Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial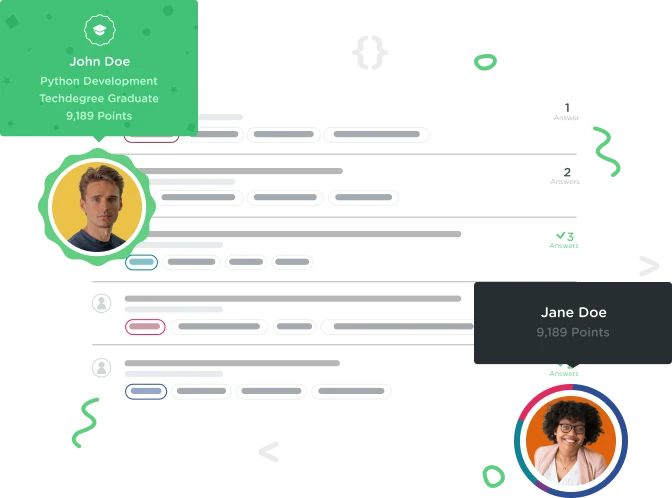

Riley Wu
1,843 PointsNeed some help with Method
class Car(): doors = 4 wheel = 4 engine = True
def __init__(self, model, year, make = "Ferrari"):
self.model = model
self.year = year
self.make = make
self.gas = 1000
self.is_moving = False
def stop(self):
if self.is_moving:
print("The car has stopped")
self.is_moving = False
else:
print("The car has already stopped")
def go(self, speed):
if self.use_gas():
if not self.is_moving:
print(f"The car has started")
self.is_moving = True
print(f"the car is going {speed} KMH")
else:
print("You are run out of gas!")
self.stop()
def use_gas(self):
self.gas -= 10
if self.gas <= 0:
return False
else:
return True
car_one = Car("F40",2022) car_one.go(100) car_one.stop() car_one.go(200) car_one.go(250) car_one.stop()
This is what is printed in the console
"The car has stopped
The car has started
the car is going 200 KMH
You are run out of gas!
The car has stopped
The car has already stopped "
I have used the method function by the instructor. But I do not understand 1) why the car has stopped when 1st go method is called 2) Why the car has stopped and run out of gas after 200 KMH even though there is still gas left.
Thanks a lot !
1 Answer
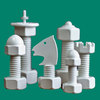
Steven Parker
230,274 PointsThe output from the first call to "go" is not shown here. That outputs:
The car has started
the car is going 100 KMH
The output shown begins with the first call to "stop".
Then, take a look at the "go" method:
def go(self, speed):
if self.use_gas():
if not self.is_moving:
print(f"The car has started")
self.is_moving = True
print(f"the car is going {speed} KMH")
else:
print("You are run out of gas!")
self.stop()
Notice that the "else" lines up with "if not self.is_moving:". This means that if the car is already moving (as it would be after the 2nd call to "go"), the output reports "out of gas" (even though it's not) and a stop is forced.
Also, the indentation seems inconsistent, which could cause an error on some Python implementations. Even if not, consistent indentation might help make errors such as this easier to spot.

Riley Wu
1,843 PointsThanks a lot !
Steven Parker
230,274 PointsSteven Parker
230,274 PointsTake a look at these videos about Posting a Question, and using Markdown formatting to preserve the code's appearance.