Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial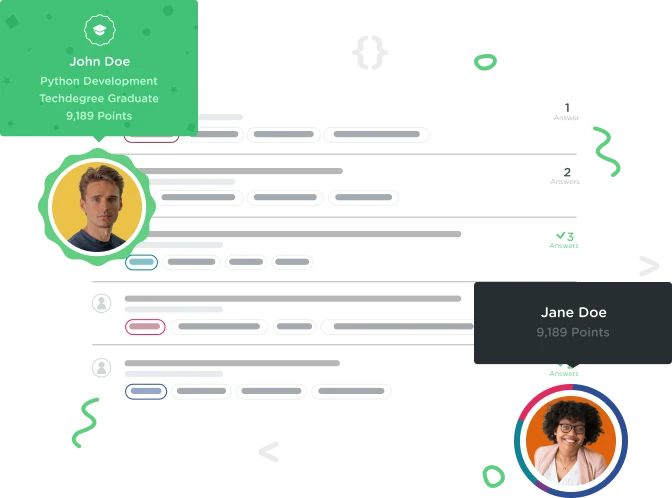

Aldrin Cabuniag
3,783 PointsNull Pointer Exception
I've been trying to find what was wrong with my code as I've been follwing step by step of the video of the Weather App project however, once I try to start the app there is an error:
Attempt to invoke virtual method 'void android.widget.TextView.setText(java.lang.CharSequence)' on a null object reference at com.teamtreehouse.auxo.MainActivity.updateDisplay(MainActivity.java:104) at com.teamtreehouse.auxo.MainActivity.access$200(MainActivity.java:27) at com.teamtreehouse.auxo.MainActivity$1$1.run(MainActivity.java:79)
I've started with updateDisplay and from the looks of it, there isn't any obvious signs for me -however I may be overlooking the obvious answer. Any suggestions would help! Thank you.
Here are some bits of my code:
private CurrentWeather mCurrentWeather;
@BindView(R.id.timeLabel) TextView mTimeLabel;
@BindView(R.id.temperatureLabel) TextView mTemperatureLabel;
@BindView(R.id.humidityValue) TextView mHumidityValue;
@BindView(R.id.precipValue) TextView mPrecipValue;
@BindView(R.id.summaryLabel) TextView mSummaryLabel;
@BindView(R.id.iconImageView) ImageView mIconImageView;
. . .
private void updateDisplay() {
mTemperatureLabel.setText(mCurrentWeather.getTemperature() + "");
mTimeLabel.setText("At " + mCurrentWeather.getFormattedTime() + "it will be");
mHumidityValue.setText(mCurrentWeather.getHumidity() + "");
mPrecipValue.setText(mCurrentWeather.getPrecipChance() + "%");
mSummaryLabel.setText(mCurrentWeather.getSummary());
}
MainActivity.xml:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/activity_main"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.wethebest.auxo.MainActivity"
android:background="@android:color/holo_blue_light">
<TextView
android:text="--"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/temperatureLabel"
android:textColor="@android:color/background_light"
android:textSize="150sp"
android:layout_centerVertical="true"
android:layout_centerHorizontal="true"/>
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:srcCompat="@drawable/degree"
android:layout_alignTop="@+id/temperatureLabel"
android:layout_alignRight="@+id/temperatureLabel"
android:layout_alignEnd="@+id/temperatureLabel"
android:layout_marginTop="50dp"
android:id="@+id/imageView"
android:layout_marginLeft="50dp"
android:layout_marginStart="5dp"
android:layout_marginBottom="5dp"
/>
<TextView
android:text="--"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/timeLabel"
android:textColor="#7bffffff"
android:textSize="18sp"
android:layout_above="@+id/temperatureLabel"
android:layout_centerHorizontal="true"/>
<TextView
android:text="Vancouver, BC"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/locationLabel"
android:textColor="@android:color/background_light"
android:textSize="24sp"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_marginTop="59dp"/>
<TextView
android:text="Stormy with a chance of feels."
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/summaryLabel"
android:textColor="@android:color/background_light"
android:textAlignment="center"
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"
android:layout_marginBottom="48dp"
android:freezesText="false"
android:gravity="center"/>
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:srcCompat="@drawable/cloudy_night"
android:id="@+id/iconImageView"
android:layout_marginTop="16dp"
android:layout_below="@+id/locationLabel"
android:layout_centerHorizontal="true"/>
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="17dp"
android:layout_below="@+id/temperatureLabel"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true">
<LinearLayout
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1">
<TextView
android:text="HUMIDITY"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/humidityLabel"
android:layout_below="@+id/temperatureLabel"
android:textColor="@android:color/background_light"
android:textSize="14sp"
android:textAlignment="center"
/>
<TextView
android:text="--"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/humidityValue"
android:textColor="@android:color/background_light"
android:textAlignment="center"
android:textSize="24sp"/>
</LinearLayout>
<LinearLayout
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1"
android:id="@+id/linear">
<TextView
android:text="PRECIPITATION"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/precipLabel"
android:layout_below="@+id/temperatureLabel"
android:layout_alignParentRight="true"
android:layout_alignParentEnd="true"
android:textColor="@android:color/background_light"
android:textSize="14sp"
android:textAlignment="center"
/>
<TextView
android:text="--"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/precipValue"
android:textColor="@android:color/background_light"
android:textAlignment="center"
android:textSize="24sp"/>
</LinearLayout>
</LinearLayout>
</RelativeLayout>

