Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial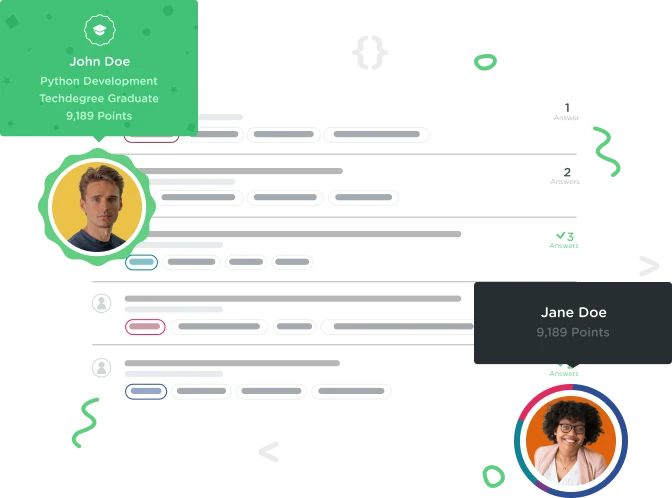
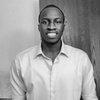
Modou Sawo
13,141 PointsNeed help with my shopping list app in JavaScript
When I preview the code, it takes the inputs, but doesn't print them out. Basically, the app is a simple app that takes input, join them and store them into an array. Here's the .js code
var my_shopping = [];
var count = 0;
var html;
function print(message) {
var outputDiv = document.getElementById('wegmens');
outputDiv.innerHTML = message;
}
// create a show_help function
function showHelp() {
var helpMessage = "<p>Hi, what are we getting today? </p>";
helpMessage += '<ul>';
helpMessage += "<li>Type 'help' to see these messages.</li>";
helpMessage += "<li>Type 'show' to see current list, or you can type 'quit' to exit the app.</li>";
helpMessage += '</ul>';
print(helpMessage);
}
// create a show_list function
function showList(arr) {
listHTML = '<ol>';
for (var i = 1; i < my_shopping.length; i += 1) {
listHTML += '<li>' + arr[i] + '</li>';
}
listHTML += '</ol>';
return listHTML;
}
// create a while-loop to enable adding items
// this while loop is not yet efficient!
while (true) {
var newItem = prompt('Enter items');
if ( newItem === 'quit') {
showList(my_shopping);
break;
} else if ( newItem === 'show' ) {
// toLowerCase() method later
showList(my_shopping);
break;
} else {
my_shopping.push(newItem);
}
}
// output
showHelp();
html = "Here are your items:";
html += showList(my_shopping);
print(html);
The HTML code where I referenced the wegmens id:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Shopping List</title>
<link rel="stylesheet" href="css/styles.css">
</head>
<body>
<div id="wegmens"></div>
<script src="js/shopping.js"></script>
</body>
</html>
3 Answers

Eric Butler
33,512 PointsHi again, I made a few changes and it's working correctly on my local setup, hopefully this works for you too:
var my_shopping = [];
function print(message) {
var outputDiv = document.getElementById('wegmens');
outputDiv.innerHTML = message;
}
// create a show_help function
function showHelp() {
var helpMessage = "<p>Hi, what are we getting today?</p>";
helpMessage += '<button id="start">Start</button>';
helpMessage += '<ul>';
helpMessage += "<li>Type 'help' to see these messages.</li>";
helpMessage += "<li>Type 'show' to see current list, or you can type 'quit' to exit the app.</li>";
helpMessage += '</ul>';
print(helpMessage);
}
// create a show_list function
function showList(arr) {
var listHTML = "Here are your items:";
listHTML += '<ol>';
for (var i = 0; i < arr.length; i++) {
listHTML += '<li>' + arr[i] + '</li>';
}
listHTML += '</ol>';
print(listHTML);
}
// create a while-loop to enable adding items
// this while loop is not yet efficient!
function startList(){
while (true) {
var newItem = prompt('Enter items');
if ( newItem === 'quit') {
showList(my_shopping);
break;
} else if ( newItem === 'show' ) {
// toLowerCase() method later
showList(my_shopping);
break;
} else {
my_shopping.push(newItem);
}
}
}
showHelp();
document.getElementById('start').addEventListener('click', startList, false);
I didn't change much, except I wrapped the prompt() in a function that only gets executed when you click the "start" button -- for some reason the page was executing that prompt before any other code, so nothing else could execute. Also you started your for loop with var i = 1, which actually omitted the first item in the array, because array counting starts at 0. Otherwise I just removed a few things that weren't being used. Let me know if this works for you and makes sense.

Eric Butler
33,512 PointsHi Modou,
I think the problem is in your showList
function. You have:
function showList(arr) {
listHTML = '<ol>';
for (var i = 1; i < my_shopping.length; i += 1) {
listHTML += '<li>' + arr[i] + '</li>';
}
listHTML += '</ol>';
return listHTML;
}
but the function doesn't know what my_shopping
is, so I think it needs to be arr
instead. So try:
function showList(arr) {
listHTML = '<ol>';
for (var i = 1; i < arr.length; i += 1) {
listHTML += '<li>' + arr[i] + '</li>';
}
listHTML += '</ol>';
return listHTML;
}
and see if that solves it for you. Let me know if it does!
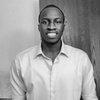
Modou Sawo
13,141 PointsHi Eric, thanks for the quick reply. I tried implementing that, but the items still don't show up.
I was trying do what we did in Python basics in this video with Kenneth Love : https://teamtreehouse.com/library/python-basics/shopping-list-app/second-shopping-list-app

goerge esrtregan
249 PointsHi, I hope this can solve your problems,
```function showList(arr) { listHTML = '<ol>'; for (var i = 0; i < arr.length; i += 1) { listHTML += '<li>' + arr[i] + '</li>'; } listHTML += '</ol>'; print(html); //notice that i change this to print into print function instead of returning it.
and at the last part of the code
//showHelp(); html = "Here are your items:"; html += showList(my_shopping); print(listHTML); // this will display your added items in the div
also i notice about your showHelp functions, it just display the in text i guess it is good if you make it in prompt() function too.. I hope this helps, sorry i am not good in explaining things, I'm just learning the basics and trying to help you.
Modou Sawo
13,141 PointsModou Sawo
13,141 PointsEric, you're genius! lol Thanks a lot! The button really made it colorful, I should've thought of that. I'll work on it and try to do it on my own over and over again, until it's second nature.