Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial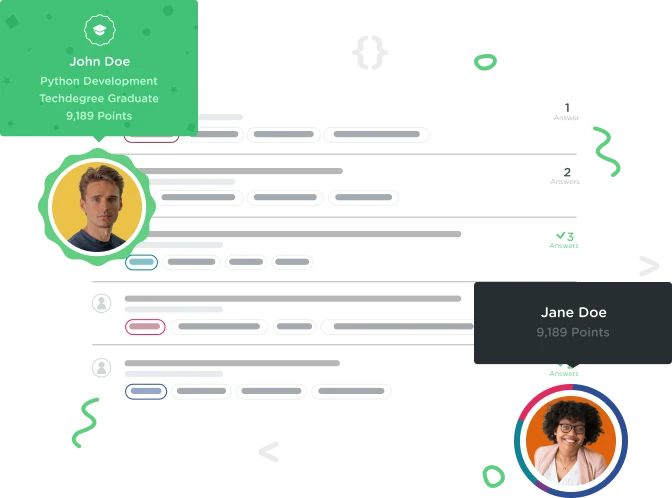
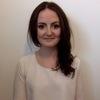
Zuzanna Kulej
1,592 PointsPreview doesn't work.... And I can't find where is a problem
.
package com.example;
import java.util.Date;
public class BlogPost {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
import com.example.BlogPost;
public class TypeCastChecker {
/***************
I have provided 2 hints for this challenge.
Change `false` to `true` in one line below, then click the "Check work" button to see the hint.
NOTE: You must set all the hints to false to complete the exercise.
****************/
public static boolean HINT_1_ENABLED = false;
public static boolean HINT_2_ENABLED = false;
public static String getTitleFromObject(Object obj) {
String result = "";
if (obj instanceof String) {
result = (String) obj;
}
return result;
}
public static String getTitleFromBlogPost(BlogPost obj) {
String answer = "";
if (obj instanceof BlogPost) {
answer = ((BlogPost) obj).getTitle();
}
return answer;
}
}
1 Answer

Yanuar Prakoso
15,196 PointsHi There Zuzanna Kulej
The main problem in your code is you do not have to make separate method to process the obj If the obj is indeed instanceof BlogPost. All you have to do to fix and pass your challenge is to move your last code into previous method. Here I show you what the code should be like:
import com.example.BlogPost;
public class TypeCastChecker {
/***************
I have provided 2 hints for this challenge.
Change `false` to `true` in one line below, then click the "Check work" button to see the hint.
NOTE: You must set all the hints to false to complete the exercise.
****************/
public static boolean HINT_1_ENABLED = false;
public static boolean HINT_2_ENABLED = false;
public static String getTitleFromObject(Object obj) {
String result = "";
if (obj instanceof String) {
result = (String) obj;
}
//you can just move the if statement from the getTitleMethod here because it will also return a String right?
//Just use result variable to get the Title you do not have to make another new method just for this:
if (obj instanceof BlogPost) {
result = ((BlogPost) obj).getTitle();//<- since BlogPost.getTitle() method will also return a String you can just put it to result variable
}
return result;
}
/* So now if the obj passed to the getTitleFromObject(Object obj) is infact a String then the result will be the obj itself
* but if the obj passed is indeed a BlogPost type object you will use the method obj.getTitle() to obtain the title which
* definitely a String to passed on to result variable. Of course after you casted the BlogPost type to obj first.*/
/*You do not need this method:
public static String getTitleFromBlogPost(BlogPost obj) {
String answer = "";
if (obj instanceof BlogPost) {
answer = ((BlogPost) obj).getTitle();
}
return answer;
}
--------------------------------------------*/
}
I hope this can help a little. Good job passing the first task of the challenge. Your code is already clean just need a little reparation for the 2nd task but the objective of your code and the implementation is already good. Keep up the good work and happy coding.
Bogdan Siverchuk
Courses Plus Student 1,707 PointsBogdan Siverchuk
Courses Plus Student 1,707 PointsHi there,
Use instanceof to see if the obj is a String. If it is, cast it to String and return that.
Start with the if. The condition is the instanceof expression. Inside the if return the cast obj.
if (obj unstanceof String){ } return(String)obj; I had the same problem and nice guy Steve told me to do like this and it worked or just check this https://teamtreehouse.com/community/java-data-structures-challenge-1-of-2