Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial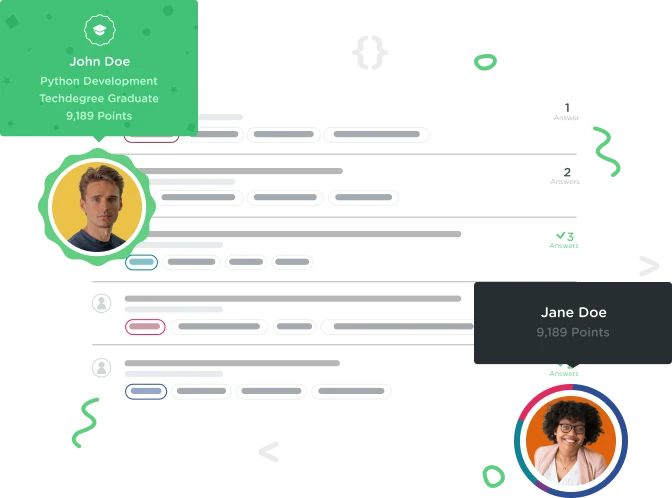
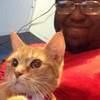
Emanuel Rouse
1,985 PointsMy solution.(Any suggestions to improve?)
Here is my code for the challenge.
var students = [
{
name: 'Dave',
track: 'Front End Development',
achievements: 158,
points: 14730
},
{
name: 'Jody',
track: 'iOS Development with Swift',
achievements: '175',
points: '16375'
},
{
name: 'Jordan',
track: 'PHP Development',
achievements: '55',
points: '2025'
},
{
name: 'John',
track: 'Learn WordPress',
achievements: '40',
points: '1950'
},
{
name: 'Trish',
track: 'Rails Development',
achievements: '5',
points: '350'
}
];
var name;
var track;
var achievements;
var points;
var html = ' ';
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
for(let i = 0; i < students.length; i += 1) {
name = students[i].name;
console.log(name);
html += '<h2>Students: ' + name + '</h2>';
print(html);
track = students[i].track;
html += '<p>' + 'Track: ' + track + '</p>';
print(html);
points = '<p>' + 'Points: ' + students[i].points + '</p>';
html += points ;
print(html);
achievements = students[i].achievements;
html += '<p>' + 'Achievements: '+ achievements + '</p>' ;
print(html);
}
Any tips on how to improve this? I tried coming up with a way to use the for in
technique however I couldn't figure out how to get the objects to show. My for in loop would just show the indexes of the objects such as 0, 1, 2, 3, 4 instead of the actual objects themselves. So I elected to go with a simple for loop.
3 Answers
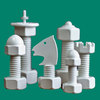
Steven Parker
229,783 PointsYou can easily translate your loop into a "for...in" like this:
// for(let i = 0; i < students.length; i += 1) { <- original
for (let i in students) {
Everything else should work as-is. Your other difficulties sound like you may have been coding the loop body to use a "for...of" (which iterates values) but ran it in a "for...in" (which iterates indexes). I'm still not sure I'd classify these changes as improvements though, while they do make the code a bit more compact the standard "for" loop is generally recommended for iterating through arrays. This is because it returns only the array elements, but a "for...in" will also return any user-defined properties.
But one definite improvement you could make is to call "print" only one time, after the loop. It's not necessary to call it multiple times inside the loop since the displayed text is completely replaced every time it is called.
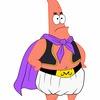
<noob />
17,062 PointsSteven Parker they didnt mention this type of loop. is it like in python?
for(let i in students)
{
if(i > 1)
{
bla bla?
}
}
another thing is in this videos they didnt use the ES6 version of javascript which became a standart. after i complete the basic videos i should watch the ES6 videos?
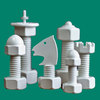
Steven Parker
229,783 PointsI don't like comparing features across languages (to easy to make incorrect inferences), but since a "for...in" iterates index values it would be roughly similar to "for i in range(len(iterable))
" in Python.
Yes, the ES6 videos are a good choice (but if you pursue a more advanced track at least one might be built into it).
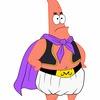
<noob />
17,062 Pointswhat do u mean by advanced track?
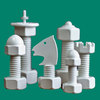
Steven Parker
229,783 PointsI was referring to the Tracks you see in the main menu, like Beginning JavaScript and Full Stack JavaScript.
Emanuel Rouse
1,985 PointsEmanuel Rouse
1,985 PointsThanks for your response! I did remove the extra calls to print function and tidied up my code. I just finished wrapping my head around the "for in" loop so I can actually use it correctly haha! I appreciate the help!