Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial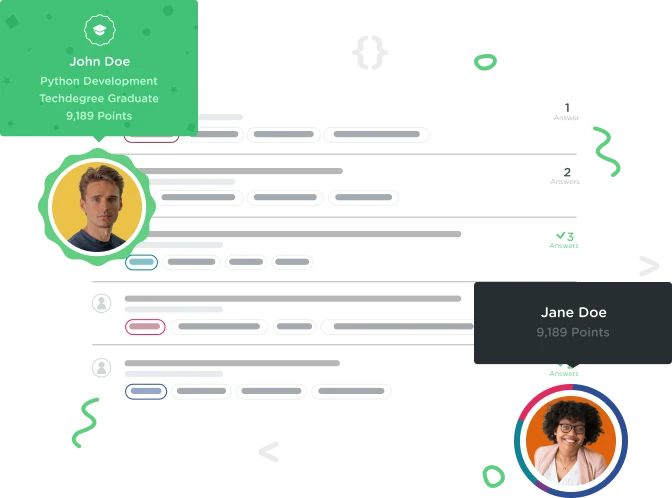

Troy Douglas
1,069 PointsObtaining random number within the sum total of list called in def random_member function. Imports, Challenge 3
I'm attempting to build upon earlier function challenges by calling a list through a function argument, then adding the total of list items through a for loop; however, I need to return a random number from that list using the random.randint() method.
I'm not sure how to wrap my head around this one yet - I've been wracking my brain all day.
import random
def random_member(list1):
length = 0
for item in list1:
length += item
return random.randint(0, length)
6 Answers
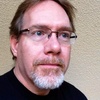
Chris Freeman
Treehouse Moderator 68,457 PointsI reran the challenge and did not see the part regarding a "sum total". Perhaps you are mixing your challenges?
I've split the answer into two sections. This first part solves Task 1 and 2:
# Task 1: Import the 'random' library
import random
# Task 2: Now, make a function named 'random_member' that accepts a list
# as an argument.
def random_member(lst):
# For now, have it return the length of the list.
return len(lst)
This should solve Task 3 (but the challenge seems broken):
import random
def random_member(lst):
# Task 3: Now, make 'random_member' pick a random number between 0
# and the length of the list, minus 1. So if the length was 5, get
# a number between 0 and 5.
index = random.randint(0, len(lst) - 1)
# Return the list item with that index.
return lst[index]
Seeking help from Kenneth Love
Kenneth, when I paste my solution above for Tasks 1 and 2, it also passes Task 3 unaltered. Though I could swear that my solution to Task three above did pass at one time. Is the Challenge broken?
[Edit formatting -cf]

Troy Douglas
1,069 PointsSorry, I worded it rather confusingly - I was over-complicating it by trying to build the list, sum the entries with the for loop, then return the total, etc. I was thinking back to the prior challenges in the Functions segment.
However, when I directly copy/paste your code into my challenge, it tells me that Task 2 is no longer working. I think this is what has caused me to go back and forth and in circles - I've tried numerous simple variations of essentially the same code as what you demonstrated and it keeps cycling back and forth between these odd errors.
This may be an issue with the tutorial/tester itself? Heck, I've tried answers that Kenneth Love has responded to/with and received errors. I understand the concept here (after having posted the original mess, that is) but can't get them to work.
Bummer indeed!
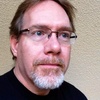
Chris Freeman
Treehouse Moderator 68,457 PointsThe code I posted won't pass all three tasks of the challenge because task 2 and 3 conflict by changing what is to be returned. I my code, I've commented out the solution for task 2. To pass this through the challenge,, uncomment the return
from task 2, and comment the return
for task 3. Once through task 2, reverse the commenting.
Cut an paste can be an issue due to issues with indentation that doesn't always survive the paste, and with the difference between Python 2.x and 3.x, and lastly, as in this challenge, one code solution won't get through all tasks without have to do some edits.
Keep up the good work! It gets easier as you gain more experience looking at code. In not too long, these issues will become much easier to spot.

Troy Douglas
1,069 PointsUsing your code:
import random
def random_member(lst):
index = random.randint(0, len(lst) - 1)
return lst(index)
This is what I'm running; I marked out the elements of task 2 (I also did in my first try at using your code) and it is still giving me the task 2 error. I believe I truly understand the principles here: we're defining the function, then calling a list with it. Then, we are assigning the a random number to the index variable, constrained by 0 and the length of the "lst" list called by the function itself, minus one. Then, we return the function with the index we just obtained the random number with as its argument.
If I am failing to understand that, please let me know. It is late and perhaps my brain is just not picking up on something here. Sorry for being such a pest on this seemingly rather direct challenge! Thank you so much for your efforts and patience thus far - you've helped me on other problems in the past as well!
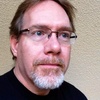
Chris Freeman
Treehouse Moderator 68,457 PointsI did have a type in my code. I've corrected my answer for Task 3:
return lst[index] #<--fix typo. Use [] instead of ()
That said, I've run the challenge 3 times. Two times it would not pass Task 3 unless I used the Task 2 solution. One time it did pass. Non-deterministic behavior is crazy making!
I've tagged Kenneth Love to see if he can verify the insanity.

Troy Douglas
1,069 PointsI just wanted to let you know that it seemed to be working briefly, but having re-visited this, it no longer works either when I type it out myself, or having copy-pasted it (with careful attention to indentation).
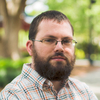
Kenneth Love
Treehouse Guest TeacherI just tried the challenge and passed it. Can you share the code that failed?
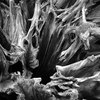
circlewave
8,232 Pointsdeleted, will ask in new thread
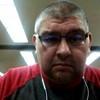
micram1001
33,833 PointsThe answer to challenge #3
// challenge #1 import random
import random;
// challenge #2 define random member with list pass and returned list
def random_member(list):
// return len(list);
// challenge #3 return the index of list with random number between (0, 4) and length of 5 minus one
index = random.randint(0, len(list) -1);
return list[index];
Kenneth Love
Treehouse Guest TeacherKenneth Love
Treehouse Guest TeacherYep, had a typo in my validator. Should be fixed now.
Chris Freeman
Treehouse Moderator 68,457 PointsChris Freeman
Treehouse Moderator 68,457 PointsThanks Kenneth! +1 Bug Bounty!