Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial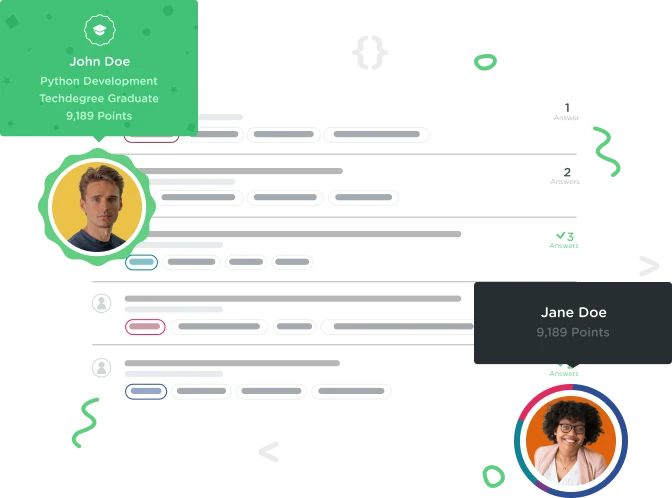
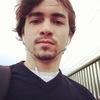
Leonardo Motta
11,284 PointsProblem with my logic lotery numbers.
I need to return an array with 6 random numbers. They need to be from 1 to 60 and all the 6 numbers have to be unique.
this current version just crashes.
function getLoteryGame() {
var loteryGame = [];
var usedNumbers = [];
var randomNumber;
for (var i = 0; i < 6; i++) {
randomNumber = Math.floor(Math.random() * 60 + 1);
usedNumbers[i] = randomNumber;
if(usedNumbers.includes(randomNumber)) {
i--;
} else {
loteryGame[i] = randomNumber;
}
}
return loteryGame;
}
console.log(getLoteryGame());
4 Answers
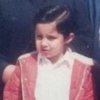
Elijah Quesada
Front End Web Development Techdegree Graduate 32,965 PointsThis article helped me figure out the sort method for arrays.
https://teamtreehouse.com/community/javascript-sort-method-how-does-the-comparison-actually-work
function getLoteryGame() {
var loteryGame = [];
var usedNumbers = [];
var randomNumber;
for (var i = 0; i < 6; i++) {
randomNumber = Math.floor(Math.random() * 60 + 1);
if(usedNumbers.includes(randomNumber)) {
i--;
} else {
loteryGame[i] = randomNumber;
usedNumbers[i] = randomNumber;
}
}
loteryGame = loteryGame.sort( function(a,b) {
return a - b;
});
return loteryGame;
}
console.log(getLoteryGame());
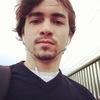
Leonardo Motta
11,284 PointsI got stuck in a infinit loop, that was the problem.
here is the solution:
function getLoteryGame() {
var loteryGame = [];
var usedNumbers = [];
var randomNumber;
for (var i = 0; i < 6; i++) {
randomNumber = Math.floor(Math.random() * 60 + 1);
if(usedNumbers.includes(randomNumber)) {
i--;
} else {
loteryGame[i] = randomNumber;
usedNumbers[i] = randomNumber;
}
}
return loteryGame;
}
console.log(getLoteryGame());
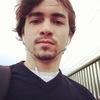
Leonardo Motta
11,284 PointsNow that it does what I want how to sort it from the lower number to the highest [1, 2, 3, 4, 5, 6]
return loteryGame.sort(); is not doing it.
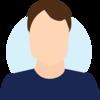
Mike Hatch
14,940 PointsUsing Elijah's workable code you would change 60
to 6
if you want the output to be [1, 2, 3, 4, 5, 6]
.
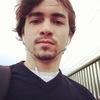
Leonardo Motta
11,284 PointsThe final version of my function.
function getLoteryGame() {
var listToPlay = [];
var usedNumbers = [];
var loteryGame;
var randomNumber;
for (var i = 0; i < 6; i++) {
randomNumber = Math.floor(Math.random() * 60 + 1);
if (usedNumbers.includes(randomNumber)) {
i--;
} else {
listToPlay[i] = randomNumber;
usedNumbers[i] = randomNumber;
}
}
listToPlay = listToPlay.sort(function(a, b) {
return a - b;
});
return (loteryGame = listToPlay.join(" "));
}
console.log(getLoteryGame());
Leonardo Motta
11,284 PointsLeonardo Motta
11,284 PointsHow would it be without arrow function? I couldn't understand that, sorry
Elijah Quesada
Front End Web Development Techdegree Graduate 32,965 PointsElijah Quesada
Front End Web Development Techdegree Graduate 32,965 PointsNice job though, on figuring out the duplicate issue.
Leonardo Motta
11,284 PointsLeonardo Motta
11,284 PointsThanks!
Leonardo Motta
11,284 PointsLeonardo Motta
11,284 PointsSo we can make a function inside another is that it?
Elijah Quesada
Front End Web Development Techdegree Graduate 32,965 PointsElijah Quesada
Front End Web Development Techdegree Graduate 32,965 PointsYes, because functions are treated as first class citizens. You can pass them into other functions.
https://teamtreehouse.com/library/callback-functions-in-javascript
Leonardo Motta
11,284 PointsLeonardo Motta
11,284 PointsThanks for the help, was a bit trick because I didn't reach this level yet, But I got this!
Elijah Quesada
Front End Web Development Techdegree Graduate 32,965 PointsElijah Quesada
Front End Web Development Techdegree Graduate 32,965 PointsNo problem. Happy coding!!