Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial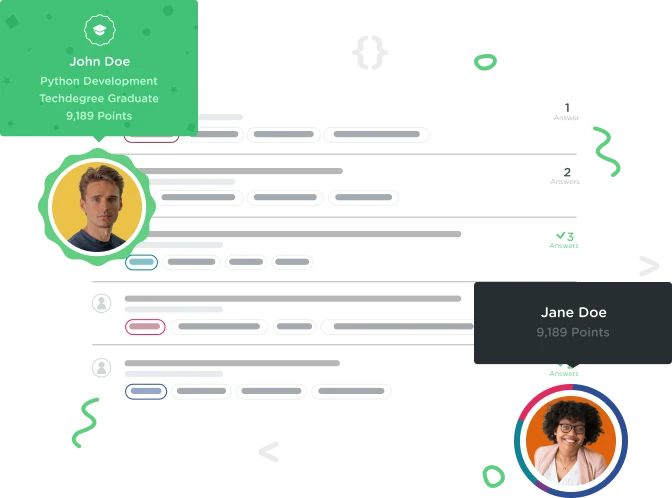
Evan Huddleson
12,623 Points"Pop" part 1 failing
Should I be running this as a function?
I'm a bit stumped but feel like the solution will be painfully simple...
messy_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Your code goes below here
messy_list.pop(3) = item
messy_list.insert(0, item)
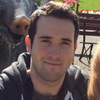
Justin Iezzi
18,199 PointsAdam,
That might pass the compiler check, but it's not the best idea to hard code the element as you did. In a real program, these elements may be different each time the program or function is ran. If not, if you ever change that element you'll now need to change it in two different places.
A more programmatic way to approach the one line solution would be to write it like this
messy_list.insert(0, messy_list.pop(3))
The pop method returns the element, making a one line solution really easy. That way we don't assume what the element is, it'll just work regardless of what data is inside that element.
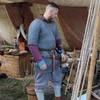
Adam Ryde
5,222 PointsHi Justin, i am confused, i am new to programming i will be the first to say that, haha. the only difference i see is that my code spanned two separate lines, and you managed to put the same code into one line. (which fair play i couldn't quite work out how to do that.)
but the confusing part to me is that you say its not best to hard code? what is hard code? and that in a real program, how would these elements be different if my code does the same as your code? surely if they are the same they will do exactly the same thing inside a real program?
sorry if this sounded bullshy, that isnt my intent at all, i am just eager to learn where i went wrong and how to better understand what you mean.
many thanks
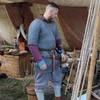
Adam Ryde
5,222 PointsEDIT - i just saw what you wrote beneath the code, i see what you mean. in my way i was inserting 1 at index 0. where as in your way it will pop and then insert what ever is at index 3 to index 0 no matter what it may be, weather its a list, dict, int, etc.
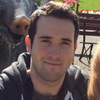
Justin Iezzi
18,199 PointsNo worries at all, you're asking the right questions! There are several different concepts at work here, so I'll break it down.
Hard coding is when you write code that isn't dynamic. Say that in your program a user can create the list instead. The user may not choose 1 as the third element, but with what you wrote -
messy_list.pop(3)
messy_list.insert(0, 1)
No matter what the user writes for that third element, a value of 1 will always replace it. What we really want is for the specified element to be transferred to the first position of the list, no matter what value is inside that element. This is where the power of programming comes into use. Even though both of our codes will work the same, since our lists are the same, we should always think ahead and prepare for content to be different than we anticipate. This will allow us to extend the program without having to go back and change that number in several different locations, reducing headaches, bugs, and errors.
How does this work?
.pop()
is a method that removes an element from a specified location in a list.
If we simply run
messy_list.pop(3)
the third element will be removed. The element is then lost, since we didn't assign it to anything. That's okay if we don't want to use this element anymore.
