Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial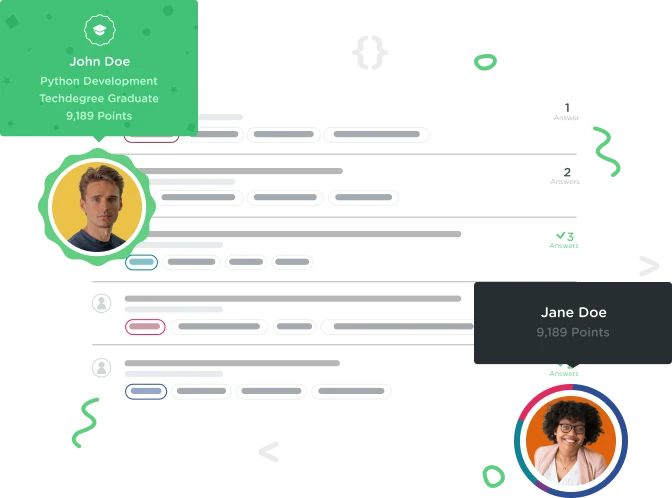
Chris Grazioli
31,225 PointsOverride the __len__ method so that it always returns the wrong number of items in the list. For example, if a list has
Kenneth Love I know I'm missing something trivial here... what is it? Your overriding the len to be incorrect, but the Challenge suggests using super(), which to me, means using the correct len for list and then changing it
class Liar(list):
def __len__(self, items):
real_length= super().__len__(items)
return real_length+=3
5 Answers

Kent ร svang
18,823 PointsHere's how I usually implement this type of code:
class Liar(list):
def __len__(self):
return super(Liar, self).__len__() + 2
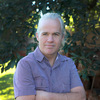
Christopher Shaw
Python Web Development Techdegree Graduate 58,248 PointsJust two things to look at:
len is the length of the list, you don't provide the items, so:
def __len__(self):
# and
real_length= super().__len__()
Lastly, remove the = from your return statement, you are not setting the value of real_length, just returning a value. return real_length + 3

tmsmth
10,543 Points
Katsiaryna Krauchanka
Full Stack JavaScript Techdegree Graduate 22,154 PointsBecause super().__len__(self)
is wrong syntax.
__len__()
does not take any arguments.
The following code works
class Liar(list):
def __len__(self):
return super().__len__() + 1
You can refer to the documentation https://docs.python.org/3/library/functions.html#super
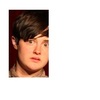
Ikuyasu Usui
36 PointsCan you explain why this doesn't work?
class Liar(list):
def __init__(self, *args, **kwargs):
super().__init__()
def __len__(self):
return self.__len__()
I get "RecursionError: maximum recursion depth exceeded" which is all right, but I don't know why it doesn't work. After super().__init__()
, self should be simply a list.

ryan forte
2,691 PointsIn Python3 we do not need to add the SubClass and instance when we call super. Nor do we need the dunder init, as Python3 will interpret it as a List. So simply we can call it like this:
class Liar(list):
def __len__(self):
return super().__len__() + 5
Christopher Carucci
1,971 PointsChristopher Carucci
1,971 PointsWish you would comment your code.
Graham Usai
16,688 PointsGraham Usai
16,688 Pointsthis worked