Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial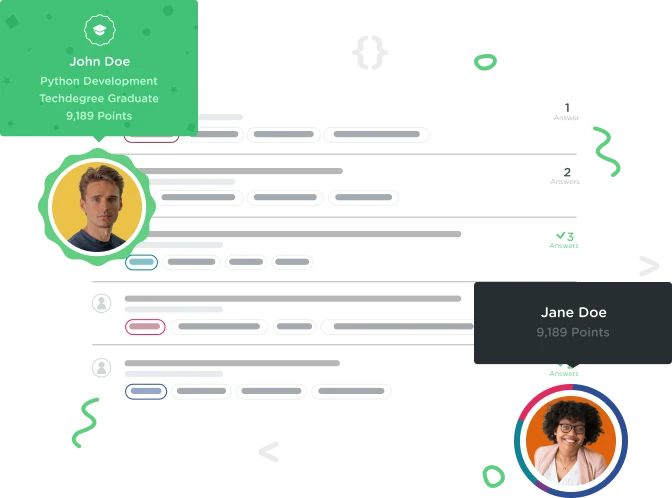

Saskia Lund
5,673 PointsMy solution to this challenge - based on my current JS learning state
Hey all!
Just wanted to share my solution to this challenge. Of course there must be better ways to solve this. However I could not come up with anything else. I think, that's because before starting the JavaScript course on Treehouse with Dave, I never wrote any JS myself... well I didn't even understand it. So I am quite proud of myself :)
/*
JavaScript course by Dave Farland on Treehouse.com
Author of this Code: Saskia Lund
Task: Quiz Challenge
URL: https://teamtreehouse.com/library/javascript-loops-arrays-and-objects/tracking-multiple-items-with-arrays/build-a-quiz-challenge-part-1
Date: June 11, 2015
*/
var correctCount = 0; // stores the number of correct answers the users provide.
var answer; // stores the user's answer.
var correctAnswer = []; // stores the correct answers and matching questions
var wrongAnswer = []; // stores wrong answer and matching questions
function print(message) {
document.write(message);
}
// array provides all quiz questions and answers
var quiz = [
[ "What's the current US president's first name?", 'barack' ],
[ 'Which film dog is named after a famous deaf composer and musician?', 'beethoven' ],
[ 'Where do the best soccer players come from?', 'germany' ],
[ 'How many toes do we have?', '20' ],
[ 'Who is playing the main male act in the movie "Braveheart"?', 'mel gibson' ]
];
// cycle through the quiz array and store the user input in the corresponding arrays ans variables
for ( var i = 0; i < quiz.length; i += 1 ) {
answer = prompt(quiz[i][0]);
if ( answer.toLowerCase() === quiz[i][1] ) {
correctAnswer.push('<li>' + quiz[i][0] + '<br>Your answer: ' + answer + '</li>');
correctCount += 1;
} else if ( answer.toLowerCase() !== quiz[i][1] ) {
wrongAnswer.push( '<li>' + quiz[i][0] + '<br>Correct answer would have been: ' + quiz[i][1] + ' </li>' );
}
}
// collect the answers for web output
var outputCorrectAnswer = correctAnswer.join(' ');
var outputWrongAnswer = wrongAnswer.join(' ');
// Let's tell the user his results
print( '<p>You got ' + correctCount + ' question(s) right.<br></p>' );
print( '<p>You got these questions correct:</p><ol>' + outputCorrectAnswer + '</ol><br>' );
if (wrongAnswer.length === 0) {
print('');
} else {
print( '<p>You got these questions wrong:</p><ol>' + outputWrongAnswer + '</ol>' );
}
I'd love to read suggestions for improvements of the code.
Hugs Saskia
P.S.: this is the challenge: https://teamtreehouse.com/library/javascript-loops-arrays-and-objects/tracking-multiple-items-with-arrays/build-a-quiz-challenge-part-1

Saskia Lund
5,673 PointsLOL!!
If you had not said it I had never recognized this XD Thank you :)

Iain Simmons
Treehouse Moderator 32,305 PointsWe have 20 toes...? Are our fingers really toes in disguise or something?
1 Answer
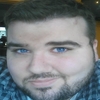
Marcus Parsons
15,719 PointsOh yeah, here is a codepen I made for someone else about a week or so ago that made their code DRYer and allows you to accept more than one answer per question. For example, in the 2nd question, it asks you if Hokkaido is in north Japan and correct answers can be given in several different languages. I put Japanese and Spanish versions of "yes" for extra answers but you could put as many as you want.

Saskia Lund
5,673 PointsThanks! I will have a look at it! Was also trying to get it done "dry". Well with my current knowledge. And I think that is still very very very limited. I started learning JavaScript just two days ago XD
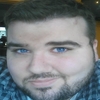
Marcus Parsons
15,719 PointsIt is very good for just having started using JavaScript! You should be proud.

Saskia Lund
5,673 PointsI am :))
Just looked at your code. I really like it. But I still don't know some of the snippets you used. I guess Dave will cover those in later videos (f.e. i++ etc).
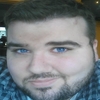
Marcus Parsons
15,719 PointsOh i++ is just a shorthand way of writing i += 1 which I'm sure you know by now is the same as writing i = i + 1. And the same thing with i-- which is just short for i -= 1 or i = i - 1.
If you have any more questions, I can cover those for you :)

Saskia Lund
5,673 PointsAh thanks for clearifying that one!
I just watched the solution video and am in the middle of Part 2 of the challenge, where he explains the dom and document.write etc
Hm, I really enjoy learning JavaScript. What a lucky coincidence that I found treehouse.com.
It is full of nice people who like to share their knowledge.
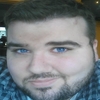
Marcus Parsons
15,719 PointsWell we're glad to have you here Saskia, and I hope you enjoy your stay! :) If you get stuck in anything in JavaScript, posting here at the forums will generally result in a very quick answer.

Iain Simmons
Treehouse Moderator 32,305 PointsBy the way Marcus Parsons, your code is great, but you could shorten the part about looking for the correct answer by using indexOf
instead of looping through the possible answers.
Basically, you just want to check if the quiz[i].indexOf(response)
> 0
, since that will check if the users response is the value matching one of the elements after the first in the inner array for that question.
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsHi Saskia,
Nice way to solve the challenge! :) You did get an answer wrong though because the president of the US's first name is Barack not Obama haha :P