Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial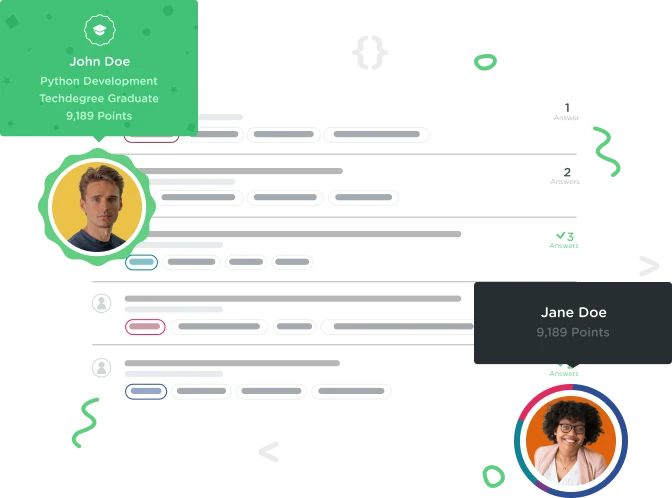
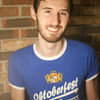
Trent Burkenpas
22,388 PointsPython 2 or Python 3 ?
So I'm still pretty new to python, but I was wondering which python makes more sense to use. I have heard that python 3 has fixed some issues with 2, and made it faster. But more people use python 2. So what Python is better to use in your opinion, python 2 or 3?
thanks !
8 Answers
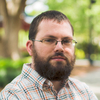
Kenneth Love
Treehouse Guest TeacherWelcome to the can of worms. :)
So, here's the state of things at the moment:
Python 2 is super old and has been officially declared to have an end-of-life which is Python 2.7. There will never be a Python 2.8. (at least, according to the BDFL). So you shouldn't use Python 2.x.
But, most libraries out there are Python 2.x compatible (we'll say that x
doesn't go any lower than 5, preferably 6). So you should use Python 2.x.
Python 3.x is the future of Python. That isn't going to change. If you want to get the latest and greatest of Python (yield from
, asyncio
, etc), you should be using Python 3.x. It's generally faster, generally has fewer bugs, and is more consistent as a language. You should use Python 3.x.
But there are several fairly important libraries that don't yet support Python 3. Now, what's important changes depending on what you're doing. Don't upload to S3? Then you don't care that boto
doesn't support Python 3. Not doing any natural language processing? Then nltk
's lack of Python 3 support isn't a big deal. So you might not want to use Python 3.
All of that said, I'll be teaching Python 3, I don't plan on doing any personal work that isn't in Python 3, and every package I release currently does and in the future will support Python 3 (or, rather, it'll support Python 2 while being written in Python 3).
You don't really have to make this choice in your own code. You can write Python 2 code that'll work perfectly in Python 3 and vice versa. You can also write Python 2 code that'll port cleanly to Python 3 (and vice versa) if you want to maintain two code bases (you probably don't).
If you're going to listen to what I say, though, use Python 3.
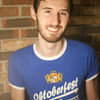
Trent Burkenpas
22,388 PointsWow thanks Kenneth Love,
I was listen to a podcast of two python programmers having a debate on which one was better. Unless i'm mistaken the podcast said that around 90% of python programmers use python 2. I think the podcast is a year old or so, so that might be different now. But why is that, why are so many python programmers not using 3?
Podcast: http://frompythonimportpodcast.com/2014/03/31/episode-017-the-one-about-python-3/
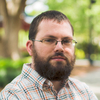
Kenneth Love
Treehouse Guest Teacher- Any link to the podcast? There's a chance it was one I produced :)
- Momentum. People have been using Python 2 for almost 14 years (Python 2.0 came out in October, 2000). They're used to its implementation of things like
print
and integer division and don't have a pressing need to move up to Python 3. Also, Python 3's early versions had some real weirdness with unicode and byte strings that bit a lot of people in the ass. That's all been cleaned up now, though, so it's not really an issue.
