Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial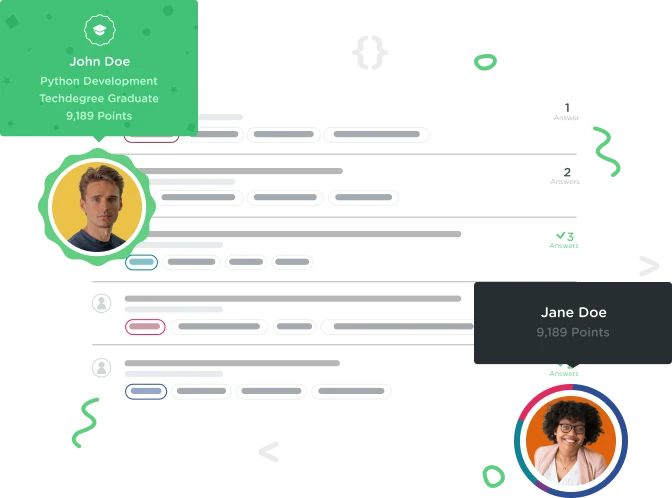
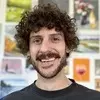
Joseph Guerra
20,674 PointsNode.js Weather Forecast Extra Credit (wunderground)
Here's the extra credit for Node.js basics: a command line weather forecast tool.
I used the Weather Underground API, instead of the forecast.io API for a different spin on this assignment. Truthfully, I couldn't figure out how to pass forecast.io a zip. They only accept lat / lon. So I used wunderground for zip direct :)
But this student cleverly used the Google Maps API to enter a zip and pass forecast.io a lat / lon. Nice job!
Full source code on for wunderground version below, and on github: https://github.com/josephjguerra/node-weather-forecast-command-line
I put my wunderground API key in a separate file with var apiFile = require('./apiFile');
// Problem: I want the weather forcast!
// Solution: Use node js to connect to wunderground api and show the weather forecast
// Sign up for your own free wunderground developer key at https://www.wunderground.com/weather/api/
// Requires
var http = require('http');
var apiFile = require('./apiFile'); // apiKey in separate apiFile.js file to protect it :)
// function to print out the weather
function printWeather(zip, forecast) {
var message = 'Your weather forcast is: ' + forecast;
console.log(message);
};
// function to print out errors
function printError(error) {
console.error(error.message);
if (error.message === "Cannot read property 'txt_forecast' of undefined") {
console.log('Try entering a real 5-digit zip!');
};
};
// Connect to wunderground API
function getForecast(enteredZip) {
var request = http.get('http://api.wunderground.com/api/' + apiFile.apiKey + '/forecast/q/' + enteredZip + '.json', function(response) {
// console.log(response.statusCode); // for testing to see the status code
var body = ''; // start with an empty body since node gets responses in chunks
// Read the data
response.on('data', function(chunk) {
body += chunk;
});
response.on('end', function() {
if ( response.statusCode === 200 ) {
try {
// Parse the data
var forecast = JSON.parse(body);
//console.dir(forecast); // for testing to see the JSON response
// Print the data
printWeather(enteredZip, forecast.forecast.txt_forecast.forecastday[0].fcttext);
} catch(error) {
// Print any errors
printError(error);
};
} else {
// Status code error
printError({message: 'OOPS! There was a problem getting the weather! (' + response.statusCode + ')'});
};
});
});
// Print connection error
request.on('error', printError);
// end getForecast function
};
// uncomment below if you want to run this file from the command line. ex: node forecast.js 28285
getForecast(process.argv); // process.argv lets you pass a zip in command line. ex: node forecast.js 28285
// uncomment below if you want to run this forecast from a separate file. ex: node app.js 28285
// module.exports.getForecast = getForecast;