Aldrin Cabuniag
3,783 PointsLine 104 is exactly:
mTemperatureLabel.setText(mCurrentWeather.getTemperature() + "");
However, I've triple checked my code with the video and a working app from a forum member- still unsure why its causing this error.
If it helps, my getTemperature line is:
public int getTemperature() { return (int)Math.round(mTemperature); }

Ben Deitch
Treehouse TeacherHave you called ButterKnife.bind(this);
in the onCreate method?

Aldrin Cabuniag
3,783 PointsYes:
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); ButterKnife.bind(this);
If you think it would be more helpful I should just post all of the code- don't hesitate to ask :)

Ben Deitch
Treehouse TeacherHmm... is your layout actually named 'MainActivity.xml'? You're referencing it as 'activity_main' (R.layout.activity_main --> /res/layout/activity_main.xml).
6 Answers

Petko Atanasov
Courses Plus Student 3,262 PointsOK so it was the Butterknife config. I had to add the second line to gradle. So for all of you guys that spent an hour or 2 here this is the way your gradle should look:
dependencies { compile fileTree(dir: 'libs', include: ['*.jar']) androidTestCompile('com.android.support.test.espresso:espresso-core:2.2.2', { exclude group: 'com.android.support', module: 'support-annotations' }) compile 'com.android.support:appcompat-v7:25.1.1' testCompile 'junit:junit:4.12' compile 'com.squareup.okhttp3:okhttp:3.6.0' compile 'com.jakewharton:butterknife:8.5.1' annotationProcessor 'com.jakewharton:butterknife-compiler:8.5.1' }

Petko Atanasov
Courses Plus Student 3,262 PointsThank you David!
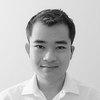
David Tran
Courses Plus Student 12,429 PointsWoohoo! I figured ButterKnife was the problem in later courses. I had to do things the long way in this course.
ButterKnife is very useful, I'm sure you'll enjoy using it!

Denton Zhou
11,775 PointsThanks Petko, that makes sense. I noticed the annotationProcessor line in the docs, but left it out since I wanted to follow the video to a tee.
Docs here: http://jakewharton.github.io/butterknife/
For anyone else running into this issue, copy and paste this into your build.gradle config (fresh as of 5/30/2017):
compile 'com.jakewharton:butterknife:8.6.0'
annotationProcessor 'com.jakewharton:butterknife-compiler:8.6.0'

Aldrin Cabuniag
3,783 PointsI've re-arranged some code and completely took out the @BindView function which worked for me: Still unsure why the original method was faulty for me :|. Thanks for your input though! Ben Deitch
TextView mTimeLabel;
TextView mTemperatureLabel;
TextView mHumidityValue;
TextView mPrecipValue;
TextView mSummaryLabel;
ImageView mIconImageView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ButterKnife.bind(this);
mTimeLabel = (TextView) findViewById(R.id.timeLabel);
mTemperatureLabel = (TextView) findViewById((R.id.temperatureLabel));
mHumidityValue = (TextView) findViewById(R.id.humidityValue);
mSummaryLabel = (TextView) findViewById(R.id.summaryLabel);
mPrecipValue = (TextView) findViewById(R.id.precipValue);
mIconImageView = (ImageView) findViewById(R.id.iconImageView);

