Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial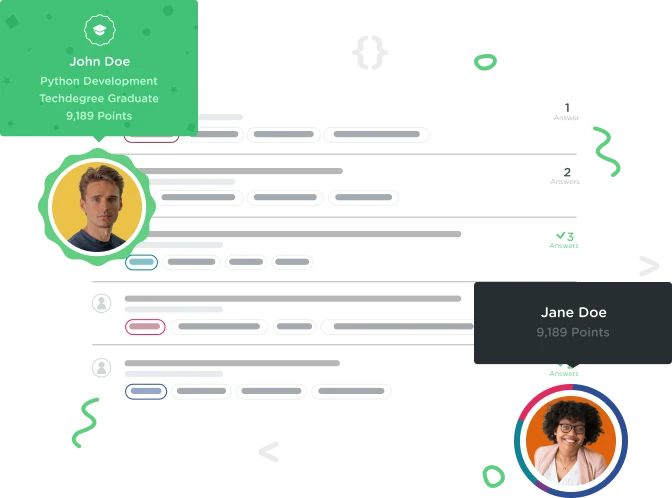

diginoma
Courses Plus Student 8,009 PointsNothing showing up in my console...
class Game:
def __init__(self):
self.size = 4
self.card_options = ['add', 'boo', 'cat', 'dev',
'egg', 'far', 'gum', 'hut']
self.columns =['A', 'B', 'C', 'D']
self.cards = []
self.locations = []
for column in self.columns:
for num in range(1, self.size + 1):
print(f'{column}{num}')
if __name__ == '__main__':
Game()
At this point in the video, the instructor ran the file and got all the locations available in the game. I'm not getting that result and can't figure out why...no errors just...nothing!
[MOD: added ```python formatting -cf]
3 Answers
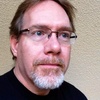
Chris Freeman
Treehouse Moderator 68,460 PointsHey diginoma, after adding formatting to your post, the issue becomes clearer.
The if __name__ == “__main__”:
is indented too far. The “if” should align with the “class”
As the code is now, the if
is inside the class definition. When run from the command line, the class is defined but nothing else is executed.
Post back if you need more help. Good luck!!!

diginoma
Courses Plus Student 8,009 Pointshow did you get it to be formatted properly btw?
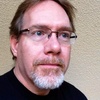
Chris Freeman
Treehouse Moderator 68,460 PointsI wrapped the code with ```python and ```
There needs to be a blank line before and after the whole block.
More details in the Markdown Cheatsheet link show below new and edited posts

diginoma
Courses Plus Student 8,009 Pointspython and
class Game: def init(self): self.size = 4 self.card_options = ['add', 'boo', 'cat', 'dev', 'egg', 'far', 'gum', 'hut'] self.columns = ['A', 'B', 'C', 'D'] self.cards = [] self.locations = [] for column in self.columns: for num in range(1, self.size + 1): self.locations.append(f'{column}{num}')
def set_cards(self):
used_locations = []
for word in self.card_options:
for i in range(2):
available_locations = set(self.locations) - set(used_locations)
random_location = random.choice(list(available_locations))
used_locations.append(random_location)
card = Card(word, random_location)
self.cards.append(card)
def create_row(self, num):
row = []
for column in self.columns:
for card in self.cards:
if card.location == f'{column}{num}':
if card.matched:
row.append(str(card))
else:
row.append(' ')
return row
def create_grid(self):
header = ' | ' + ' | '.join(self.columns) + ' |'
print(header)
for row in range(1, self.size + 1):
print_row = f'{row}| '
get_row = self.create_row(row)
print_row += ' | '.join(get_row)
print(print_row)
def check_match(self, loc1, loc2):
cards = []
for card in self.cards:
if card.location == loc1 or card.location == loc2:
cards.append(card)
if cards[0] == cards[1]:
cards[0].matched = True
cards[1].matched = True
return True
else:
for car in cards:
print(f'{card.location}: {card}')
return False
def check_win(self):
for card in self.cards:
if card.matched == False:
return False
else:
return True
def check_location(self,time):
while True:
guess = input(f"What's the location of your {time} card? ")
if guess.upper() in self.locations:
return guess.upper()
else:
print("That's not a valid location")
if name == 'main': game = Game() game.set_cards() game.create_grid() if name == 'main': game = Game() game.set_cards()
python and
just for practice

diginoma
Courses Plus Student 8,009 Pointsum....with or without the quote marks?
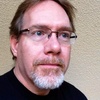
Chris Freeman
Treehouse Moderator 68,460 PointsIt needs to be:
Blank before start of code block
```python
your code in the middle
```
Blank line following close of code block
to yield
your code in the middle
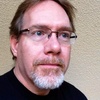
Chris Freeman
Treehouse Moderator 68,460 PointsYou can also use the ellipsis ...
below your post I edited, and select “edit post” to see the raw formatting changes.
diginoma
Courses Plus Student 8,009 Pointsdiginoma
Courses Plus Student 8,009 PointsThanks!!
diginoma
Courses Plus Student 8,009 Pointsdiginoma
Courses Plus Student 8,009 PointsFinally figured out how to post my code for help! Thanks again Chris!!!!