Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial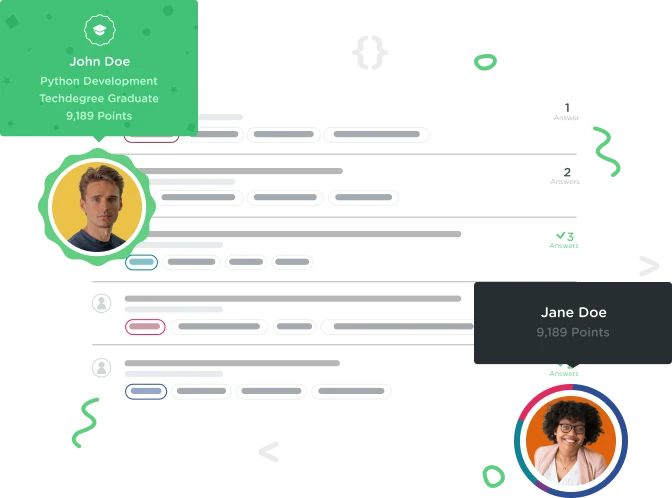

Iain Simmons
Treehouse Moderator 32,305 PointsNo need for jsonify
Hi Kenneth Love, I believe Flask-RESTful will automatically return JSON from a Python dict, so your initial setup of the resources would look like this:
from flask.ext.restful import Resource
import models
class CourseList(Resource):
def get(self):
return {'courses': [{'title': 'Python Basics'}]}
class Course(Resource):
def get(self, id):
return {'title': 'Python Basics'}
def put(self, id):
return {'title': 'Python Basics'}
def delete(self, id):
return {'title': 'Python Basics'}
and
from flask.ext.restful import Resource
import models
class ReviewList(Resource):
def get(self):
return {'reviews': [{'course': 1, 'rating': 5}]}
class Review(Resource):
def get(self, id):
return {'course': 1, 'rating': 5}
def put(self, id):
return {'course': 1, 'rating': 5}
def delete(self, id):
return {'course': 1, 'rating': 5}
Hopefully you don't just point this out in the following video... :)
2 Answers
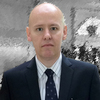
Nathan Tallack
22,160 PointsNice!!!

Sami Mäntysaari
55 PointsExactly what I was wondering too. :)
Also why is this course using flask_restful when there's a better one called flask_restplus?