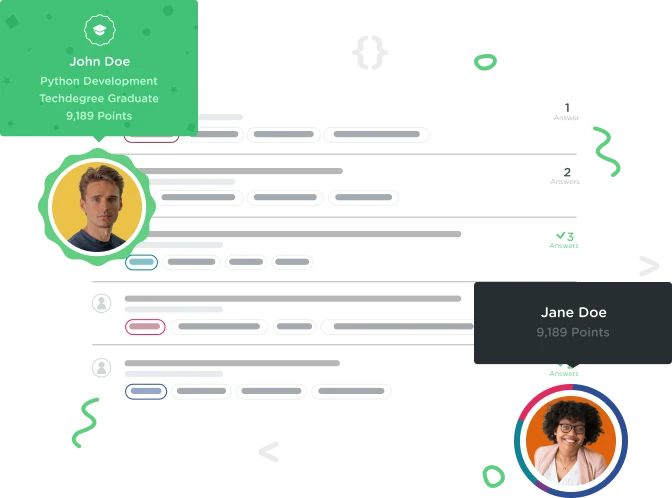
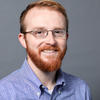
Kurt Maurer
16,725 PointsMorse code printer and list comprehension
I wanted to use a list comprehension for this exercise, but I was having trouble with the syntax and functionality. I ended up just using a normal for loop to get it to pass, but I'd be interested in some help with understanding how to get list comprehensions to work in this context.
This is what I was trying:
def __str__(self, pattern):
return '-'.join(['dot' if param is '.' else 'dash' if param is '_' for param in self.pattern])
2 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Kurt,
Before getting to the list comprehension, I wanted to mention that you would need to take out your second argument of pattern
to avoid getting an error and bummer message since it's not required. You only need to pass in self
.
Also, you used the is
operator in your code which happens to work out in this case but it's not the correct operator to use. You should be using ==
for equality comparison. The is
operator checks if 2 objects have the same identity.
When using an if/else
like that it's called a conditional expression When using these you always need an else to go with each if. This works similarly to how the ternary operator works in other languages if you're familiar with that.
It's possible to chain these together. In other words, the part after the else
can itself be another conditional expression.
So your syntax problem is that you have that 2nd if
but not an else
to go with it.
One way to fix your syntax problem is to add another else
:
return '-'.join(['dot' if param == '.' else 'dash' if param == '_' else param for param in self.pattern])
This is an example of a conditional expression within another conditional expression. This would have the effect of passing through the pattern item unchanged if it wasn't a dot or dash.
For the purposes of this code challenge though, you can assume you're only getting dots and dashes and you could simplify to a single conditional expression.
return '-'.join(['dot' if param == '.' else 'dash' for param in self.pattern])
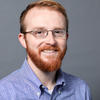
Kurt Maurer
16,725 PointsJason, This was an awesome response. Thank you so much for taking the time to answer my question; I'm definitely better for it!
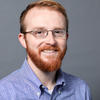
Kurt Maurer
16,725 PointsJust to go for the encore Jason, thank you again for an amazing answer. I understand is
vs ==
now, but I might have to visit a secluded monastery and meditate on string interning for a few days.
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsYou can check through this post here: https://teamtreehouse.com/community/why-a-b-it-returns-different-results if you'd like more details on
is
vs.==
as well as string interning.The blog post linked there which I'll repost here: http://guilload.com/python-string-interning/ covers the details of when strings are automatically interned and when they're not.
As it relates to your code, the strings '.' and '_' are automatically interned and so you would get the correct results using the
is
operator but for the wrong reasons.By contrast, a string with 2 dots ('..'), would not be automatically interned and the
is
operator would fail in that case if checking against that string.