Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial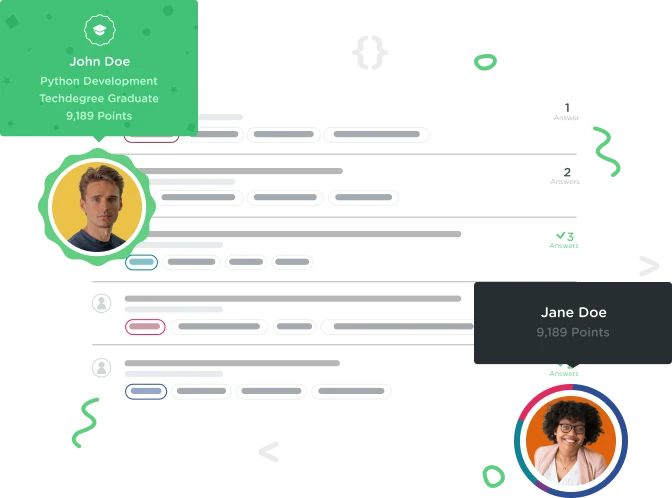

Mary Yang
1,769 PointsRegular Python Expressions - Challenge task 2 of 2. Please clarify the question for me.
Hi everyone, I have no idea what this question is asking me to search for. I'm a native English speaker, but the question just doesn't make sense to me. Can anyone please explain what it wants me to do using another phrasing? Here's the question:
Challenge Task 2 of 2
Now, write a function named numbers() that takes two arguments: a count as an integer and a string. Return a match for exactly count numbers in the string. Remember, you can multiply strings and integers to create your pattern.
For example: r"\w" * 5 would create r"\w\w\w\w\w".
import re
def first_number(strings):
data = re.search(r'\d', strings)
return data
def numbers(count, user_string):
# The heck am I supposed to be searching for?
outcome = re.search(r'', user_string)
8 Answers

james white
78,399 PointsI'm a native English speaker and this forum thread was just confusing..
Malcolm Jury was almost correct in the line of code he posted.
return re.search(r'\d' * number, string)
However, taking into account the original poster's def:
def numbers(count, user_string):
I think Malcolm's line of code would have to be adjusted (re-factored) to fit the rest of the code as follows:
import re
def first_number(strings):
data = re.search(r'\d', strings)
return data
def numbers(count, user_string):
return re.search(r'\d' * count, user_string)

Mary Yang
1,769 PointsHey Thanks for the explanation Kenneth Love. That was actually rather easy. The phrasing of the question just threw me off, as I wasn't able to figure out what I was expected to do. It probably didn't help that I was doing the lesson at midnight after a full day's work. :)
I'm not sure if you figured it out yet, but you basically just have to multiply the regular expression number operator by the number variable and search for it in the string. Something like this:
return re.search(r'\d' * number, string)
Where number and string are your input variables of course.
Cheers!
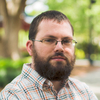
Kenneth Love
Treehouse Guest TeacherYou're going to get a count and a string. In that string, find where there are count
numbers in a row in the string. So if count
is 5, find 5 numbers in a row. If it's 3, find 3 numbers in a row.

SUDHARSAN CHAKRAVARTHI
Courses Plus Student 2,434 PointsYes . i did it . Again a Bummer !!!!....
def numbers(count, str): return re.match(r'\d' * count,str)
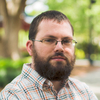
Kenneth Love
Treehouse Guest TeacherAh, it's because of the re.match
. Change to re.search
and it'll pass. I'll update the prompt so it's less confusing.

SUDHARSAN CHAKRAVARTHI
Courses Plus Student 2,434 PointsGot it . But i think little rephrase in question needed ...Thank you for your quick response.
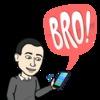
Malcolm Jury
11,194 PointsI understand that if the count was 5 then something like this would produce the result
return re.search(r'\d\d\d\d\d', aString)
Ive attempted to build up that r'/d/d/d/d/d' string using concatenation but am getting no where, it keeps on outputting none when i run the script
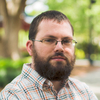
Kenneth Love
Treehouse Guest TeacherWell, /d/d/d/d/d
is not the same thing as \d\d\d\d\d
. The first one will be looking for exactly five /
with five d
s in between them. The second will match five numbers in a row.

SUDHARSAN CHAKRAVARTHI
Courses Plus Student 2,434 PointsHi can anyone tell me is this the right solution ?.
return re.search(r'\d' * number, string)
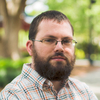
Kenneth Love
Treehouse Guest TeacherAssuming number
is the desired number of digits and string
is the string argument, yes, that should pass. Is it not? Can you show the rest of your code if this is failing.

SUDHARSAN CHAKRAVARTHI
Courses Plus Student 2,434 PointsTask :
write a function named numbers() that takes two arguments: a count as an integer and a string. Return a match for exactly count numbers in the string. Remember, you can multiply strings and integers to create your pattern.
The complete Code:
import re
def first_number(str): return re.search(r'\d', str)
def numbers(count, str): return re.match(r'\w' * count, str)
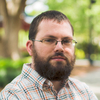
Kenneth Love
Treehouse Guest Teacher\w
catches word characters. We only want to catch digits so we should use \d
.