Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial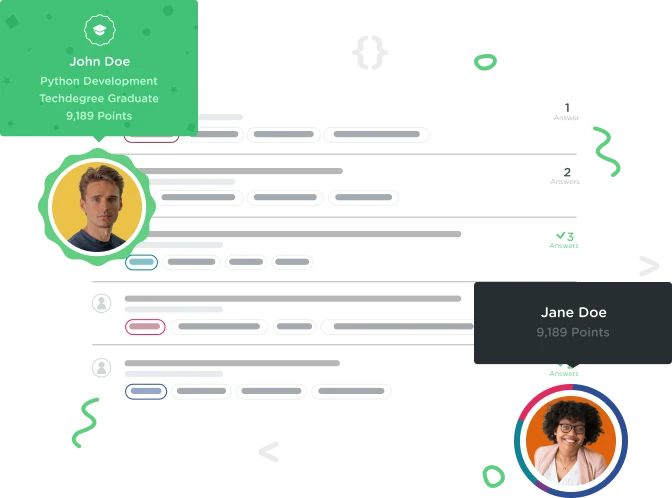

David Drysdale
10,815 PointsServer crashing after successfully displaying JSON data
I'm having a peculiar issue.
Everything works fine for the home route and for the error route, but when I write in a valid username, I get the correct data but the server then crashes with the following error:
Server running at port 3000.
events.js:141
throw er; // Unhandled 'error' event
^
Error: write after end
at ServerResponse.OutgoingMessage.write (_http_outgoing.js:413:15)
at Profile.<anonymous> (/home/treehouse/workspace/router.js:39:16)
at emitOne (events.js:77:13)
at Profile.emit (events.js:169:7)
at IncomingMessage.<anonymous> (/home/treehouse/workspace/profile.js:38:36)
at emitNone (events.js:72:20)
at IncomingMessage.emit (events.js:166:7)
at endReadableNT (_stream_readable.js:905:12)
at nextTickCallbackWith2Args (node.js:441:9)
at process._tickCallback (node.js:355:17)
Here is what's in my router.js file:
var Profile = require("./profile.js");
// Handle HTTPS route GET / and POST / i.e. Home
function home(request, response) {
// if url == "/" && GET
if(request.url === "/") {
// Show the search field
response.writeHead(200, {'Content-Type': 'text/plain'});
response.write("Header\n");
response.write("Search\n");
response.end('Footer\n');
}
// if url == "/" && POST
// redirect to /:username
}
// Handle HTTP route GET /:username i.e. /chalkers
function user(request, response) {
// if url == "/..."
var username = request.url.replace("/", "");
if(username.length > 0) {
response.writeHead(200, {'Content-Type': 'text/plain'});
response.write("Header\n");
// get json from Treehouse
var studentProfile = new Profile(username);
// on "end"
studentProfile.on("end", function(profileJSON) {
// show profile
// Store the values we need
var values = {
avatarUrl: profileJSON.gravatar_url,
username: profileJSON.profile_name,
badges: profileJSON.badges.length,
javascriptPoints:profileJSON.points.JavaScript
}
// Simple response
response.write(values.username + ' has ' + values.badges + ' badges.\n');
response.end('Footer\n');
});
// on "error"
studentProfile.on('error', function(error) {
// show error
response.write(error.message + '\n');
response.end('Footer\n');
});
}
}
module.exports.home = home;
module.exports.user = user;
Any ideas? I was wondering at first that I was having a problem because the data displays on end, and then I have the response.end line, but that's the way the error works, too, and I don't have a problem with that.
EDIT: Here's a snapshot of my workspace: https://w.trhou.se/srfspy68i9
4 Answers

Ryan Chatterton
5,914 PointsIf I remember when I did this, it has a lot of bugs mainly because its older. here is the project all finished (snap shot of mine). https://w.trhou.se/f3cwzn7q65
You will run into this as well https://teamtreehouse.com/community/cant-display-username-receiving-error
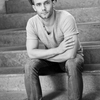
Rune Rapley-Møller
Courses Plus Student 24,411 PointsHi Ryan, could you repost your workspace snapshot again (it's not found 404).?
I keep getting the following error: error connect econnrefused ?
any advice towards the right direction is much apreciated.
Thx

David Drysdale
10,815 PointsThanks, Ryan Chatterton—good to know it isn't just me. It is a bit frustrating that the lesson includes such buggy code, though. I spent a lot of time trying to debug it, while also trying to figure out how to apply it to a project of my own. I hope they update this course soon.
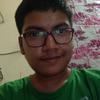
Abirbhav Goswami
15,450 Points// get json from Treehouse
var studentProfile = new Profile(username);
// on "end"
studentProfile.on("end", function(profileJSON) { // <-- Problem lies on this line
// show profile
Since the profile is shown on request end, you cannot write anything after the first successful call.

David Drysdale
10,815 PointsThanks, Ryan Chatterton . I'm still confused, though, because I don't think my code's any different from Andrew Chalkley's code at this point, and his seems to work without getting that error. Is there some way to fix this, or is it a fault in the lesson? (And if so, will it be fixed, I wonder.)

Tyler Taylor
17,939 PointsRyan's answer skipped a few lessons ahead. So I just kept going until I got to that part, now my server doesn't crash. My code was exactly the same as Andrew's, and I was experiencing the same issue as you. I'm a little annoyed with the quality of these lessons. It's as if they had to record it in one shot, with no editing.
Ryan Chatterton
5,914 PointsRyan Chatterton
5,914 PointsHi David,
in your code:
is executed synchronously, but each call does asynchronous calls to external ressources before calling response.write. So response.end is getting called to early.