Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial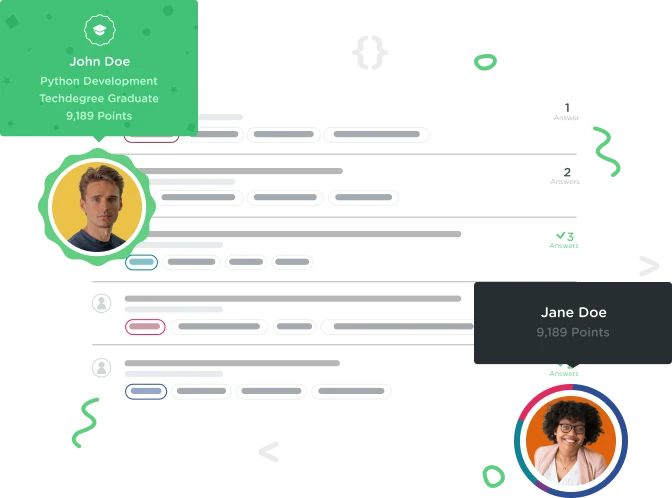

dh144011
1,231 PointsQuestion about formatting for Code Challenge: Word Count (Python)
I was having trouble getting this to complete, and i was hoping someone could shed some light on my error. The error was that it could not find all the words it needed to. I think it might just be because they wanted it formatted a certain way, but I am unsure.
Here is the task and the comments:
Create a function named word_count() that takes a string. Return a dictionary with each word in the string as the key and the number of times it appears as the value.
- E.g. word_count("I am that I am") gets back a dictionary like: {'i': 2, 'am': 2, 'that': 1}
- Lowercase the string to make it easier.
- Using .split() on the sentence will give you a list of words.
- In a for loop of that list, you'll have a word that you can
- Check for inclusion in the dict (with "if word in dict"-style syntax).
- Or add it to the dict with something like word_dict[word] = 1.
This is the way I tried it and it DIDN'T work
dictionary = {}
string = "in this city i am in my car driving in the city my car is gray like the sky in the city that i am driving in"
string = string.split(" ")
def word_count(string):
for word in string:
if word not in dictionary:
qty = string.count(word)
dictionary.update({word:qty})
return dictionary
dict_complete = word_count(string)
print(dict_complete)
This is the way I tried it and it did work
dictionary = {}
def word_count(string):
string = string.split(" ")
for word in string:
if word not in dictionary:
qty = string.count(word)
dictionary.update({word:qty})
return dictionary
dict_complete = word_count("in this city i am in my car driving in the city my car is gray like the sky in the city that i am driving in")
print(dict_complete)
Was there an issue I am missing on the first one, or is it simply a case of me not following instructions closely enough?
6 Answers
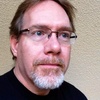
Chris Freeman
Treehouse Moderator 68,423 PointsWhen a challenge asks you to create a specific function it is solely this function that is reviewed by the checker.
In the failing example the sample string
is created and split()
outside the function. When the checker directly calls the function with a test string there is no split. The
for word in string
Becomes, effectively,
for letter in string
Since a string can be considered as a list of letters.
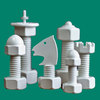
Steven Parker
229,786 PointsThe obvious difference is converting the string into a word array using split. The first example didn't do that, so the iteration would be for every character of the string instead of every word.
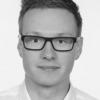
Urs Merkel
Courses Plus Student 4,552 PointsPlease could you advise on the difference between your working solution and my proper solution ;-) Mine is not working so far – I always get a "try again" =( thanks for your help in advance
def word_count(string):
dic = {}
array = string.lower()
string = array.split(" ")
for word in string:
if word not in dic:
qty = string.count(word)
dic.update({word:qty})
return dic
string = "Johanna ist ein sehr intelligentes Mädchen, sie ist hübsch und witzig. Johanna ist ein Mädchen."
dic_complete = word_count(string)
print(dic_complete)
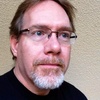
Chris Freeman
Treehouse Moderator 68,423 PointsUrs, if I remove the three lines of test code beneath the function definition it will pass. Those lines seem to interfere with the checker.
Also, as a coding style suggestion, it's best not to redefine the argument such as assigning to string
. This can affect functionality outside the function since Python is a "pass by object reference" language.
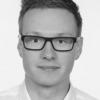
Urs Merkel
Courses Plus Student 4,552 PointsHi Chris, thank you for your help :-) now it is working.
Also, I will try to adopt your coding style suggestion ;-) thanks for that advise.

elizabeth gonzalez
2,532 PointsNot sure if this helps but my solution passed and would love feed back from the teachers/moderators to know if this is there is a better way to do it.
def word_count(x):
y = {}
x = x.lower()
x = x.split(" ")
for words in x:
if words not in y:
counts = x.count(words)
y.update({words : counts})
return y
[MOD: added ```python formatting -cf]
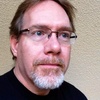
Chris Freeman
Treehouse Moderator 68,423 PointsHi Elizabeth,
Your code is cleanly structured and easy to read, but it is using list.count()
(as the other solutions in this thread). This is not as efficient as using a linear count method as used in the best answer for this solution.
Using list.count()
will parse the entire list to find the count for each word in the list which results in an O(n**2) performance. Also, it is repeating the count for words already seen.
In the linked solution above, the list is parsed exactly once which results in an O(n) performance.

elizabeth gonzalez
2,532 PointsHi Chris, I have 3 responses to that code... Hope you dont mind.
Are you referring to this
word_list = {}
count = 0
for word in words:
word_list.update(dict([(word, count)]))
print(word_list)
count += 1
If so, I have a few questions. Where you do the word_list.update(dict[(word,count)]) is the dict in front of the [] a function? also is it just saying put this list of word and count as a dict pair?
Also I dont see in that code how the words get split and lowercased?
You mention O(n**2) and O(n) I know this is to be algorithms specific but I havent learned any of the algorithms and would like to know when is treehouse going to do a algorighms course for python. Its something I would really like to do. Thank you