Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial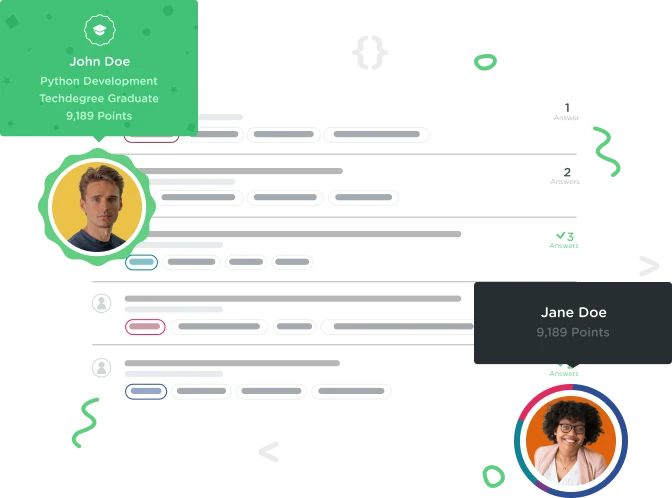

Halcyon Abraham Ramirez
846 PointsPython collections. random choice. challenge task 1
I swear to go is it me or are the code challenges and the video lectures before each challenge are a quantum leap in difficulty?
I mean hey. the random choice lecture was 2 minutes long explaining random choice and now the code challenge wants multiple random choices from an iterable where it was never even mentioned in the lecture how to do so.
am I missing something?
import random
def nchoices(x,y):
lists = []
lists.append(random.choice(x,y))
return lists
8 Answers
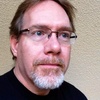
Chris Freeman
Treehouse Moderator 68,423 PointsCreate a function named nchoices() that takes an iterable and an integer. The function should return a list of n random items from the iterable where n is the integer. Duplicates are allowed.
Modifying your code slightly,
Edit: updated code for clarity
import random
def nchoices(iterable, n):
'''Take an iterable and an integer and
return a list of n random items from the
iterable, where n is the integer.
Duplicates are allowed.
'''
# initialize empty list for results
results = []
for index in range(n): #<-- Add loop to run "n" times
# pick random item
pick = random.choice(iterable)
# append pick to results list
results.append(pick) #<-- in each loop, choose random item from "iterable" and append to results list
# after looping complete, return results list
return results
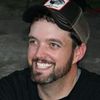
Nick Miller
4,417 PointsI have to somewhat agree with the OP's statement about the code challenges often being a lot more complex than their accompanying videos. I understand that the code challenges are a good opportunity to apply everything we've learned (not just the one video). That being said, some of these challenges can be pretty difficult. The OP is right, we went from a quick video that explained one simple function to a code challenge that required us to apply several advanced (for us) topics.
Speaking of which, when did we learn how to loop through a function n
times? Even if we did learn how to do that, I'm sure we didn't learn how to loop through something n
times where n
is equal to a function's argument.
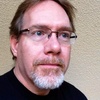
Chris Freeman
Treehouse Moderator 68,423 PointsTagging Kenneth Love for comment.
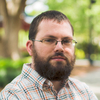
Kenneth Love
Treehouse Guest TeacherWe've gone over for
loops and range()
multiple times both in this course and in Python Basics. A value coming in as an argument is no different than you having it set as a variable. Yeah, the combination of all of these might be new but you're going to run into hundreds of new things as you do programming.
That said, this course is due for an overhaul and will probably get one this summer.

Peter Rzepka
4,118 PointsHalcyon Abraham Ramirez I swear to go is it me or are the code challenges and the video lectures before each challenge are a quantum leap in difficulty?
Posted by Kenneth
"That said, this course is due for an overhaul and will probably get one this summer."
Please!!!! i would appreciate it if you did the quiz questions in the lecture and b r o k e t h e m d o w n doing it in work spaces and then having them do the same thing on the quiz. i wanted to honestly give up after dictionaries. but googled the answers so i can get the badge. but i honestly have been having a hard time knowing what your trying to quiz on cause the questions are so vague...

Emmet Lowry
10,196 PointsHi Chris could u break that down a bit more for me . Im finding it hard to get what its asking thanks
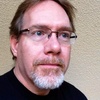
Chris Freeman
Treehouse Moderator 68,423 PointsUpdated answer above. Hope it's clearer.

Emmet Lowry
10,196 PointsThanks for that wondering could u please explain some of the core concepts eg dictionarys and lists a little bit . I an finding python difficult and help on it to make it easier would be fantastic thanks.
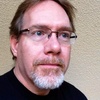
Chris Freeman
Treehouse Moderator 68,423 PointsIt might be best to repost this as a new forum question.

Annie Scott
27,613 Pointsimport random
def nchoices(n,y):
random_items = []
for i in range(y):
random_items.append(random.choice(n))
return random_items

Blake Handson
1,642 PointsThe question is rather ambiguous.It's not actually returning a list of 'n random' items, n is in fact a set value am I not correct? It is then choosing a 'random' item from that particular loop instance based on the passed in 'n' value. So I suppose technically, you are receiving a list of random items, however, the number of times you loop is not based on a random n value which is how I read the question. In saying that I am happy to admit I am completely mis-interpreting what was being asked in the question haha
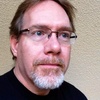
Chris Freeman
Treehouse Moderator 68,423 PointsI would read "n
" as the "number of random values to be chosen"
Let's break down the task description into input
, guts
, outputs
, and misc info
:
- "Create a function named
nchoices()
that takes an iterable and an integer [, sayn
,]...." (inputs) - "The function should return a list of
n
random items..." (output) - "...[the]
n
random items [are chosen] from the iterable, wheren
is the [input] integer." (guts) - "Duplicates are allowed." (misc info)

Alexey Li
8,936 Pointsimport random
def nchoices(itr, int_1):
list_of_n = []
while int_1 > 0:
random_item = random.choice(itr)
list_of_n.append(random_item)
int_1 -= 1
return list_of_n
Carina De Jager
1,779 PointsCarina De Jager
1,779 Pointsmy code is set up just like this one. But it tells me that it returned 1 item instead of 5...? How do you get it to make more than one random selection without having to do multiple lines of the exact same code?
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsCarina, can you post your code?
Carina De Jager
1,779 PointsCarina De Jager
1,779 PointsThis is the set up I currently have.
It says it returns a list of 1 when it expected 5.
[edit: formatting --cf]
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsCarina, After reformatting your code, it looks like your
return
statement is inside thefor
loop which makes the function return at the end of its first iteration. Hence only 1 random item will be returned.Try unindenting the
return
statement to align with thefor
statement.Carina De Jager
1,779 PointsCarina De Jager
1,779 PointsACK!!!! Thank you very much!!! Something so small and simple and I've been going crazy for days trying to fix it... Finding this very frustrating with no coding experience. I really appreciate your help. Thanks again.