Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial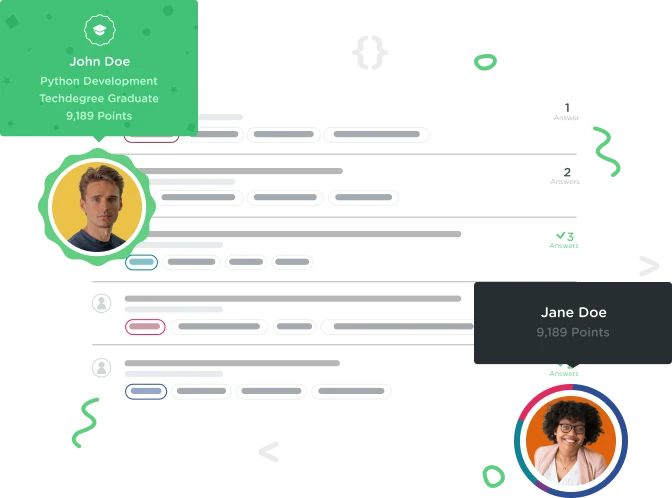

Patrick Munemo
14,892 PointsService binding and unbinding
Now use that ServiceConnection to bind and unbind GeolocationService at the appropriate points in the Activity lifecycle. Also, add a new boolean field named 'isBound', and use it to make sure that we only call 'unbindService' if we're actually bound to the Service.
public class MainActivity extends Activity {
private boolean isBound = true;
private ServiceConnection serviceConnection = new ServiceConnection() {
@Override
public void onServiceConnected(ComponentName name, IBinder service) {
isBound = true;
}
@Override
public void onServiceDisconnected(ComponentName name) {
isBound = false;
}
};
int latitude;
int longitude;
@Override
public void onCreate(Bundle savedInstanceState) {
setContentView(R.layout.activity_main);
Button updateLocationButton = (Button) findViewById(R.id.update_button);
updateLocationButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v){
}
});
@Override
protected void onStart() {
super.onStart();
Intent intent = new Intent(this, GeolocationService.class);
bindService(intent, serviceConnection, Context.BIND_AUTO_CREATE);
isBound = true;
}
@Override
protected void onStop() {
super.onStop();
if(isBound){
unbindService(serviceConnection);
isBound = false;
}
}
}
}
public class GeolocationService extends Service {
@Override
public IBinder onBind(Intent intent) {
return null;
}
//Client methods
public int getLatitude() { return getLat(); }
public int getLongitude() { return getLng(); }
}
1 Answer

Ben Deitch
Treehouse TeacherHey Patrick!
In MainActivity you've got your brackets out of order. It looks like the closing bracket for onCreate is actually below onStop. Also, you want onStart and onStop to be 'public' instead of 'protected'.
Hope that helps!
Patrick Munemo
14,892 PointsPatrick Munemo
14,892 PointsHey Ben Deitch , can you please tell me whats wrong with my code. Its giving me a bunch of errors