Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial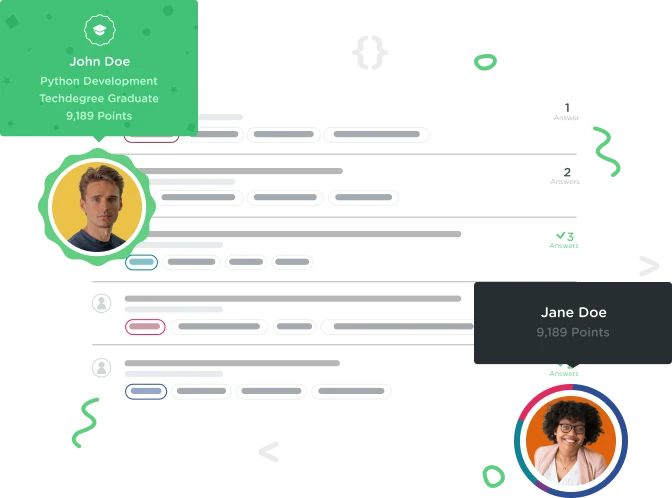
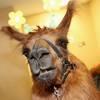
A X
12,842 PointsPassed into a Function
Aisha's video on Callback Functions confused me as I don't know what the phrase "passed into a function" means. Does it mean that the callback function stores data for later use using the return...method?
I also wanted to clarify the differences between a function, a method, a class, and an object as I'm starting to confuse these terms.
Thanks!
3 Answers
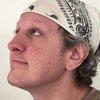
Dennis Brown
28,742 PointsOk! Let's get this one 100% answered, because this is something extremely important in JavaScript, especially when working with Asynchronous JavaScript.
A callback function is a function in which you are asking for a continuation of the function once it's done, so that you may do even more. Since this is a jQuery video, let's look at jQuery's getJSON method, a method commonly used with a callback:
$.getJSON('https://someawesome/api/?callback=?', function(data) {
// getJSON has sent a request to an API, and is now waiting for us to do awesome
// things with the "data" that came back in our callback function here
console.log(data); // to start, we'll just look at the json object ;)
});
Without a callback, we're just requesting data, then receiving it without doing anything else (the method would stop after the API URL). Of course may API servers will refuse connections without a callback, because you always want to do something with that data, and having a function waiting for the response is a great way to do so.
As you can see we have passed a function into the function being called. This is so that when data is received, the callback function makes use of the data that has been returned. This is the same thing that happens when we write events in jQuery. At the end we have a callback function to be run as soon as that event is triggered. Pretty cool. :)
$('#someid').on('click', function() {
$('#div').text('clicked!');
});
There really isn't much more to it. I could show you many different patterns of callbacks to make it look more involved, but all in all it's the same thing except under different circumstances.
I also wanted to clarify the differences between a function, a method, a class, and an object as I'm starting to confuse these terms.
No problem!
First off functions are objects with a function declared. That means anything that does something, and returns a result. We use functions to do exactly that; to do something with the data we have to create another result.
A method is a specific function available from another function, or object type (simplified version). For instance the various jQuery functions used like .append, .show, hide, .on, and everything else are considered jQuery methods. The same can be said for array methods which are only available when you are working directly with an array object like reduce, shift, pop, push, or a string method when working with a string like slice, split, concat, and filter.
I wouldn't worry too much about classes right now, because since JavaScript is NOT a statically typed language, it's not the type of class you would be use to in other languages, even though ES6 uses them the same way. Once you start to learn about using prototypes, you'll learn all about making prototypal classes for JavaScript, and even create your own methods. Just understand that it has everything to do with working with and instantiating the prototypes of an object. :)
Lastly let's talk about objects. You may have noticed up until now I've talked about a lot of objects, and that's because JavaScript considers everything to be an object. Of course the type of object you are probably confusing the most is an Object literal, the last and most common JavaScript object type. The most common is an Object Literal, or even a JSON (JavaScript Object Notation) object (we grabbed one with getJSON earlier).
var awesomeObject = {
name: 'awesome object',
type: 'just an object',
array: ['I', 'can', 'hold', 'arrays'],
exists: true,
total: 10
}
So that's an object literal, showing the power of an object, and how it can hold all of that data in a single object named awesomeObject. Each of the properties inside can be called like an array as well by reference the key (the name of the property). e.g. awesomeObject.name, awesomeObject[exists], awesomeObject.array[0] .
I hope that helps!
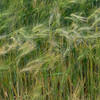
Christof Baumgartner
20,864 PointsHi,
I haven't seen the video, but I understand as passed into a function, that the function gets parameters. This parameters can be existing variables. E.g.:
var parameter1 = 4;
var parameter2 = 5;
function(parameter1, parameter2){
console.log("you have entered " + parameter1 + " and " + parameter2 "into this function");
};
Before I try to explain what all this terms are, I would like to share a few resources on this topic. This question has asked quite often, because every one who starts programming has to learn this terms: codacedemy forum stackoverflow other treehouse thread This should answer most questions.
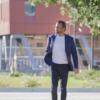
Bappy Golder
13,449 PointsHere is how I understand call back function in simple terms:
//how I understand call back functions
/*call back functions are functions that are called/executed when something else happens (or some event/s takes place). The primary difference between a call back function and a normal function:
- normal functions are first declared and then called in the JavaScript code later on.
- a call back function is never called/written to execute later in the code. Rather they excuted based on an event that happens later. Due to this a call back function often have the following two parameters
1. At what event the call back function will be run (or the event)
2. What will be done or the function that will be run*/
Hope that helps.