Aldrin Cabuniag
3,783 PointsUnder "layout" directory, it is named exactly:
"activity_main.xml"
I've tried Refactor->Rename to "MainActivity.xml", however it says I can only have lowercases as the name. I've reverted it to "activity_main.xml" again.
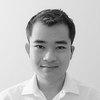
David Tran
Courses Plus Student 12,429 PointsFour months later, I am experiencing the same problem with Aldrin Cabuniag. Getting rid of the @BindView function and replacing it with the original method works for me too.
It could probably be Android Studio version problem? I am using 2.2.3.

Petko Atanasov
Courses Plus Student 3,262 PointsHey guys I got the same FATAL EXCEPTION: main. I added a few lines of code and it appears that JSON is not returning data. Here is the code:
private CurrentWeather getCurrentWeather(String jsonData) throws JSONException { JSONObject forecast = new JSONObject(jsonData); String timezone = forecast.getString("timezone"); double rightTemperature = forecast.getDouble("temperature"); Log.i(TAG, "From JSON: " + timezone); Log.i(TAG, "From JSON: " + rightTemperature);
JSONObject currently = forecast.getJSONObject("currently");
CurrentWeather currentWeather = new CurrentWeather();
currentWeather.setHumidity(currently.getDouble("humidity"));
currentWeather.setTime(currently.getLong("time"));
currentWeather.setIcon(currently.getString("icon"));
currentWeather.setPrecipChance(currently.getDouble("precipProbability"));
currentWeather.setSummary(currently.getString("summary"));
currentWeather.setTemperature(currently.getDouble("temperature"));
currentWeather.setTimeZone(timezone);
Log.d(TAG, currentWeather.getFormattedTime());
return currentWeather;
}
and here is the new error message I'm getting:
E/MainActivity: Exception caught: org.json.JSONException: No value for temperature at org.json.JSONObject.get(JSONObject.java:389) at org.json.JSONObject.getDouble(JSONObject.java:444) at com.example.koko.stormy.MainActivity.getCurrentWeather(MainActivity.java:106) at com.example.koko.stormy.MainActivity.access$100(MainActivity.java:27) at com.example.koko.stormy.MainActivity$1.onResponse(MainActivity.java:71) at okhttp3.RealCall$AsyncCall.execute(RealCall.java:135) at okhttp3.internal.NamedRunnable.run(NamedRunnable.java:32) at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1133) at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:607) at java.lang.Thread.run(Thread.java:761)
Any ideas why this is happening? Maybe that is why a lot of people are getting NULL POINTER EXCEPTION.
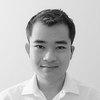
David Tran
Courses Plus Student 12,429 PointsAre you using ButterKnife as per illustrated in the videos?

Petko Atanasov
Courses Plus Student 3,262 PointsAs far as I can see yes. This method returns values from JSON. The second I added this 2 lines
double rightTemperature = forecast.getDouble("temperature"); Log.i(TAG, "From JSON: " + rightTemperature);
I get the new error message and not the NULL POINTER. I do get the timezone tho.
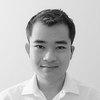
David Tran
Courses Plus Student 12,429 PointsI think the reason why I got this NULL POINTER EXCEPTION is because of ButterKnife. You might too?
The video shows adding one line into Gradle. But the newest ButterKnife (if you are using the latest version) requires two lines:
compile 'com.jakewharton:butterknife:8.5.1'
annotationProcessor 'com.jakewharton:butterknife-compiler:8.5.1'
Try that to see if it works? If it doesn't, don't use ButterKnife and do it the long way to see if that error still persists.

Yusuf Mohamed
2,631 PointsI don't know what those magical lines of code were but you saved my life. Could you explain them a bit to me?
Ben Deitch
Treehouse TeacherBen Deitch
Treehouse TeacherLooks like one of mTemperatureLabel, mTimeLabel, mHumidityValue, mPrecipValue, mSummaryLabel is null. It'll be whichever one is on line 104.