Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial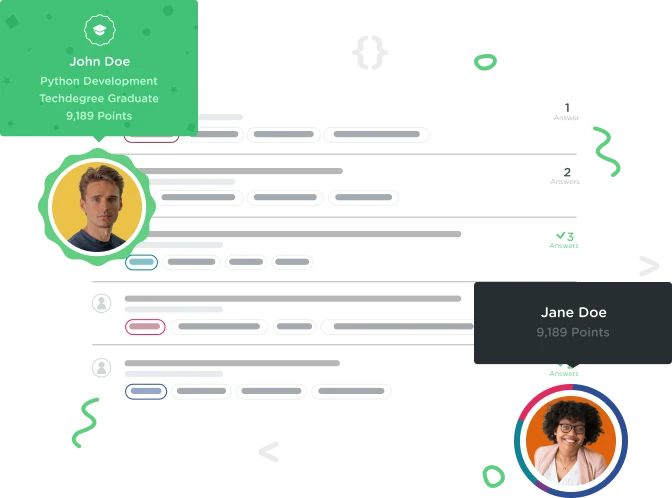

Andrew Mlamba
15,642 PointsObject Oriented Programming
Kenneth Love I have this problem that i need some help with. I can't get the correct balance after withdrawal.
In the SavingsAccount class, implement the withdraw method that takes in the cash withdrawal amount, deducts this amount from the current balance and returns the balance. This method should NEVER allow the balance to get below 500. (Check for this condition and output "Cannot withdraw beyond the minimum account balance" if it happens). Also, output "Cannot withdraw beyond the current account balance" if withdrawal amount is greater than current balance. For a negative withdrawal amount, return "Invalid withdraw amount".
class BankAccount:
def withdraw(self):
pass
def deposit(self):
pass
class SavingsAccount(BankAccount):
def __init__(self):
self.balance = 500
def deposit(self, cash):
if cash > 0:
self.balance += cash
return self.balance
else:
return 'Invalid deposit amount'
def withdraw(self, cash):
if cash > 0:
if cash > self.balance:
return 'Cannot withdraw beyond the current account balance'
elif (self.balance - cash) < self.balance:
return 'Cannot withdraw beyond the minimum account balance'
else:
self.balance -= cash
return self.balance
else:
return 'Invalid withdraw amount'
1 Answer
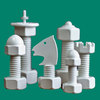
Steven Parker
229,744 Points
Your formula may need some adjustment
Looking at these lines:
elif (self.balance - cash) < self.balance:
return 'Cannot withdraw beyond the minimum account balance'
You are testing if the balance after withdrawal is smaller than the starting balance, which it certainly must always be. You probably meant to check if the balance after withdrawal is smaller than the minimum amount instead.