Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial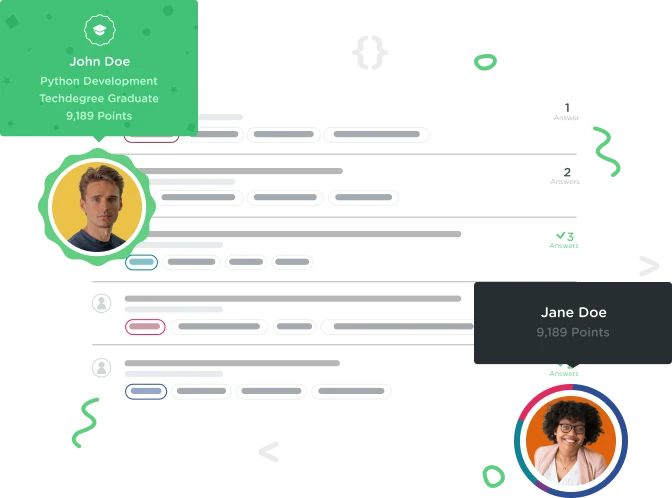

upul jayalath
1,294 Points'return' in the shopping_list.py
Why we need 'return' here? because we are not collecting the return value (shopping_list) in the main() specifically.
def add_to_list(shopping_list, new_item): # add new items to our list shopping_list.append(new_item) print("Added {}. List now has {} items.".format(new_item, len(shopping_list))) return shopping_list
def show_help():
# print out instructions on how to use the app
print("What should we pick up at the store?")
print("""
Enter 'DONE' to stop adding items.
Enter 'HELP' for this help.
Enter 'SHOW' to see your current list.
""")
def show_list(shopping_list):
# print out the list
print("Here's your list:")
for item in shopping_list:
print(item)
def add_to_list(shopping_list, new_item):
# add new items to our list
shopping_list.append(new_item)
print("Added {}. List now has {} items.".format(new_item, len(shopping_list)))
return shopping_list
show_help()
# make a list to hold onto our items
shopping_list = []
while True:
# ask for new items
new_item = input("> ")
# be able to quit the app
if new_item == 'DONE':
break
elif new_item == 'HELP':
show_help()
continue
elif new_item == 'SHOW':
show_list(shopping_list)
continue
add_to_list(shopping_list, new_item)
show_list(shopping_list)
4 Answers

markmneimneh
14,132 PointsHello
In Python, a method comes in two forms ... for the purpose of this discussion
form1
def method_name1(method_parameter1, method_parameter2):
do something
do more things
print things.
write to DB
read DB
and so on and so on
form 2
def method_name2(method_parameters):
do something
do more things
print things.
write to DB
read DB
and so on and so on
NOW RETURN SOMETHING BACK TO ME
if stuff_got_done ==True:
return true
else:
return False
inside the body of the program. I call form 1 like this
method_name1(1234, "take this input and do something")
now note. I called method_name1, gave it some input values, and I asked the method to use these input to do stuff. I am not expecting method_name1 to report back to me.
Again, inside the body of the program. 2 call form 1 like this
is_job_well_done = method_name1(1234, "take this input and do something")
so note here that method_name2 is reporting back to me "True or False" which for the purpose of this discussion, I am going to use it to infer that whatever I asked the function to get done ... it got/got not done.
now in the body of the program I can use is_job_well_done to do other stuff.
So ... in summary,
sometimes we call method and have them do things, but necessarily expecting a return value.
and sometimes we want them to return something back that we can use in other parts of the program.
You as a coder make that decision, and it is generally based on the design of the program you write.
Hope this help clear the concept. If this answers your question, please mark the question as answered.
thanks

upul jayalath
1,294 PointsThanks Mark. Your answer is clear. But still i have my concern in the given code. The 'return shoppling_list' statement is in the function named add_to_list(shopping_list, new_item), but we are not using this return value in the main function (body of the main function). so why this return statement is in the said function.
Is it because of we are updating the list in the add_to_list function and it should be updated in the main function even we dont use it in the main function.

markmneimneh
14,132 PointsHello
I believe this is the part that you are having issue with
def add_to_list(shopping_list,new_item):
shopping_list.append(new_item)
print("Added {}. List now has {} items.".format(new_item, len(shopping_list)))
return shopping_list
let take a look at this.
Please recall that a method is a part of the application tht specializes on doing things.
so you can write
a method to cut wood
a method to glue woods together to make a bird house
a method to paint the bird house
ad method to ship the birdhouse to market.
... and so on ... and so on.
now this is a method that specializes in adding an item to a list and return the list back to the caller,
def add_to_list(shopping_list,new_item):
shopping_list.append(new_item)
print("Added {}. List now has {} items.".format(new_item, len(shopping_list)))
return shopping_list
so instead of cluttering your code with too many things to do ... you create functions to handle specialized tasks.
This is such a function or method. its job is to get shopping list from the caller, and an item. Then push the item into the shopping list and then return the stuffed shopping list back to the caller.
analogy ... I can wrap all my Christmas presents ... but what I normally do is have the clerk (the method) at the store do it for me .... I hand him/her the a bag with items (passing in parameters to the method) and she/he returns the bag to me with the Christmas items wrapped.
so is the method analogy
def wrapped_gifts(blg_empty_bag, items_to_wrap):
get_wrapping_paper
wrap items_to_wrap (in the atgument list)
put a nice bow on the wrapped item
put the wrapped gift in the blg_empty_bag
return the blg_empty_bag to the customer (me)
``

upul jayalath
1,294 PointsThanks Mark. As mentioned I got your explanation completely. Much appreciated.
But my point is just simple thing..please understand..
This is taken from your explanation.
def add_to_list(shopping_list,new_item): shopping_list.append(new_item) print("Added {}. List now has {} items.".format(new_item, len(shopping_list))) return shopping_list
This job is to get shopping list, an item from the caller. Then push the items into the shopping list and then return the stuffed shopping list back to the caller.
But where is the caller. There is no caller in the main function? No one is calling this in body of the main function. So why we are returning this 'shopping_list' value to the caller.
See the main function below, it desnt show the caller.
shopping_list = [] while True: new_item = input("> ") if new_item == 'DONE': break elif new_item == 'HELP': show_help() continue elif new_item == 'SHOW': show_list(shopping_list) continue add_to_list(shopping_list, new_item) show_list(shopping_list)
My question is not in the theory. It is bound to this specific exercise.
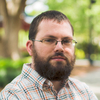
Kenneth Love
Treehouse Guest TeacherHmm, is the return
there in the course? I don't remember doing that but it's totally possible I did.

markmneimneh
14,132 PointsI found this s interesting way to check out the behavior (by ref and by val concepts)
Always good to try
def modify(l):
l.append('HI from Modify')
return l
def preserve(l):
t = list(l)
t.append('HI from preserve')
return t
example = list()
modify(example)
print(example)
example = list()
preserve(example)
print(example)
Alexander Davison
65,469 PointsAlexander Davison
65,469 PointsTagging Kenneth Love