Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial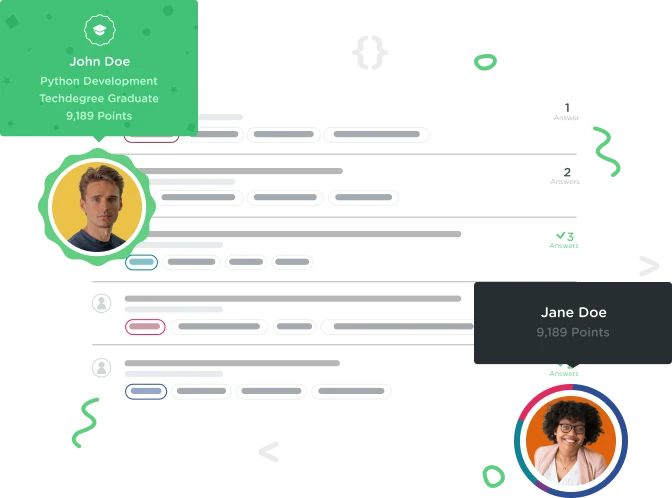

Andrew Weston
490 PointsShopping List not showing
I'm running this script. It compiles perfectly but show_list() doesn't seem to return a list.
shopping_list = []
def show_help():
print("\nSeperate each item with a comma.")
print("Type DONE to quit, SHOW to see the current list, and HELP to get this message.")
def show_list():
count = 1
for item in shopping_list:
print("{} : {}".format(count, item))
count += 1
print("Give me a list of things you want to shop for.")
show_help()
while True:
new_stuff = input("> ")
if new_stuff == "DONE":
print("\nHere's your list:")
show_list()
break
elif new_stuff == "HELP":
show_help()
continue
elif new_stuff == "SHOW":
show_list()
continue
else:
new_list = new_stuff.split(",")
index = input("Add this at a certain spot? Press enter for the end of the list,"
"or give me a number. Currently {} items in the list.".format(len(shopping_list)))
if index:
spot = int(index) - 1
for item in new_list:
shopping_list.insert(spot, item.strip())
spot += 1
else:
for item in new_list:
shopping_list.append(item.strip())
3 Answers
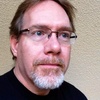
Chris Freeman
Treehouse Moderator 68,457 PointsYou are correct. show_list()
doesn't return anything explicitly since there is no return
statement. It appears to simply print the contents of the shopping_list
What action were you looking for?
Here's what it looks like to me:
>>> Give me a list of things you want to shop for.
Seperate each item with a comma.
Type DONE to quit, SHOW to see the current list, and HELP to get this message.
> apple, banana, cherry
Add this at a certain spot? Press enter for the end of the list,or give me a number. Currently 0 items in the list.
> SHOW
1 : apple
2 : banana
3 : cherry
> DONE
Here's your list:
1 : apple
2 : banana
3 : cherry
>>>
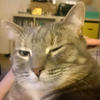
Rui W
6,269 PointsI think you're asking the same question as: https://teamtreehouse.com/community/what-is-wrong-please-help
Please refer to Aaron Kaye's answer in that question using above link. In short words, currently your last "else" is indented with the "for" 3 lines above it. To fix this you'll need to back-tab/un-indent the last "else" block of code.
if index:
spot = int(index) - 1
for item in new_list:
shopping_list.insert(spot, item.strip())
spot += 1
else: #<------back-tabbed this else and all below lines
for item in new_list:
shopping_list.append(item.strip())
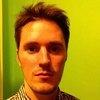
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsFirst of all, I want to make sure you're clear on the difference between a function printing something out and returning a value. This function isn't supposed to return a list, it's suppose to print out all the items in the list. But if it's not printing anything, I suspect the reason is that the list was empty.
When you run the app, it prompts you: "Give me a list of things you want to shop for.". You're supposed to enter in a list of items separated by commas, and it makes a list out of them. Then if you type SHOW it will show you the list items, but if you haven't made a list it will be empty.
Michel van Essen
8,077 PointsMichel van Essen
8,077 PointsNot sure if this is what you're looking for but if you want your program to show list you need to add show_list() somewhere at the end.