However, since we do want to use this specific element again, we can take advantage of the .pop()
method's return value. Some methods have return values, some don't. Because .pop()
does return a value, we can assign it to a variable as Evan did.
item = messy_list.pop(3)
An easy way to think about it is knowing that since .pop()
returns a value, you can think of the entire messy_list.pop(3)
as the value it returns.
item = messy_list.pop(3)
# item = 1;
My # comment is how the compiler will read it, since 1 will be returned from .pop(3)
. Knowing this, we can place messy_list.pop(3)
anywhere we want knowing that it will return that element's value.
So
messy_list.insert(0, messy_list.pop(3))
# messy_list.insert(0, 1)
If we change the elements value in the list, it will work accordingly. If we specify a different element to pop, it will still work accordingly.
Hopefully this makes sense! Don't be afraid to ask more if you don't quite understand.
5 Answers
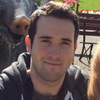
Justin Iezzi
18,199 PointsYou have the right idea, but your code is organized incorrectly.
Since you're trying to assign the pop element to item
, the correct syntax would be
item = messy_list.pop(3)
Evan Huddleson
12,623 PointsThanks for help everyone : )
lol, now running into issues getting this thing to loop through properly and remove the specified items.
Tried running my own examples and they were failing, went to the forums to see if anyone had solved it.
One fella had this as his code...
for item in messy_list: if not isinstance(item, int): messy_list.remove(item)
I read up a bit on the isinstance func, not sure what the issue is here...halp?
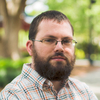
Kenneth Love
Treehouse Guest Teacherisinstance()
tells you whether or not a variable is an instance of a particular class (for now, just think "type", like str
or int
).
That won't actually work for one value in this, though, because I was being a bit tricky :D
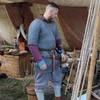
Adam Ryde
5,222 Points@Justin Iezzi, thank you very much mate, i when i was typing my reply i saw what you wrote underneath the piece of code you put in. after reading through a few times i understood what you were getting at. but you hit the nail on the head with the in depth explanation :) thank you very much it has cleared it up for me. It has also reiterated the importance of thinking ahead (which will undoubtedly make me a better programmer in the long run) to stop bug occurring and like you said, the head ache (or ball ache, which ever way you see it) of having to change lines or blocks of code that could be 100 lines previous,
much appreciated my friend,
thanks again
Evan Huddleson
12,623 PointsKenneth Love Tricky indeed my friend!
I've been running through this for loop in the workspace...
for item in messy_list:
if item == "a":
messy_list.remove(item)
elif item == False:
messy_list.remove(item)
elif item == messy_list.index(3):
messy_list.remove(item)
print(messy_list)
However I don't get a print excluding the last array item...originally in messy_list.index(5)...my last printed statement is --->>> [1, 2, 3, [1, 2, 3]]
Thoughts?
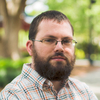
Kenneth Love
Treehouse Guest TeacherWhy do it as a loop? Just remove the items you need to remove. You're making it harder than it needs to be :D
Not to mention, changing an iterable while looping through it makes your loop not work exactly like you think it will. Best to avoid doing that.
Evan Huddleson
12,623 PointsGot it, definitely was overcomplicating it. Thanks Kenneth : )
messy_list = ["a", 2, 3, 1, False, [1, 2, 3]]
messy_list.insert(0, messy_list.pop(3)) messy_list.remove("a") messy_list.remove(False) messy_list.remove([1, 2, 3]) print(messy_list)
Adam Ryde
5,222 PointsAdam Ryde
5,222 Pointsaup mate, first of all the variable you are trying to make, ITEM, will fail. to assign a variable it needs to be on the left on the = sign.
item = messy_list.pop(3) would assign index 3 of the list to the variable item.
secondly, i know it says try to do it in once step, i didnt but thats just me.
i would just pop the 1 off at index 3, without assigning it to a variable. and then just insert 1 back at index 0 using insert.
messy_list.pop(3)
messy_list.insert(0, 1)