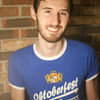
Trent Burkenpas
22,388 Pointsyeah I added the link! Yeah I also heard that when 3 first came out it had a lot of problems.
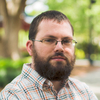
Kenneth Love
Treehouse Guest TeacherAh, yeah, I haven't had anything to do with __fpip__
yet. They're a solid podcast, though.
I think these are the only things that have tripped me up, even a little, between 2 and 3.
Printing
In Python 2:
print "some string"
In Python 3:
print("some string")
But you can do this in Python 2:
from __future__ import print_function
print("some string")
And it'll work. (Those of you that already know a bit of Python will know that print("some string")
would work anyway in Python since parens can be used as a sort of container; this shortcut fails once you want to print more than one thing as then you start printing a tuple and don't get the result that you want, which is why you need the import)
Generators
If you don't know what a generator is, don't worry about details; it's a list that's not stored in live memory and gives you only the next iteration each time you call it.
Python 2:
g = my_generator
g.next()
Python 3:
g = my_generator
next(g)
I don't know of a backwards-compatible hook for this but I haven't looked, either. At the very least, you could catch an AttributeError
and call the other method.
Relative Imports
You can define things locally so that Python doesn't use the global package (or just to have things locally). In Python 2, importing these can be tricky so you bring part of Python 3 in to make it work cleanly.
from __future__ import absolute_import
from stuff import thing
In Python 3 it's just:
from stuff import thing
But the __future__
import will fail silently, I think, so it's a non-issue. Just write it the Python 2 way, just in case.
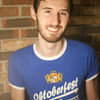
Trent Burkenpas
22,388 PointsThanks for all the info! btw what is the podcast that you worked on?
Thanks Kenneth Love !
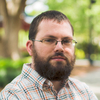
Kenneth Love
Treehouse Guest TeacherI used to do the Forrst Podcast and I did the Django Round-up podcast. Both of which are now dead :)
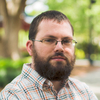
Kenneth Love
Treehouse Guest TeacherOh, I remembered another thing that tripped me up between 2 and 3.
__unicode__
vs __str__
So, in Python, most classes implement a __repr__
method that's used for debugging purposes and gives a bunch of info about the object. If you want a string/unicode representation of the object, you define the __str__
or __unicode__
methods on the object and have it print something (for example, it might print the name of the person that the object represents).
The problem is that Python 3's base object
doesn't have a __unicode__
method, it only has __str__
. Python 2 has both and strings and unicode aren't the same thing (everything is considered unicode in Python 3 unless otherwise specified).
So most of the time you write the __str__
method and then write a __unicode__
method that returns a unicode-ified version of the __str__
output for Python 2. It's a bit messy but :shrug:
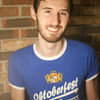
Trent Burkenpas
22,388 PointsKenneth Love lol ":shrug:" that cracked me up. Thanks for the extra info. So python 3 is more convenient when is comes to unicode?
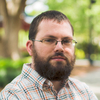
Kenneth Love
Treehouse Guest TeacherTrent Burkenpas yes and no? Unicode is one of the areas that developers never really seem to understand (I'm guilty myself). But all strings in Python 3 are unicode unless otherwise noted.
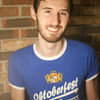
Trent Burkenpas
22,388 PointsOk good to know. Thanks!
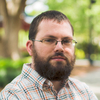
Kenneth Love
Treehouse Guest TeacherSeems like it never ends! Just came across this article today. It's a great comparison between the two.
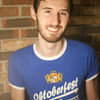
Trent Burkenpas
22,388 PointsKenneth Love Thanks for the article! Speaking of articles do you know any good sources for Python. Like blogs, people to follow on twitter, text books, websites...ect ect.
Thanks!