Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial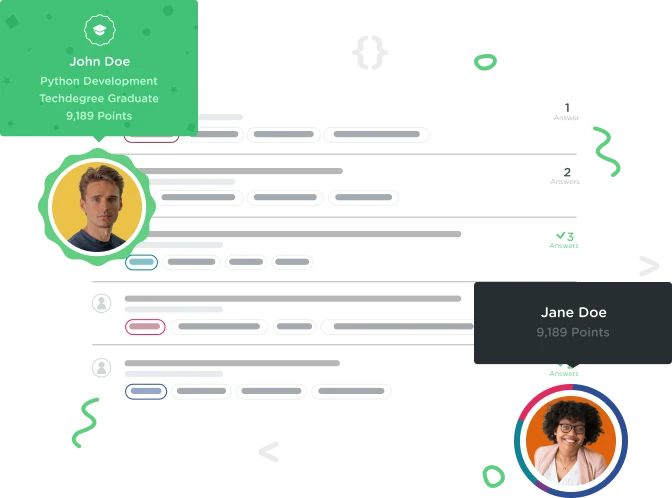

Jeff Quinn
Courses Plus Student 891 PointsPython Collections: Stage 2 - sillycase challenge
Here is the challenge: Create a function named sillycase that takes a string and returns that string with the first half lowercased and the last half uppercased. E.g. 'Treehouse' should become 'treehOUSE'
I have tested my code in the workspace and the only problem is with the round function: instead of returning 'treehOUSE' it returns 'treeHOUSE' and I am not sure how to fix this. (4.5 is being rounded down instead of up?)
def sillycase(str):
return str[:round(len(str) / 2.0)].lower() + str[round(len(str) / 2.0):].upper()
14 Answers

Annie Scott
27,613 Pointsdef sillycase(c):
return c[:round(len(c) / 2)].lower() + c[round(len(c) / 2):].upper()
Honestly unsure about how came to this answer, never seen example shown there, So confused on this python Track but his is the answer

Jason Anello
Courses Plus Student 94,610 Pointsfixed code formatting
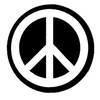
john larson
16,594 PointsAnnie, it took me a minute to see what you did...that's brilliant :D

simon bao
5,522 PointsI did it similar but in more of a crude way
def sillycase(a):
String = a
half = round(len(String)/2)
return a[:half].lower() + a[half:].upper()

Ary de Oliveira
28,298 Pointsseems a bit archaic, I remember when we use MS-DOS C: /, but on days like today, Python 2 Python 3, today we already have REAL TIME UPDATE but run program in two versions, seems a bit archaic ancestor .. . without evolution, I get an email, there have to figure out if it was written in Python 2 to Python 3, archaic .... A version eliminates the other and, only works with the most updated Mr Python2 OR 3 ...
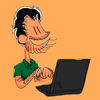
Juan Martin
14,335 PointsHello Jeff, I checked your code and the problem was that the round() function returns 4 instead of 5 (4.5 is actually being rounded down). So the solution for this is adding 1 after the 4.5 is rounded down just like this:
def sillycase(str):
return str[:round(len(str) / 2.0)+1].lower() + str[round(len(str) / 2.0)+1:].upper()
Hope this works :)
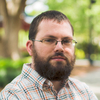
Kenneth Love
Treehouse Guest TeacherYou don't need to add anything to the round()
to make it correct.

Jason Anello
Courses Plus Student 94,610 PointsI might be over thinking this challenge but I think the accepted solution doesn't consistently place the middle character for odd length strings in the second half where it's uppercased. It does for a 9 character string but the middle character would be put in the first half for both a 7 and 11 character string.
python 3 will round 4.5 down to 4 but it rounds 5.5 up to 6. I think this rounding behavior changes which group the middle character will belong to.
I would think that we would either need additional logic to use the round()
function or perhaps use math.ceil()
instead if the goal was to consistently keep the middle character either in the first half or last half.
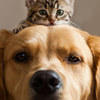
Devin Scheu
66,191 PointsYou don't need the +1's :)
def sillycase(c):
return c[:round(len(c) / 2.0)].lower() + c[round(len(c) / 2.0):].upper()
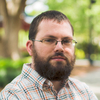
Kenneth Love
Treehouse Guest TeacherYou don't need the .0
s either (at least not in Python 3. Python 2 users would want them) :)
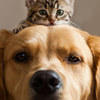
Devin Scheu
66,191 PointsYes Kenneth, and no .0s either :)

