Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial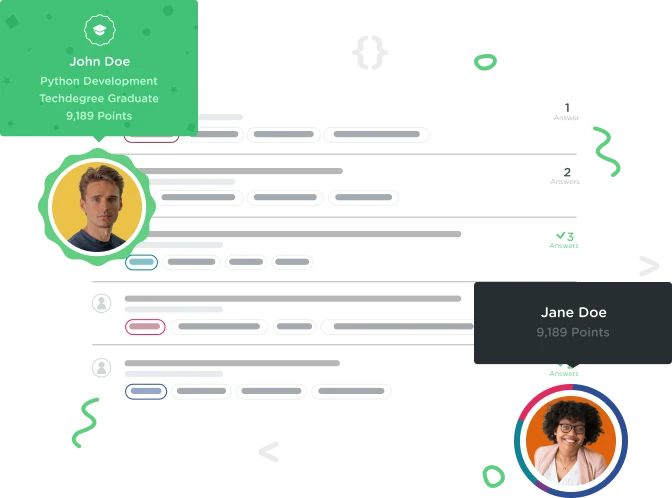

Malcolm Mutambanengwe
4,205 Pointssetting date and time
What am I doing wrong?
import java.util.Date;
public class Movie {
private String mTitle;
private Date mReleaseDate;
private String mFormattedReleaseDate;
public String getTitle() {
return mTitle;
}
public void setTitle(String title) {
mTitle = title;
}
public Date getReleaseDate() {
return mReleaseDate;
}
public void setReleaseDate(Date date) {
mReleaseDate = date;
}
public void getFormattedReleaseDate() {
return mFormattedReleaseDate;
}
public void setFormattedReleaseDate(String FormattedReleaseDate) {
mFormattedReleaseDate = formattedReleaseDate;
}
}
2 Answers
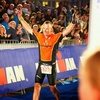
Steve Hunter
57,712 PointsHi,
You want to call the format
method on the new formatter
instance and pass in mReleaseDate
as the parameter. Use dot notation for the method call; formatter.format();
and put the date that is already declared in the brackets. That will return a formatted string to the call; return that straight out of your method:
public String getFormattedReleaseDate(){
SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd");
return formatter.format(mReleaseDate);
}
Make sense?
Steve.
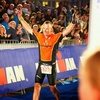
Steve Hunter
57,712 PointsHi Mal,
For the first part of this just return an empty string from your new method:
public String getFormattedReleaseDate(){
return "";
}
Is that where you're at?
Steve.

Malcolm Mutambanengwe
4,205 PointsHi Steve
Yep, this is where I am. Thanks for the speedy reply.

Malcolm Mutambanengwe
4,205 PointsThe mReleaseDate member variable is already stored as a Date object. Convert it and return it using the SimpleDateFormat variable.
import java.util.Date;
public class Movie {
private String mTitle;
private Date mReleaseDate;
public String getTitle() {
return mTitle;
}
public void setTitle(String title) {
mTitle = title;
}
public Date getReleaseDate() {
return mReleaseDate;
}
public void setReleaseDate(Date date) {
mReleaseDate = date;
}
public String getFormattedReleaseDate(ReleaseDate) {
SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd");
return formatter;
}
}
I was thinking that I would take the output from ReleaseDate and use it as input to FormattedReleaseDate and then the output returned.
[MOD: edited code block - srh]
Malcolm Mutambanengwe
4,205 PointsMalcolm Mutambanengwe
4,205 PointsOh okay, I get it. Slowly I'm getting there.
Thanks Steve.
Steve Hunter
57,712 PointsSteve Hunter
57,712 Points