Damascan Ion
3,511 PointsTrye this for me it works
def sillycase(my_string): return my_string[:int(round(len(my_string))/2+0.5)].lower() + my_string[int(round(len(my_string) - round(len(my_string))/2+0.5)):].upper()
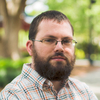
Kenneth Love
Treehouse Guest TeacherYour solution should work now for the CC if you want to try it again.
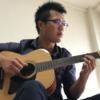
CHEN HAOMING
5,376 PointsBro. This quiz is too hard.....

Theodore Karropoulos
22,149 PointsYou can try this one
def sillycase(a_string):
return a_string[:round(len(a_string)/2)].lower() + a_string[round(len(a_string)/2):].upper()
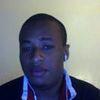
Abdoulaye Diallo
15,863 PointsI tried this one without adding 1 to the rounded length. def sillycase(str): return str[:round(len(str) / 2.0)].lower() + str[round(len(str) / 2.0):].upper()</p>

Bright Zhao
2,505 PointsI did below way, but seek for a better simple answer, because earlier I tried .lower() and .capitalize(), but that only work for one character instead of go through all of them.
def sillycase(str):
half = len(str) / 2
first_half = str[:4]
last_half = str[4:]
new_first = []
new_last = []
for l in first_half:
new_first.append(l.lower())
for u in last_half:
new_last.append(u.capitalize())
new_word = ''.join(new_first+new_last)
print(new_word)
return new_word

Jason Anello
Courses Plus Student 94,610 Pointsfixed code formatting
Hi Bright Zhao
See Annie Scott's answer which is currently at the top for a simpler solution.
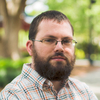
Kenneth Love
Treehouse Guest Teacherstr.capitalize
only capitalizes the first character of the string.

William Brown
1,303 Pointsdef sillycase(string):
math = int(len(string) / 2)
return string[:math+1].lower() + string[-math:].upper()
print(sillycase("treehouse"))

simon bao
5,522 Pointsdef sillycase(a):
String = a
half = round(len(String)/2)
return a[:half].lower() + a[half:].upper()

Lisa Burbon
1,030 Points"Entities must not be multiplied beyond necessity"(Occam's razor) String = a

Lisa Burbon
1,030 Pointsdef sillycase(string):
new_str = round(len(string) / 2)
return string[:new_str].lower() + string[new_str:].upper()

Ammar Fatihallah
7,417 Pointsthis is what i did i hope this help for the people who are looking around for a simple code
def sillycase(s):
strng = s
half = round(len(strng)/2)
return strng[:half].lower() + strng[half:].upper()
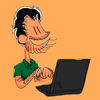
Juan Martin
14,335 PointsWhat should we do then? If I don't add 1, 4.5 will be rounded to 4 and the result will be "treeHOUSE" instead of "treehOUSE". Btw I changed str to a different variable (as str is the built-in type for strings), it has the same result :(
def sillycase(c):
return c[:round(len(c) / 2.0)+1].lower() + c[round(len(c) / 2.0)+1:].upper()
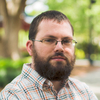
Kenneth Love
Treehouse Guest TeacherI have a feeling I need to fix the challenge description :)
Thanks for finding a bug!
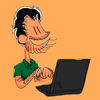
Juan Martin
14,335 PointsThank you for teaching us how awesome is python! Btw, please check the code I've submitted on the forums for the "Dungeon Game" :)
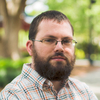
Kenneth Love
Treehouse Guest Teacher@Juan Martin: I'll definitely check out your code. I'm actually on vacation today (first day of school) but I'll give it a look-over tomorrow. Thanks for submitting something!
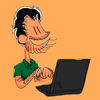
Juan Martin
14,335 PointsI had fun adding new things to the game! It's cool hehe! Thanks :)
Kenneth Love
Treehouse Guest TeacherKenneth Love
Treehouse Guest TeacherSince
str
is the built-in type for strings, you might want to pick a different variable name.